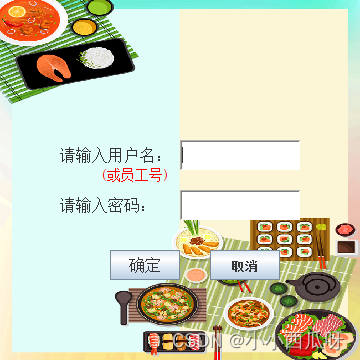
手把手带你完成数据库课程设计(餐饮管理系统)
手把手带你完成数据库课程设计
·
期末快到了,不知道各位小伙伴有没有在为自己的课程设计还没完成而发愁,不用担心,这篇文章将手把手带你完成一个餐饮管理系统。
1.顾客点餐部分
1.1界面部分
1.1收集顾客信息
这里收集到了顾客的电话和就餐人数信息后,会将对应信息收集到对应的数据表中
/**
* 起始页面,收取顾客联系电话和就餐人数信息并点击点餐后跳转CustomerOrderWindow页面
*/
public class CustomerIndex extends JFrame implements ActionListener {
Image image;
JPanel jp,jp_phone,jp_person;
JTextField jp_phone_jtf,jtf_person;
JLabel jp_phone_jl,jl_person;
JButton jb;
String phone;//传给点餐的那个页面
String number;
public static void main(String[] args) {
CustomerIndex index = new CustomerIndex();
}
public CustomerIndex() {
jp_phone_jl=new JLabel("请输入联系电话");
jp_phone_jl.setFont(MyTools.f3);
jp_phone_jtf=new JTextField(20);
jb = new JButton("点餐");
jb.addActionListener(this);
jb.setFont(MyTools.f3);
jp_phone=new JPanel();
jp_phone.add(jp_phone_jl);
jp_phone.add(jp_phone_jtf);
jp_phone.add(jb);
jp_person=new JPanel();
jl_person=new JLabel("请输入就餐人数");
jl_person.setFont(MyTools.f3);
jtf_person=new JTextField(20);
jp_person.add(jl_person);
jp_person.add(jtf_person);
jp = new JPanel(new BorderLayout());
image = Toolkit.getDefaultToolkit().getImage("image/二维码.png");//背景图片
ImagePanel ip = new ImagePanel(image);
jp.add(jp_phone,"South");
jp.add(ip, "Center");
jp.add(jp_person,"North");
this.add(jp);
this.setSize(500, 500);
/**
* 确定JWindow的初始位置
*/
this.setLocationRelativeTo(null);
this.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource()==jb){
phone = jp_phone_jtf.getText();
number= jtf_person.getText();
Connection connection=null;
PreparedStatement preparedStatement=null;
ResultSet resultSet=null;
try {
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
//获取相关的值
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
String url = properties.getProperty("url");
//1. 注册驱动
Class.forName(driver);//建议写上
//2. 得到连接
connection = DriverManager.getConnection(url, user, password);
//3. 得到Statement
String sql="insert into 顾客(联系电话) values (?)";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1,phone);
preparedStatement.executeUpdate();
this.dispose();
} catch (IOException ex) {
ex.printStackTrace();
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} catch (SQLException ex) {
ex.printStackTrace();
} finally {
try {
if(resultSet!=null){
resultSet.close();
}
if(preparedStatement!=null) {
preparedStatement.close();
}
if(connection!=null) {
connection.close();
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
new CustomerOrderWindow(phone,number);
}
}
}
1.2顾客选择餐桌
/**
* 起始页面,收取顾客联系电话和就餐人数信息并点击点餐后跳转CustomerOrderWindow页面
*/
public class CustomerIndex extends JFrame implements ActionListener {
Image image;
JPanel jp,jp_phone,jp_person;
JTextField jp_phone_jtf,jtf_person;
JLabel jp_phone_jl,jl_person;
JButton jb;
String phone;//传给点餐的那个页面
String number;
public static void main(String[] args) {
CustomerIndex index = new CustomerIndex();
}
public CustomerIndex() {
jp_phone_jl=new JLabel("请输入联系电话");
jp_phone_jl.setFont(MyTools.f3);
jp_phone_jtf=new JTextField(20);
jb = new JButton("点餐");
jb.addActionListener(this);
jb.setFont(MyTools.f3);
jp_phone=new JPanel();
jp_phone.add(jp_phone_jl);
