使用python构建数据库_使用Python构建(半)自主无人机
使用python构建数据库They might not be delivering our mail (or our burritos) yet, but drones are now simple, small, and affordable enough that they can be considered a toy. You can even customize and program
使用python构建数据库
They might not be delivering our mail (or our burritos) yet, but drones are now simple, small, and affordable enough that they can be considered a toy. You can even customize and program some of them via handy dandy Application Programming Interfaces (APIs)! The Parrot AR Drone has an API that lets you control not only the drone’s movement but also stream video and images from its camera. In this post, I’ll show you how you can use Python and node.js to build a drone that moves all by itself.
他们可能还没有交付我们的邮件( 或我们的墨西哥卷饼 ),但是无人机现在非常简单,小巧且价格适中,因此可以视为玩具。 您甚至可以通过方便的花哨的应用程序编程接口( API )对其中的一些进行自定义和编程! 派诺特AR无人机具有API,该API不仅使您可以控制无人机的运动,还可以从其摄像头中传输视频和图像。 在本文中,我将向您展示如何使用Python和node.js构建可自行移动的无人机。
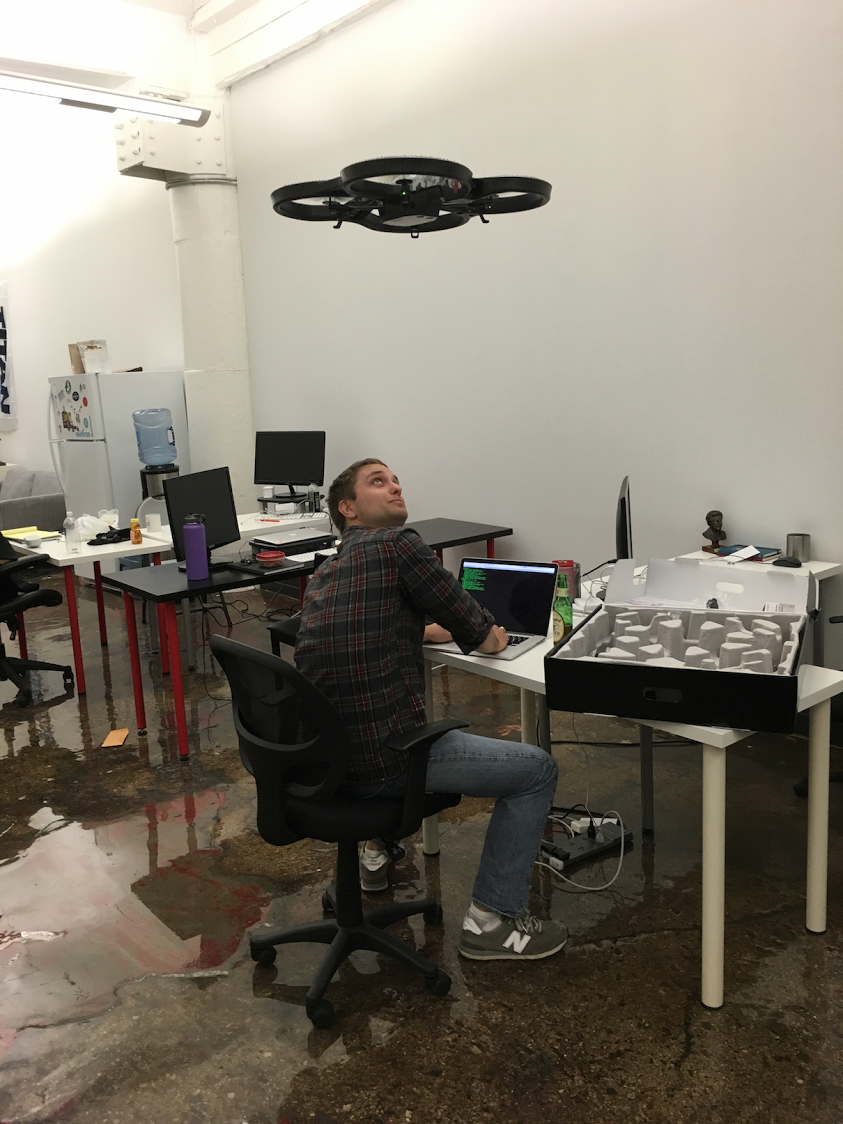
Hold onto your butts.
抓住你的屁股。
该项目 (The Project)
So given that I’m not a drone, or a machine vision professional, I’m going to have to keep things simple. For this project, I’m going to teach my drone how to follow a red object.
因此,鉴于我不是无人驾驶飞机或机器视觉专业人士,我将必须保持简单。 对于这个项目,我将教无人机如何跟随红色物体 。
I know, I know, it’s a far cry from a T-800 Model 101 (or even something like this), but given my time and budget constraints it’s a good place to start! In the meantime, feel free to send your best autonomous terminators or drone swarms my way.
