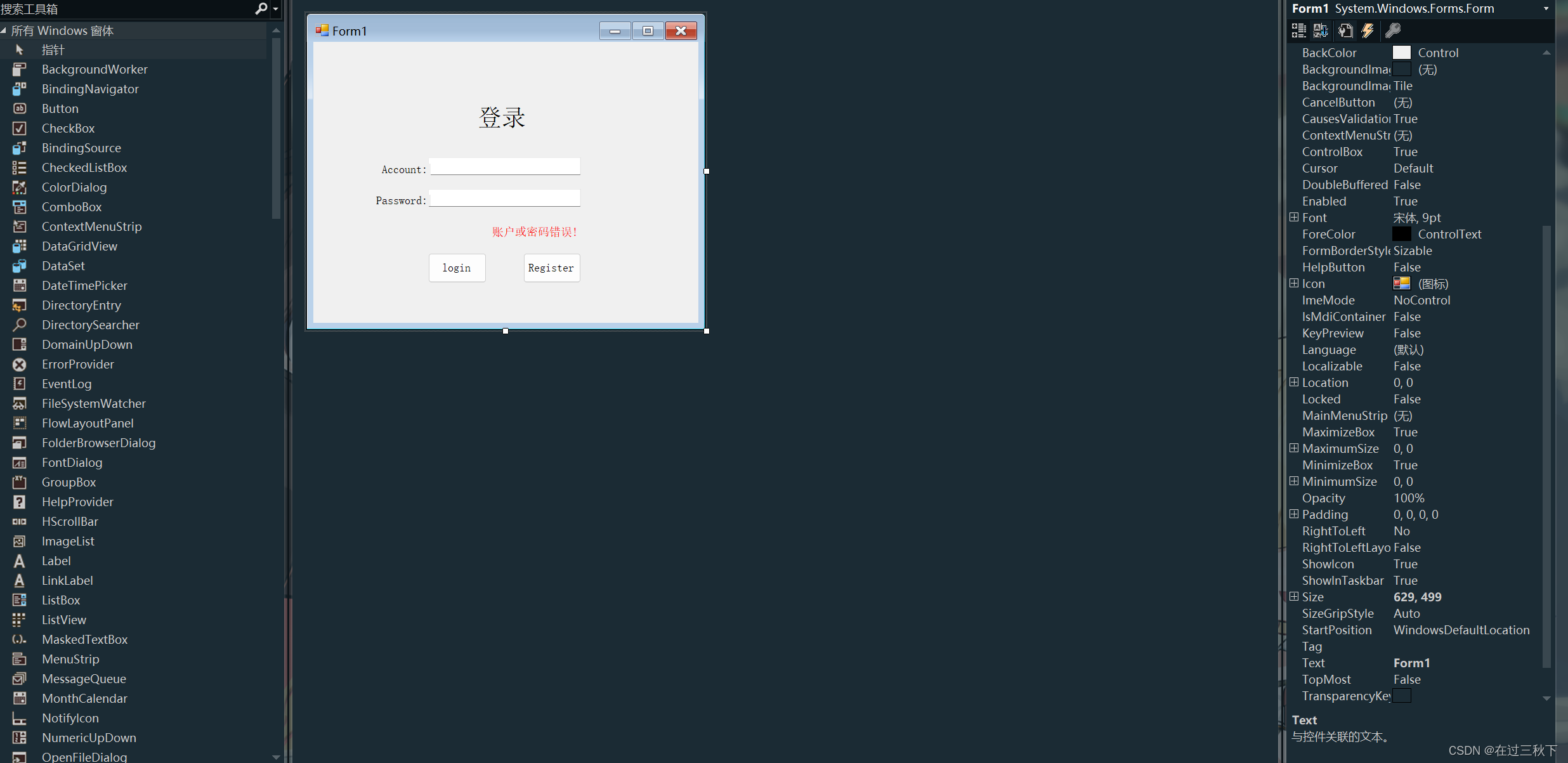
C#连接数据库实现登录注册功能
C#连接数据库实现登录注册功能(MySQL),需要一定的数据库操作基础
·
通过C#实现基本的登录及注册功能(需要一定数据库原理基础)
文章目录
没有目录工具
Visual Studio 2022
一、设计登录界面
a.使用Visual Studio创建项目
b.制作注册界面
using MySql.Data.MySqlClient;//引用MySql.Data,没有可以从MySQL官网下载(链接如下)
MySQL :: Download MySQL Connector/NET (Archived Versions)
以下为较为关键的代码
P1.连接MySQL
string mysqlcon = "server=本机服务器名;database=数据库名;user=MySQL账号;password=MySQL密码";
P2.建立MySqlConnection对象
MySqlConnection con = new MySqlConnection(mysqlcon);
P3. 执行con对象的函数,返回一个MySqlCommand类型的对象并把输入的数据拼接成sql语句交给cmd对象
在这里的information是我自己新建的表
MySqlCommand cmd = con.CreateCommand();
cmd.CommandText = "SELECT * FROM information";
MySqlDataReader dr = cmd.ExecuteReader();
P4.查找数据库内信息判断用户账户是否存在及密码正确(使用try-catch-finally)
while (dr.Read())
{
if (Account.Text.Trim() == dr["user"].ToString().Trim() && Password.Text.Trim() == dr["password"].ToString().Trim())
{
MessageBox.Show("登录成功!Welcome" + dr["name"].ToString().Trim());
loginSuccessful = true;
break; // 找到匹配项后退出循环
}
}
完整代码:
private void login_Click(object sender, EventArgs e)
{
string mysqlcon = "server=localhost;database=MyApplication;user=root;password=密码";
// 建立MySqlConnection对象
MySqlConnection con = new MySqlConnection(mysqlcon);
try
{
con.Open();
// 执行con对象的函数,返回一个MySqlCommand类型的对象
MySqlCommand cmd = con.CreateCommand();
// 把输入的数据拼接成sql语句,并交给cmd对象
cmd.CommandText = "SELECT * FROM information";
MySqlDataReader dr = cmd.ExecuteReader();
bool loginSuccessful = false;
while (dr.Read())
{
if (Account.Text.Trim() == dr["user"].ToString().Trim() && Password.Text.Trim() == dr["password"].ToString().Trim())
{
MessageBox.Show("登录成功!Welcome" + dr["name"].ToString().Trim());
loginSuccessful = true;
break; // 找到匹配项后退出循环
}
}
if (!loginSuccessful)
{
MessageBox.Show("用户名或密码错误!");
}
else{
Form3 main = new Form3();
this.Hide();
main.ShowDialog();
}