jp_phone.add(jp_phone_jtf);
jp_phone.add(jb);
jp_person=new JPanel();
jl_person=new JLabel("请输入就餐人数");
jl_person.setFont(MyTools.f3);
jtf_person=new JTextField(20);
jp_person.add(jl_person);
jp_person.add(jtf_person);
jp = new JPanel(new BorderLayout());
image = Toolkit.getDefaultToolkit().getImage("image/二维码.png");//背景图片
ImagePanel ip = new ImagePanel(image);
jp.add(jp_phone,"South");
jp.add(ip, "Center");
jp.add(jp_person,"North");
this.add(jp);
this.setSize(500, 500);
/**
* 确定JWindow的初始位置
*/
this.setLocationRelativeTo(null);
this.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource()==jb){
phone = jp_phone_jtf.getText();
number= jtf_person.getText();
Connection connection=null;
PreparedStatement preparedStatement=null;
ResultSet resultSet=null;
try {
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
//获取相关的值
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
String url = properties.getProperty("url");
//1. 注册驱动
Class.forName(driver);//建议写上
//2. 得到连接
connection = DriverManager.getConnection(url, user, password);
//3. 得到Statement
String sql="insert into 顾客(联系电话) values (?)";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1,phone);
preparedStatement.executeUpdate();
this.dispose();
} catch (IOException ex) {
ex.printStackTrace();
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} catch (SQLException ex) {
ex.printStackTrace();
} finally {
try {
if(resultSet!=null){
resultSet.close();
}
if(preparedStatement!=null) {
preparedStatement.close();
}
if(connection!=null) {
connection.close();
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
new CustomerOrderWindow(phone,number);
}
}
}
1.3顾客开始点菜+加入餐车+支付
/**
* 菜单表格,点击加入菜单按钮后弹出CanChe页面
*/
public class Menu extends JFrame implements ActionListener{
//定义需要的组件
/**
* p1是最上面的查找
* p2是中间的表格
* p3是最下面的加入餐车,点餐完毕
*/
JPanel p1,p2,p3,p4,p5;
JLabel p1_lab1,p3_lab1;
JTextField p1_jtf1;
JButton p1_jb1,p1_jb1_refresh,p3_jb_add,p3_jb_over;
//用于显示人事信息的表格
JTable jtable;
//滚动面板
JScrollPane jsp;
Model customermodel;
MenuModel mm;//做类的成员变量
// public static void main(String[] args) {
CustomerOrderWindow w=new CustomerOrderWindow("123456");
new Menu(w,"123456");
// }
/**
* 点餐界面
* @param COwindow 餐桌界面
* @param phone 顾客的电话
*/
public Menu(CustomerOrderWindow COwindow,int desk,String phone) {
/**
* 通过电话查询到顾客编号
*/
Menu MenuWindow=this;
customermodel=new Model();
String [] params={phone};
customermodel.query("select * from 顾客 where 联系电话=?",params);
//此时customermodel模型里面只有联系方式为phone的这一行
//下面通过getValueAt获取顾客编号即可
//创建需要的组件
p1=new JPanel(new FlowLayout(FlowLayout.CENTER));
p1_lab1=new JLabel("请输入想查询的菜名");
p1_lab1.setFont(MyTools.f3);
p1_jtf1=new JTextField(20);
/**
* 查询
*/
p1_jb1=new JButton("查询");
p1_jb1.setFont(MyTools.f3);
p1_jb1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("用户希望查询:");
//因为把对表的数据封装到StuModel中,我们就可以比较简单的完成查询
String name = p1_jtf1.getText().trim();//把文本框中的内容赋给name
String id = p1_jtf1.getText().trim();//把文本框中的内容赋给id
//写一个sql语句
// String sql = "select * from 人事资料 where stuName='" + name + "' and 1 =? ";
mm=new MenuModel();
String [] params={"1"};
//关闭详细信息界面后显示的信息,要与点击之前的保持一致