我知道,这与T-800 101型( 甚至是类似的东西 )相去甚远,但是鉴于我的时间和预算限制,这是一个不错的起点! 同时,请随时发送您最好的自动终结器或无人驾驶的无人机群。
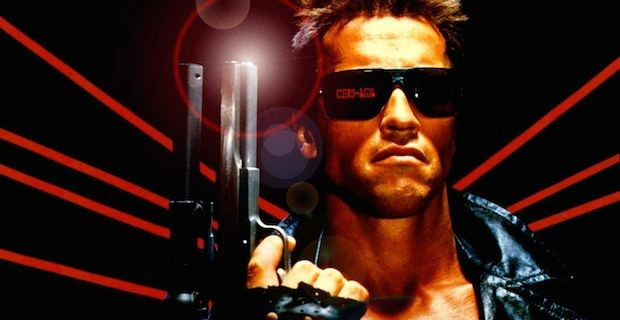
No neural net processors here, just node.js and Python.
这里没有神经网络处理器,只有node.js和Python。
无人机 (The Drone)
When I opened my drone on Christmas morning I wasn’t entirely sure what I was going to do with it, but one thing was for certain: This thing was cool. The AR Drone 2.0 (I know super lame name) is a quadcopter. If you’re imagining those fit in the palm of your hand, single-rotor, RC gizmos, you’re in the wrong ballpark. The first thing I noticed (and was most surprised by) was how big the AR Drone is. With its “indoor shell” on, it’s about 2 feet wide, 2 feet long, and 6 inches high. It’s also kind of loud–in a good way (like a terrify your dog kind of way, unlike this down to drone pup). Combine that with 2 cameras–one front and one bottom, and you’ve got yourself the ultimate grown up geek toy.
在圣诞节早晨打开无人机时,我不确定自己要怎么做,但是可以肯定的是,有一件事情很酷。 AR Drone 2.0 (我知道超级la脚的名字)是一个四轴飞行器。 如果您正在想象那些适合您的手掌,单转子,RC小物件,那您就错了。 我注意到的第一件事(最让他感到惊讶的是)AR Drone有多大。 启用“室内外壳”后,它大约2英尺宽,2英尺长,6英寸高。 它也很响亮-很好(就像吓坏狗一样,不像无人机那样)。 将其与2个摄像头(一个前置和一个底部)结合使用,您便拥有了最终的成人怪胎玩具。
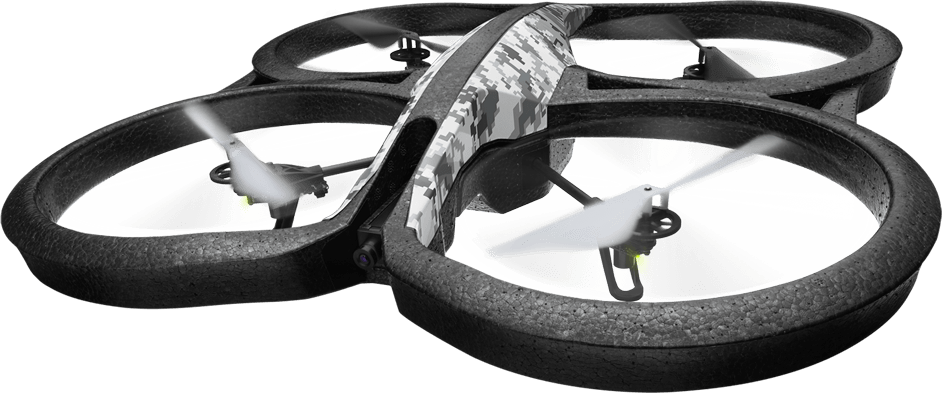
对无人机进行编程 (Programming your drone)
What sets the AR Drone apart is that it’s old (in drone years)–it was first released in 2012. This might seem like a bad thing BUT since we’re trying to program this gizmo, it’s actually a good thing.
AR Drone的与众不同之处在于它很古老(在无人机年代中)–它于2012年首次发布。但这似乎是一件坏事,因为我们正在尝试对此Gizmo进行编程,这实际上是一件好事。
Given that it’s had 4 years to “mature”, there are some really great APIs, helper libraries, and project/code samples for controlling/programming the drone (see list of resources below). So in essence, someone else has already done the hard part of figuring out how to communicate with the drone in bytecode, so all I have to do is import the node_module and I’m off to the figurative drone races.
