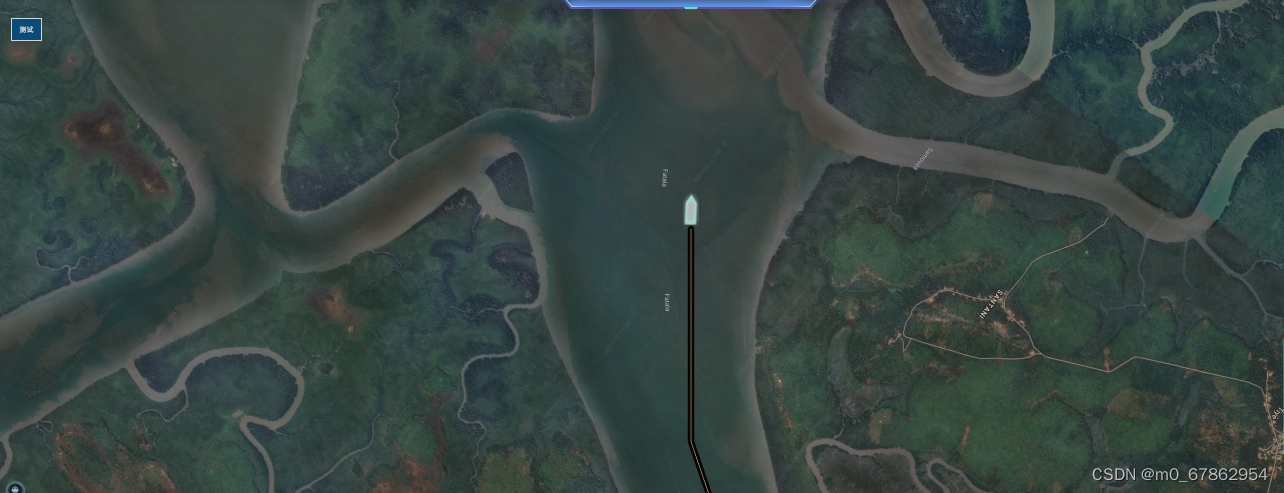
vue、angular结合高德地图实现镜头追踪+轨迹运动效果、切换视图、分段动画、无缝衔接 封装
【代码】angular结合高德地图实现镜头追踪+轨迹运动效果、切换视图、分段动画、无缝衔接 封装。
·
<div style="background-color: blue;padding: 10px 15px;cursor: pointer;z-index: 9;color:#fff;border: 1px solid #eee;" class="start-btn" (click)="stepOneStartFn()">测试</div>
declare var AMap: any;
declare var AMapUI: any;
declare var Loca: any;
AMap;
Loca;
stepOneFinished = false;
stepOnePolyline = null;
stepTwoPathSimplifierIns = null;
ngAfterViewInit() {
this.initMapFn()
}
initMapFn(){
let that = this
that.AMap = new AMap.Map('map', {
terrain: true,
viewMode: '3D',
zoom: 13.5,
center: [102.620455, 30.973672],
pitch: 45,
rotation: 0,
showLabel: true,
mapStyle: 'amap://styles/509934ebf66e54cbfe10ccae0056c462',
showBuildingBlock: false,
dragEnable: false,
zoomEnable: false,
});
}
stepOneStartFn(){
let that = this,
lineArr1 = [[102.620455,30.973672],[102.620983,30.973509],[102.621572,30.973171],[102.62226,30.972732],....],
lineArr2 = [[102.829925,30.993177],[102.831209,30.993469],[102.832315,30.99383],[102.834562,30.994623],....]
if(that.lineData02.coords){
// 初始化重置
if(that.stepOnePolyline){
that.Loca = null;
that.AMap.remove(that.stepOnePolyline)
}
if(that.stepTwoPathSimplifierIns){
that.stepTwoPathSimplifierIns.clearPathNavigators();//销毁现存的所有轨迹巡航器
that.AMap.remove(that.stepTwoPathSimplifierIns)
}
// 放大地图
for(let i = 1;i<107;i+=3){
that.AMap.setZoomAndCenter(Number((3.8+i/10).toFixed(1)), lineArr1 [0], false, 120*(i/10))
}
setTimeout(()=>{
that.AMap.setRotation(142, true, 0)
that.stepOneStartFn(lineArr1,lineArr2)
},3500)
}
}
// =====镜头追踪+轨迹运动=====
/**
* 参数说明
* @param stepOneLinePath --镜头追踪线段数据[[],[],...]
* @param stepTwoLinePath --轨迹运动线段数据[[],[],...]