mm.init("select 菜名,价格 from 菜单 where 菜名='"+name+"' and 1=?",params);//精准查询
// mm.init("select * from 人事资料 where 姓名 LIKE'"+name+"%' and 1=?",params);//模糊查询
// mm.init("select * from 人事资料 where 员工号='"+id+"' and 1=?",params);//精准查询
jtable.setModel(mm);
jsp=new JScrollPane(jtable);
p2.add(jsp);
}
});
p1_jb1_refresh=new JButton("刷新");
p1_jb1_refresh.setFont(MyTools.f3);
p1_jb1_refresh.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("用户点击了刷新");
mm=new MenuModel();
String [] params={"1"};
//关闭详细信息界面后显示的信息,要与点击之前的保持一致
mm.query("select 菜名,价格 from 菜单 where 1=?",params);
jtable.setModel(mm);
jsp=new JScrollPane(jtable);
p2.add(jsp);
}
});
//加入到p1
p1.add(p1_lab1);
p1.add(p1_jtf1);
p1.add(p1_jb1);
p1.add(p1_jb1_refresh);
// p1.add(p1_jb1_refresh);
/**
* 处理中间的
*/
mm=new MenuModel();
String [] param={"1"};
mm.query("select 菜名,价格 from 菜单 where 1=?",param);
jtable=new JTable(mm);
jtable.setSize(500,500);
p2=new JPanel();
/**
* 将jtable放入滚动面板才能看到列名
* p2.add(jtable);//这样不行
*/
jsp=new JScrollPane(jtable);
p2.add(jsp);
/**
* 加入餐车
*/
p3_jb_add=new JButton("加入餐车");
p3_jb_add.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int selectedRow = jtable.getSelectedRow();//返回选择的行数
if(selectedRow==-1){
JOptionPane.showMessageDialog(COwindow,"请点餐","提示",JOptionPane.INFORMATION_MESSAGE);
return ;
}
String c_id=(String)customermodel.getValueAt(0,0);
// System.out.println(c_id+"号顾客");
String [] params={"1"};
mm.query("select 菜名,价格 from 菜单 where 1 = ?",params);
new MenuAddCanChe(mm,selectedRow,Integer.parseInt(c_id));
// new CanChe(Integer.parseInt(c_id));
new CanChe(MenuWindow,COwindow,Integer.parseInt(c_id),desk,"满桌");
System.out.println(" 加入餐车");
}
});
p3_jb_add.setFont(MyTools.f3);
p3=new JPanel();
p3.add(p3_jb_add);
// p3.add(p3_jb_over);
this.setTitle("请点餐");
this.add(p1,"North");
this.add(p2,"Center");
this.add(p3,"South");
int width = Toolkit.getDefaultToolkit().getScreenSize().width;
int height = Toolkit.getDefaultToolkit().getScreenSize().height;
this.setLocation(width/2-800,height/2-300);
this.setSize(800,600);
this.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
}
}
class MenuAddCanChe{
Model customermodel;
String caiming;
String price;
public MenuAddCanChe(MenuModel mm,int selectedRow,int c_id) {
customermodel=new Model();
String [] params={"1"};
customermodel.query("select * from 顾客 where 1=?",params);
caiming=(String)mm.getValueAt(selectedRow,0);
System.out.print(caiming+" ");
price=(String)mm.getValueAt(selectedRow,1);//这里的price是String类型,后面记得转换
System.out.print(price);
Connection connection=null;
PreparedStatement preparedStatement=null;
ResultSet resultSet=null;
try {
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
//获取相关的值
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
String url = properties.getProperty("url");
//1. 注册驱动
Class.forName(driver);//建议写上
//2. 得到连接
connection = DriverManager.getConnection(url, user, password);
//3. 得到Statement
String sql="insert into 餐车(菜名,价格,顾客编号) values(?,?,?)";
// String sql="update 人事资料 set 姓名=?,性别=?,年龄=?,身份证号=?,联系电话=?,职位=? where 员工号=?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1,caiming);
preparedStatement.setInt(2,Integer.parseInt(price));