鉴于它已经“成熟”了4年,所以有一些非常出色的API,帮助程序库以及用于控制/编程无人机的项目/代码示例(请参见下面的资源列表)。 因此,从本质上讲,其他人已经完成了如何与字节码进行无人机通信的艰苦工作,因此,我要做的就是导入node_module,然后开始进行具有象征意义的无人机竞赛 。
Programming the drone is actually quite easy. I’m using the ar-drone
node.js module. I’ve found that it works really well despite not being under super active development. To start, let me show you how to do a pre-programmed flightplan. The following program is going to:
对无人机进行编程实际上非常容易。 我正在使用ar-drone
node.js模块。 我发现尽管没有进行积极的开发,它仍然可以很好地工作。 首先,让我向您展示如何进行预先设定的飞行计划。 以下程序将去:
- connect to the drone over wifi
- tell the drone to takeoff
- after 1 second, spin clockwise at full throttle
- 1 second after that, stop and then move forwards at 50% thrust
- another 1 second after that, stop and land
- 通过wifi连接到无人机
- 告诉无人机起飞
- 1秒后,以全油门顺时针旋转
- 此后1秒钟,停下来,然后以50%的推力向前移动
- 再过一秒钟,停下来降落
Pretty simple little program. Now even though it’s pretty straightforward, I will still highly recommend having an emergency landing script readily available. Cause you never know you need one till you really need one 😉
非常简单的小程序。 现在,即使非常简单,我仍然强烈建议您随时准备一个紧急着陆脚本 。 因为你永远不知道自己需要一个直到真正需要一个
var arDrone = require('ar-drone'); var drone = arDrone.createClient(); drone.takeoff(); drone .after(1000, function() { drone.clockwise(1.0); }) .after(1000, function() { drone.stop(); drone.front(0.5); }) .after(1000, function() { drone.stop(); drone.land(); })
var arDrone = require('ar-drone'); var drone = arDrone.createClient(); drone.takeoff(); drone .after(1000, function() { drone.clockwise(1.0); }) .after(1000, function() { drone.stop(); drone.front(0.5); }) .after(1000, function() { drone.stop(); drone.land(); })
[embedded content]
[嵌入内容]
You can also pull off some fancier moves–you know, to impress your friends. My personal favorite is a backflip.
您也可以采取一些更奇妙的举动,以打动您的朋友。 我个人最喜欢的是后空翻。
[embedded content]
[嵌入内容]
机器视觉 (Le Machine Vision)
Ok now for the second piece of the puzzle: teaching our drone how to see. To do this, we’re going to be using OpenCV and the Python module cv2. OpenCV can be a little prickly to work with, but it can do some really impressive stuff and even has some machine learning libraries baked right into it.
现在好了,拼图的第二部分:教我们的无人机如何看。 为此,我们将使用OpenCV和Python模块cv2 。 OpenCV的使用可能有点棘手,但它可以做一些非常令人印象深刻的工作,甚至还包含一些机器学习库。
We’re going to be using OpenCV to do some basic object tracking. We’re going to have the camera track anything red that appears in its field of vision. Sort of like a bull at a bullfight.
我们将使用OpenCV进行一些基本的对象跟踪。 我们将使相机跟踪其视野中出现的任何红色 。 有点像斗牛。
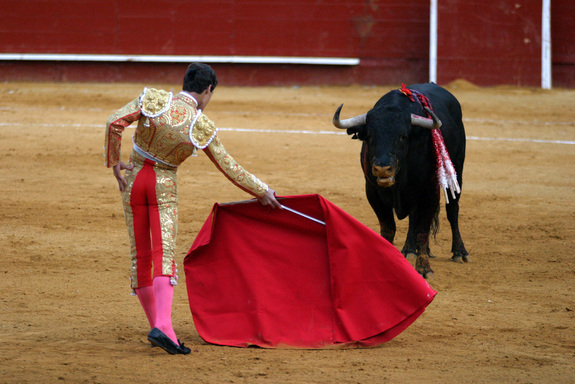
Just like this, except substitute the bull for a drone, and the red cape (muleta) for a Greg ☹! Also, my pants aren’t quite that tight.
就像这样,除了用公牛代替无人驾驶飞机,用红色斗篷(穆莱塔)代替格雷格☹! 另外,我的裤子不太紧。
Good news for us is that cv2
makes this really easy to do.