*/
//第一段
stepOneStartFn(stepOneLinePath,stepTwoLinePath){
let that = this;
let stepOnePath = [];
that.stepOneFinished = false;
stepOneLinePath.forEach(item=>{
let cood = []
item.forEach(element => {
cood.push(Number(element))
});
stepOnePath.push(cood)
})
that.Loca = new Loca.Container({
map: that.AMap,
});
// 轨迹
var marker = new AMap.Marker({
position: stepOnePath[0],
icon: "https://a.amap.com/jsapi_demos/static/demo-center-v2/car.png",
anchor: 'bottom-center',
map: that.AMap,
})
that.stepOnePolyline = new AMap.Polyline({
path: [stepOnePath[0], stepOnePath[0]],
isOutline: true,
outlineColor: '#000000', // 描边
borderWeight: 6,
strokeColor: "#ffeeff",
strokeOpacity: 1,
strokeWeight: 1,
strokeStyle: 'solid',
lineJoin: 'round',
lineCap: 'round',
zIndex: 500,
map: that.AMap,
});
function run() {
if (!that.stepOneFinished) {
var center = that.AMap.getCenter().toArray();
var lastPath = that.stepOnePolyline.getPath();
lastPath.push(center);
if (lastPath.length === 1) {
lastPath.push(center);
}
that.stepOnePolyline.setPath(lastPath);
marker.setPosition(center);
}
requestAnimationFrame(run);
}
run();
that.Loca.animate.start();
that.stepOnePolyline.setPath([stepOnePath[0], stepOnePath[0]]);
that.Loca.viewControl.addTrackAnimate({
path: stepOnePath, // 镜头轨迹,二维数组,支持海拔
duration: 15000, // 完成时间,毫秒
timing: [[0, 0.3], [1, 0.7]], // 动画速度控制器,使用贝塞尔曲线控制
rotationSpeed: 360, // 在拐弯处每秒旋转多少度
}, function () {// 动画完成的回调函数
that.stepOneFinished = true;
console.log('完成');
setTimeout(()=>{
that.AMap.remove(that.stepOneMaker)
that.Loca.viewControl.clearAnimates();
that.AMap.setRotation(0, true, 0)
for(let i = 1;i<105;i+=3){
that.AMap.setZoomAndCenter(Number((14.4-i/10).toFixed(1)), that.lineData02.coords[0], false, 200*(i/10))
}
that.AMap.setZoomAndCenter(3.8, [51.358225, 10.180080], false, 2100)
},800)
setTimeout(()=>{
that.stepTwoStartFn(lineArr2)
},4000)
}
);
}
//第二段
stepTwoStartFn(pathVal){
let that = this
that.AMap.setZoomAndCenter(4, [51.358225, 10.180080], true)
AMapUI.load(['ui/misc/PathSimplifier', 'lib/$'], function(PathSimplifier, $) {
if (!PathSimplifier.supportCanvas) {
alert('当前环境不支持 Canvas!');
return;
}
var emptyLineStyle = {
lineWidth: 0,
fillStyle: null,
strokeStyle: null,
borderStyle: null
};
that.stepTwoPathSimplifierIns = new PathSimplifier({
zIndex: 100,
autoSetFitView:false,//是否在绘制后自动调整地图视野以适合全部轨迹,默认true。
map: that.AMap, //所属的地图实例
getPath: function(pathData, pathIndex) {
return pathData.path;
},
getHoverTitle: function(pathData, pathIndex, pointIndex) {
return null;
},
renderOptions: {
//将点、线相关的style全部置emptyLineStyle
pathLineStyle: emptyLineStyle,
pathLineSelectedStyle: emptyLineStyle,
pathLineHoverStyle: emptyLineStyle,
keyPointStyle: emptyLineStyle,
startPointStyle: emptyLineStyle,
endPointStyle: emptyLineStyle,
keyPointHoverStyle: emptyLineStyle,
keyPointOnSelectedPathLineStyle: emptyLineStyle
}
});
that.stepTwoPathSimplifierIns.setData([{
name: '测试',
path: pathVal
}]);
function onload() {
that.stepTwoPathSimplifierIns.renderLater();
}
function onerror(e) {
console.log('图片加载失败!');
}
//创建一个轨迹巡航器。createPathNavigator(pathIndex:number, options:Object) pathIndex:关联的轨迹索引
var navg1 = that.stepTwoPathSimplifierIns.createPathNavigator(0, {
loop: false,//循环播放
speed: 5000000,//速度
pathNavigatorStyle: {
width: 53,
height: 83,
content: PathSimplifier.Render.Canvas.getImageContent(/MapLegend2-2.png',onload, onerror),
strokeStyle: null,
fillStyle: null,
//经过路径的样式
pathLinePassedStyle: {
lineWidth: 6,
strokeStyle: 'black',
dirArrowStyle: {
stepSpace: 15,
strokeStyle: 'red'
}
}
}
});
navg1.start();
});
}
更多推荐
所有评论(0)