preparedStatement.setInt(3,c_id);
// preparedStatement.setInt(3,id);
preparedStatement.executeUpdate();
} catch (IOException ex) {
ex.printStackTrace();
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} catch (SQLException ex) {
ex.printStackTrace();
} finally {
try {
if(resultSet!=null){
resultSet.close();
}
if(preparedStatement!=null) {
preparedStatement.close();
}
if(connection!=null) {
connection.close();
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
}
}
/**
* 餐车页面,点击点餐完毕后跳出Consume页面
*/
public class CanChe extends JFrame implements ActionListener {
JButton jb_select,jb_pay,jb_reflesh;
JPanel p1,p2,p3;
JTable jtable;
JScrollPane jsp;
MenuModel mm;
int c_id;
int desk;
String state;
// public static void main(String[] args) {
new CanChe(40,5,"满桌");
// }
public CanChe(Menu MenuWindow,CustomerOrderWindow COwindow,int c_id,int desk,String state){
CanChe CanCheWindow=this;
this.c_id = c_id;
this.desk = desk;
this.state = state;
/**
* 表格
*/
mm=new MenuModel();
int [] params={c_id};
mm.query("select 菜名,价格,菜品数量 from 餐车 where 顾客编号=?",params);
jtable=new JTable(mm);
jsp=new JScrollPane(jtable);
p1=new JPanel();
p1.add(jsp);
jb_pay=new JButton("点餐完毕");
jb_pay.setFont(MyTools.f3);
jb_pay.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
modifyState();
new Consume(CanCheWindow,MenuWindow,COwindow,c_id,desk,"空闲");
}
});
p2=new JPanel();
// p2.add(jb_select);
p2.add(jb_pay);
this.setTitle("您已选择如下菜品");
this.setLayout(new BorderLayout());
this.add(p1,"Center");
this.add(p2,"South");
// this.add(p3,"North");
int width = Toolkit.getDefaultToolkit().getScreenSize().width;
int height = Toolkit.getDefaultToolkit().getScreenSize().height;
this.setLocation(width/2,height/2-300);
this.setSize(800,600);
this.setVisible(true);
}
public void modifyState(){
System.out.println("用户提交了菜单");
Connection connection=null;
PreparedStatement preparedStatement=null;
ResultSet resultSet=null;
try {
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
//获取相关的值
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
String url = properties.getProperty("url");
//1. 注册驱动
Class.forName(driver);//建议写上
//2. 得到连接
connection = DriverManager.getConnection(url, user, password);
//3. 得到Statement
String sql="update 餐桌 set 使用状态=? where 餐桌号=?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1,state);
preparedStatement.setInt(2,desk);
preparedStatement.executeUpdate();
} catch (IOException ex) {
ex.printStackTrace();
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} catch (SQLException ex) {
ex.printStackTrace();
} finally {
try {
if(resultSet!=null){
resultSet.close();
}
if(preparedStatement!=null) {
preparedStatement.close();
}
if(connection!=null) {
connection.close();
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
}
@Override
public void actionPerformed(ActionEvent e) {
}
}
public class Consume extends JFrame implements ActionListener {
JLabel jl;
JButton jb_ensure;
JPanel p1,p2;
int desk;
String state;
String total_price;
int c_id;
int num;
// public static void main(String[] args) {
// new Consume(15,2,"满桌");
// }