对我们来说好消息是cv2
使得此操作非常容易。
As you can see above, I’m using a color mask to filter the pixels in an image. It’s a simple but intuitive approach. And more importantly it works. Take a look:
正如您在上面看到的那样,我正在使用颜色遮罩来过滤图像中的像素。 这是一种简单但直观的方法。 而且更重要的是它有效 。 看一看:
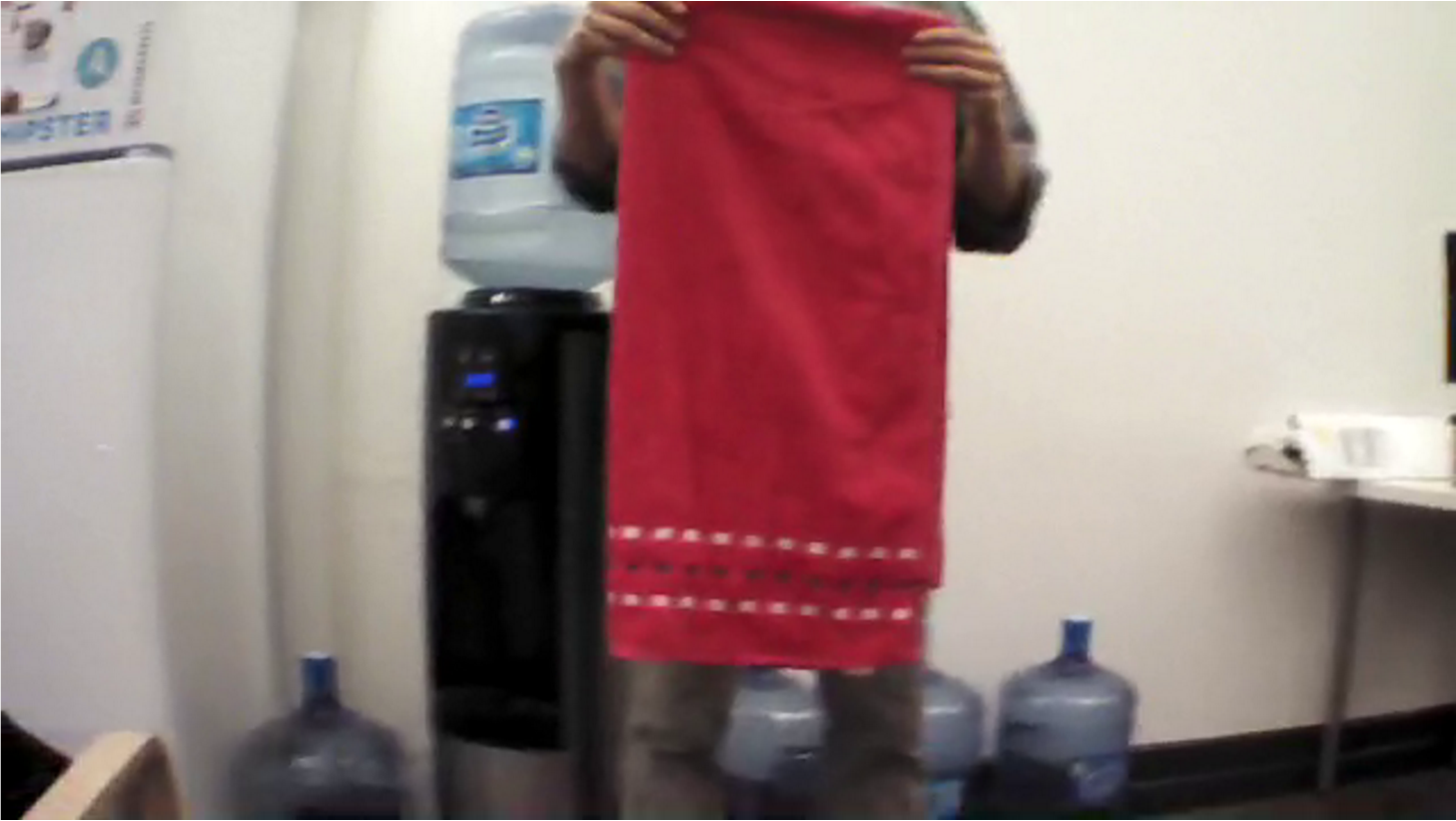
Raw camera feed
原始相机供稿
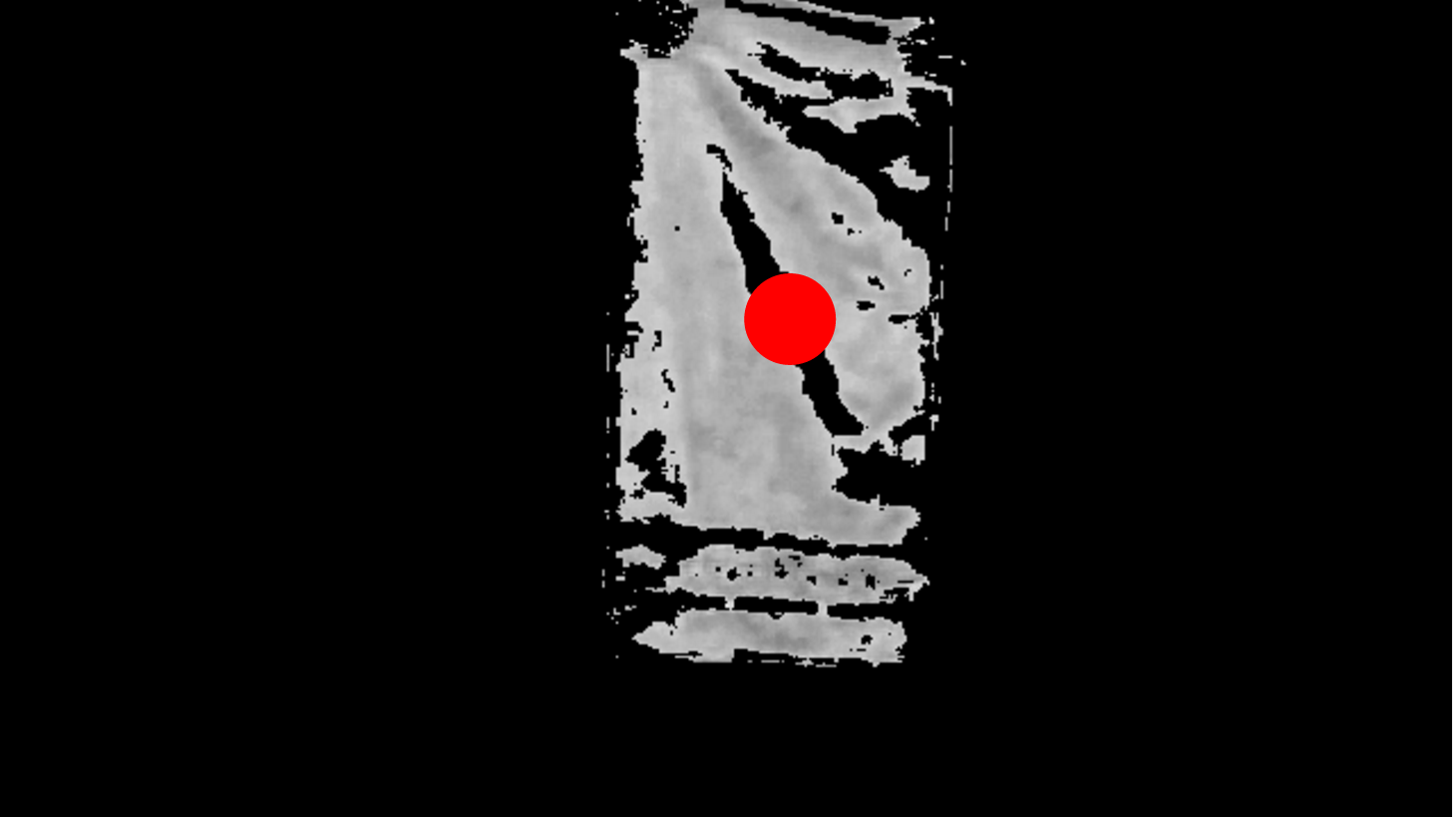
Processed with red filter
用红色滤光片处理
It’s learning! Ok well maybe not quite like a T-800 Model 101, but it’s at least a start.
在学习! 好吧,也许不太像T-800 101型,但这至少是一个开始。