dr.Close();
}
catch (MySqlException ex)
{
MessageBox.Show("数据库连接或查询失败: " + ex.Message);
}
finally
{
con.Close(); // 确保连接被关闭,即使在出现异常的情况下也是如此
}
}
二、注册界面设计
注册界面操作基本和登录界面类似,在此仅提供区别的写入数据库代码
P1.注册界面
P2.区别代码:加入Allow User Variables=True(运行用户写入信息)
string connectionString = "server=localhost;database=MyFirstApplication;user=root;password=密码;Allow User Variables=True;";
P3.连接并写入信息(textBox1为账户,textBox2为密码)
connection.Open();
MySqlCommand cmd = new MySqlCommand();
cmd.Connection = connection;
// 用户表名为 'Information',且有 'user' 和 'password' 两个字段
cmd.CommandText = "INSERT INTO information (user, password) VALUES (@user, @password)";
cmd.Parameters.AddWithValue("@user", textBox1.Text);
cmd.Parameters.AddWithValue("@password", textBox2.Text);
cmd.ExecuteNonQuery(); // 执行插入操作
MessageBox.Show("新账户创建成功!");
P4.完整代码
private void button1_Click(object sender, EventArgs e)
{
string connectionString = "server=localhost;database=MyApplication;user=root;password=密码;Allow User Variables=True;";
string username = textBox1.Text;
string password = textBox2.Text;
using (MySqlConnection connection = new MySqlConnection(connectionString))
{
try
{
connection.Open();
MySqlCommand cmd = new MySqlCommand();
cmd.Connection = connection;
// 用户表名为 'Information',且有 'use' 和 'password' 两个字段
cmd.CommandText = "INSERT INTO information (user, password) VALUES (@user, @password)";
cmd.Parameters.AddWithValue("@user", textBox1.Text);
cmd.Parameters.AddWithValue("@password", textBox2.Text);
cmd.ExecuteNonQuery(); // 执行插入操作
MessageBox.Show("新账户创建成功!");
}
catch (Exception ex)
{
MessageBox.Show("创建账户时出错: " + ex.Message);
}
finally
{
connection.Close(); // 确保连接被关闭
}
}
}
编写时遇到的错误
1.创建账户失败:Fatal error encountered during command execution
解决方法:在string connectionString中加入Allow User Variables=True
2.Error【1146】:Table 'xxx.xxx' doesn't exist
解决方法:检查是否存在该表!
总结?
没有总结
更多推荐
所有评论(0)