public Consume(CanChe CanCheWindow,Menu MenuWindow,CustomerOrderWindow COWindow,int c_id,int desk,String state) {
this.num=0;
this.c_id = c_id;
this.desk=desk;
this.state=state;
p1=new JPanel();
p2=new JPanel();
MenuModel mm=new MenuModel();
int [] params={c_id};
mm.query("select sum(价格) from 餐车 where 顾客编号=?",params);
total_price=(String)mm.getValueAt(0,0);
System.out.println("该顾客共消费了"+total_price+"元");
jl=new JLabel("你共消费了"+total_price+"元");
jl.setFont(MyTools.f3);
jb_ensure=new JButton("支付");
jb_ensure.setFont(MyTools.f3);
jb_ensure.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("用户已完成支付");
modifyState();
addDingdan();
CanCheWindow.dispose();
MenuWindow.dispose();
COWindow.dispose();
}
});
p1.add(jl);
p2.add(jb_ensure);
this.setLayout(new BorderLayout());
this.add(p1,"Center");
this.add(p2,"South");
this.setTitle("这是你的消费账单");
int width = Toolkit.getDefaultToolkit().getScreenSize().width;
int height = Toolkit.getDefaultToolkit().getScreenSize().height;
this.setLocation(width/2-200,0);
this.setSize(400, 200);
this.setVisible(true);
}
/**
* 添加数据进订单表
*/
public void addDingdan(){
Connection connection=null;
PreparedStatement preparedStatement=null;
ResultSet resultSet=null;
try {
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
//获取相关的值
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
String url = properties.getProperty("url");
//1. 注册驱动
Class.forName(driver);//建议写上
//2. 得到连接
connection = DriverManager.getConnection(url, user, password);
//3. 得到Statement
String sql="insert into 订单(消费金额,顾客编号) values (?,?)";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1,total_price);
preparedStatement.setInt(2,c_id);
preparedStatement.executeUpdate();
this.dispose();
} catch (IOException ex) {
ex.printStackTrace();
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} catch (SQLException ex) {
ex.printStackTrace();
} finally {
try {
if(resultSet!=null){
resultSet.close();
}
if(preparedStatement!=null) {
preparedStatement.close();
}
if(connection!=null) {
connection.close();
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
}
/**
* 修改餐桌状态为空闲并将就餐人数设置回0
*/
public void modifyState(){
Connection connection=null;
PreparedStatement preparedStatement=null;
ResultSet resultSet=null;
try {
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
//获取相关的值
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
String url = properties.getProperty("url");
//1. 注册驱动
Class.forName(driver);//建议写上
//2. 得到连接
connection = DriverManager.getConnection(url, user, password);
//3. 得到Statement
String sql="update 餐桌 set 使用状态=?,就餐人数=? where 餐桌号=?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1,state);
preparedStatement.setInt(2,num);
preparedStatement.setInt(3,desk);
preparedStatement.executeUpdate();
} catch (IOException ex) {
ex.printStackTrace();
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} catch (SQLException ex) {
ex.printStackTrace();
} finally {
try {
if(resultSet!=null){
resultSet.close();
}
if(preparedStatement!=null) {
preparedStatement.close();
}
if(connection!=null) {
connection.close();
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
}
@Override
public void actionPerformed(ActionEvent e) {