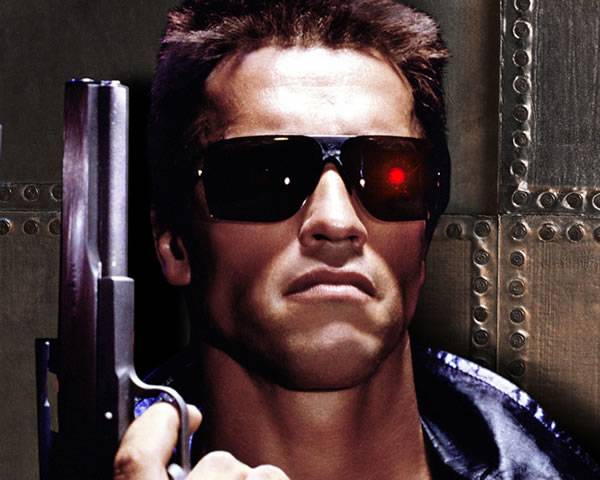
Is the red dot a coincidence? Think again…
红点是巧合吗? 再想一想…
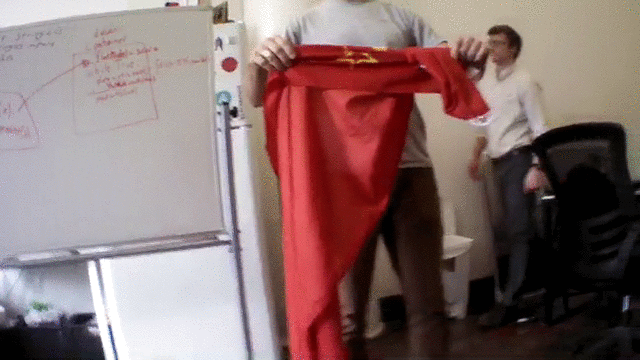
Raw camera feed
原始相机供稿
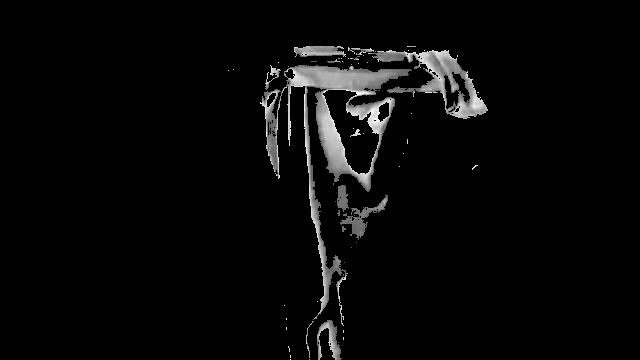
Processed with red filter
用红色滤光片处理
缝合在一起 (Stitching things together)
Ok here comes the tricky part. We’ve got our little node.js script that can control the drone’s navigation, and we’ve got the python bit that can detect where red things are in an image, but the question looms: How do we glue them together?
好了,这是棘手的部分。 我们有可以控制无人机导航的小node.js脚本,还有可以检测图像中红色物体在哪里的python位,但是问题迫在眉睫: 我们如何将它们粘合在一起?
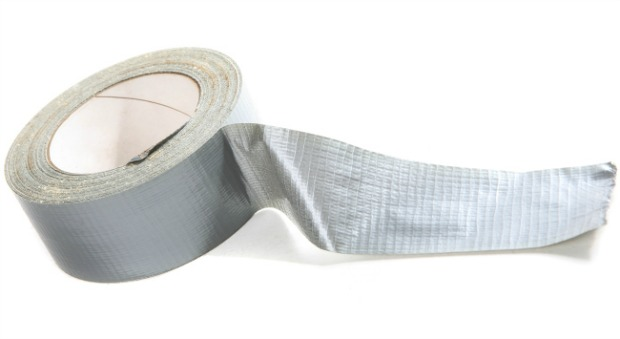
Well my friends, to do this I’m going to use Yhat’s own model deployment software, ScienceOps. I’m going to deploy my Python code onto ScienceOps, where it’ll be accessible via an API, and then from node.js I can call my model on ScienceOps. What this means is that I’ve boiled my OpenCV red-filtering model into a really simple HTTP endpoint. I’m using ScienceOps to make my childhood drone bull fighting dreams come true, but you could use it to embed any R or Python model into any application capable of making API requests, be it drone or otherwise.
我的朋友们,要做到这一点,我将使用Yhat自己的模型部署软件ScienceOps 。 我将把我的Python代码部署到ScienceOps上,在这里可以通过API进行访问,然后从node.js可以在ScienceOps上调用我的模型。 这意味着我已经将OpenCV红色过滤模型构建为一个非常简单的HTTP端点。 我正在使用ScienceOps来实现我的童年无人驾驶斗牛梦想,但您可以使用它将任何R或Python模型嵌入到能够发出API请求的任何应用程序中,无论它是无人机还是其他。
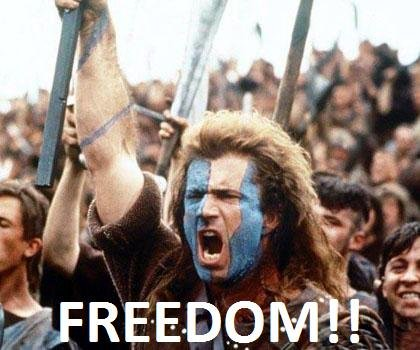
No more recoding to get models into production. FREEDOM!
无需进行重新编码即可将模型投入生产。 自由!
I don’t need to mess around with any cross-platform baloney, and if I need to up the horsepower of my model (say for instance if I’m controlling more than one drone), I can let ScienceOps scale out my model automatically. If you want more info about deploying models (or drones) into production using ScienceOps, head over to our site or schedule a demo to see it live.