}
}
1.2数据库部分
2.后台管理部分
2.1界面部分
2.1.1用户登录(员工账号和经理账号因为权限不同会有不同界面,下面只展示经理界面)
package com.restaurant.view;
import com.restaurant.model.UserModel;
import com.restaurant.tools.MyTools;
import javax.imageio.ImageIO;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
/**
* 用户登录界面
*/
public class UserLogin extends JDialog implements ActionListener {
JLabel jl1,jl2,jl3;
JTextField jName;
JPasswordField jPassword;
JButton jConf,jCancel;
public static void main(String[] args) {
UserLogin userLogin = new UserLogin();
}
public UserLogin() {
//把一个组件放入JFrame或者JDialog可以直接放,也可以先放到Container
Container ct=this.getContentPane();
//空布局
this.setLayout(null);
//创建各组件
jl1=new JLabel("请输入用户名:");
// jl1.setBounds(60,190,150,30);
jl1.setBounds(60,140,150,30);
jl1.setFont(MyTools.f1);
ct.add(jl1);
jName=new JTextField(20);
jName.setFocusable(true);
// jName.setBounds(180,190,120,30);
jName.setBounds(180,140,120,30);
jName.setBorder(BorderFactory.createLoweredBevelBorder());//下凹
ct.add(jName);
jl2=new JLabel("(或员工号)");
jl2.setForeground(Color.red);//前景色
jl2.setFont(MyTools.f2);
// jl2.setBounds(100,210,100,30);
jl2.setBounds(100,160,100,30);
ct.add(jl2);
jl3=new JLabel("请输入密码:");
// jl3.setBounds(60,240,150,30);
jl3.setBounds(60,190,150,30);
jl3.setFont(MyTools.f1);
ct.add(jl3);
jPassword=new JPasswordField(20);
// jPassword.setBounds(180,240,120,30);
jPassword.setBounds(180,190,120,30);
jPassword.setBorder(BorderFactory.createLoweredBevelBorder());
ct.add(jPassword);
jConf=new JButton("确定");
jConf.setFont(MyTools.f1);
// jConf.setBounds(110,300,70,30);
jConf.setBounds(110,250,70,30);
ct.add(jConf);
jConf.addActionListener(this);
jCancel=new JButton("取消");
// jCancel.setBounds(210,300,70,30);
jCancel.setBounds(210,250,70,30);
ct.add(jCancel);
jCancel.addActionListener(this);
//创建一个BackImage对象
BackImage bi = new BackImage();
//把位置确定
bi.setBounds(0,0,360,360);
//不使用上下框
ct.add(bi);
//this.add(bi);
this.setUndecorated(true);
this.setSize(360,360);
this.setLocationRelativeTo(null);
this.setVisible(true);
}
/**
* 响应用户登录请求
* @param e
*/
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource()==jConf){
//取出员工号,密码
String u=this.jName.getText().trim();
String p=new String(this.jPassword.getPassword());
UserModel um=new UserModel();
String res=um.checkUser(u,p);
//调试
System.out.println(res);
if(res.equals("系统管理员")||res.equals("经理")){
new Window1();
this.dispose();
}else if(res.equals("员工")){
new Windows2();
this.dispose();
}else{
JOptionPane.showMessageDialog(this,"你无权登录或用户名密码错误","登陆提示",JOptionPane.ERROR_MESSAGE);
return ;
}
}else if(e.getSource()==jCancel){
this.dispose();
System.exit(0);
}
}
private class BackImage extends JPanel{
Image im;
public BackImage(){
try {
im= ImageIO.read(new File("image/login.jpg"));
} catch (Exception e) {
e.printStackTrace();
}
}
public void paintComponent(Graphics g){
g.drawImage(im,0,0,360,360,this);
}
}
}
2.1.2后台管理主界面
后续代码就不做公布了,太长了
如果大家只需要应付课程设计的话,前面的顾客点餐部分就可以当作一个完整的点餐系统来完成课程设计要求了,并且已经有了完整的数据库设计的逻辑,足够大家自主学习了,源码现在已经开源到了gitee社区,链接地址如下:餐饮管理系统: 数据库课程设计——一个较为完整的餐饮管理系统 (gitee.com)
大家可以去这里下载源码,希望各位小伙伴觉得不错的话可以给我一个star。
希望对大家有所帮助。
更多推荐
所有评论(0)