我不需要弄乱任何跨平台的baloney,并且如果我需要提高模型的功能(例如,如果我控制多于一架无人机),我可以让ScienceOps自动扩展模型。 如果您想了解有关使用ScienceOps将模型(或无人机)部署到生产中的更多信息,请转到我们的网站或安排演示以现场观看。
from yhat import Yhat, YhatModel class DroneModel(YhatModel): REQUIREMENTS = [ "opencv" ] def execute(self, data): return get_coords(data['image64']) yh = Yhat(USERNAME, APIKEY, "https://sandbox.yhathq.com/") yh.deploy("DroneModel", DroneModel, globals(), True)
from yhat import Yhat, YhatModel class DroneModel(YhatModel): REQUIREMENTS = [ "opencv" ] def execute(self, data): return get_coords(data['image64']) yh = Yhat(USERNAME, APIKEY, "https://sandbox.yhathq.com/") yh.deploy("DroneModel", DroneModel, globals(), True)
What does all this mean? Well for one, it means my node.js code just got a lot simpler. I can even use the Yhat node.js library to execute my model:
这是什么意思呢? 好了,这意味着我的node.js代码变得简单了很多。 我什至可以使用Yhat node.js库执行我的模型:
Sweet! Now I can pretty much just drop this into my navigation script. All I need to do is tell my script how I want to react to the response. In this case it’s going to be a couple steps:
甜! 现在,我几乎可以将其放到导航脚本中。 我需要做的就是告诉脚本我要如何对响应做出React。 在这种情况下,将需要几个步骤:
- Call the
DroneModel
model hosted on ScienceOps - If there weren’t any errors, look at the result. The result will give me the
x
andy
coordinates of the red in the image. - Make course adjustments to the drone that attempt to move the red to the center of the drone’s field of vision.
- 调用ScienceOps上托管的
DroneModel
模型 - 如果没有任何错误,请查看结果。 结果将为我提供图像中红色的
x
和y
坐标。 - 对无人机进行路线调整,以尝试将红色移动到无人机视野的中心。
So simple! What could possibly go wrong?
很简单! 可能出什么问题了?
[embedded content]
[嵌入内容]
修补我的隐喻针脚 (Mending my metaphorical stitching)
As the adage goes, If at first you don’t succeed try, try again. It took me a few iterations to get the autonomous piece to actually work. Turns out, combining individual components has the propensity to compound your error!
正如谚语所说,如果一开始您没有成功,请再试一次。 我花了几次迭代才能使自主作品真正发挥作用。 事实证明,组合单个组件有可能加剧您的错误!
But not to worry! My drone took its fair share of bumps and bruises but it’s a tough little guy–Pro Tip: You can patch up your drone with duct tape. Just be sure to apply equal amounts to each side of the drone so it’s balanced!
但是不用担心! 我的无人驾驶飞机碰到了很多颠簸和瘀伤,但这是一个坚强的小家伙– 专家提示:您可以用胶带将无人驾驶飞机打补丁。 只要确保将等量的东西均匀地涂在无人机的每一侧,就可以保持平衡!
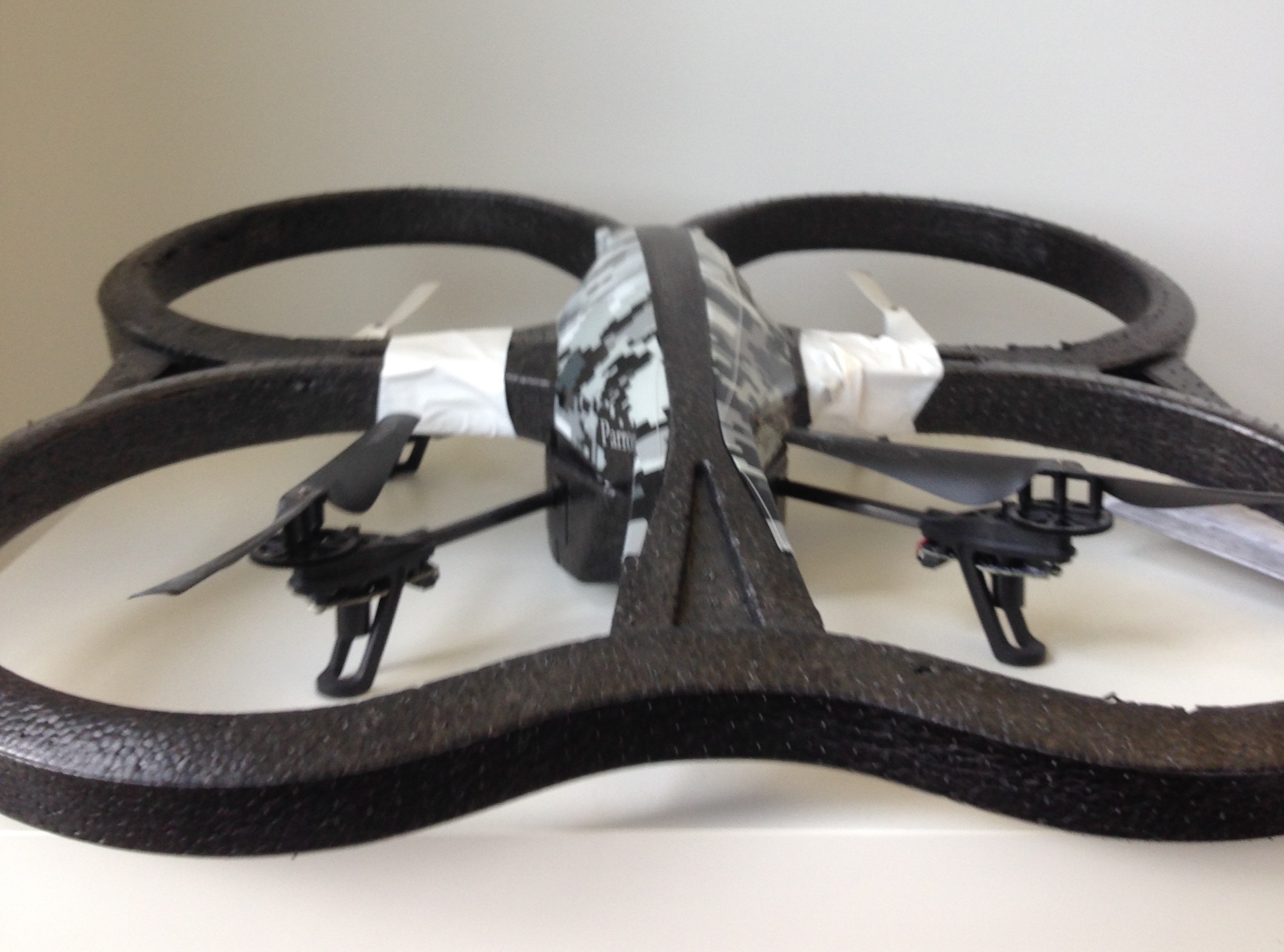
Duct Tape: More than a metaphor.
胶带:不仅仅是一个隐喻。
A couple of things I learned the hard way:
我学到了一些困难的方法:
- Build a helper app: After a few trial runs I built a helper app (see video below) to determine what/why things were happening. Let me tell you, this should’ve been step #1. It was invaluable being able to see what code my program was executing and what the processed images looked like.
- Don’t over-correct: For simple things like this, if you tell the drone to do too much at the same time, it freaks out and either (a) just sits there or (b) starts errantly flying all over the room (see video above).
- Always have an emergency landing script handy: I mentioned this earlier but it can’t be overemphasized. The reason is that if your program crashes and you haven’t landed your drone, you’re in deep … trouble. Your drone is going to keep flying (possibly errantly) until you tell it to land. Having
emergency-landing.js
handy will save you some maintenance (and possibly from a lawsuit). - If it’s windy, don’t fly your drone: Learned this one the really hard way…
- 构建一个辅助应用程序:经过几次试用运行,我构建了一个辅助应用程序(请参见下面的视频)以确定发生什么/为什么发生。 让我告诉你, 这应该是第一步 。 能够看到我的程序正在执行什么代码以及处理后的图像是什么样的,这是非常宝贵的。
- 请勿过度纠正:对于像这样的简单事情,如果您告诉无人机同时执行过多操作,它会吓跑,并且(a)只是坐在那里,或者(b)开始在整个房间内乱飞(参见上面的视频)。
- 始终准备好紧急着陆脚本:我之前已经提到过,但不要过分强调。 原因是,如果您的程序崩溃,而您还没有降落无人机,那么您将陷入严重的……麻烦。 您的无人机将继续飞行(可能是错误的),直到您告诉它降落。 方便使用
emergency-landing.js
将为您节省一些维护(并且可能会避免诉讼)。 - 如果有风,不要飞无人机:真的很难学到这一点……
In the end with some persistence and a little luck, I was able to get a couple of good autonomous runs in!
最后,有了一些毅力和一点运气,我得以加入了几个不错的自治系统!
[embedded content]
[嵌入内容]
在野外 (In the wild)
I wound up presenting this at PAPIs Valencia which was a lot of fun (BTW PAPIs is awesome! I highly recommend it for anyone interested in predictive analytics). Unfortunately my PAPIs demo didn’t go quite as smoothly. The lighting in the lecture hall was different than in our office and as a result, the red didn’t quite get filtered the same way. Despite the less than stellar performance, it was still a lot of fun!
我在Valencia的PAPI上介绍了这个过程,这很有趣(顺便说一句,PAPI太棒了!我强烈推荐给对预测分析感兴趣的人)。 不幸的是, 我的PAPI演示进行得并不顺利 。 演讲厅的灯光与我们办公室的灯光不同,因此红色的过滤效果并不完全相同。 尽管性能不及出色,但仍然很有趣!
资源资源 (Resources)
Want to learn more about programming your own drone? Here are some great resources for getting started:
想更多地了解如何为自己的无人机编程? 这里有一些很好的入门资源:
One for the road.
醉酒驾车。
翻译自: https://www.pybloggers.com/2016/04/building-a-semi-autonomous-drone-with-python/
使用python构建数据库
更多推荐
所有评论(0)