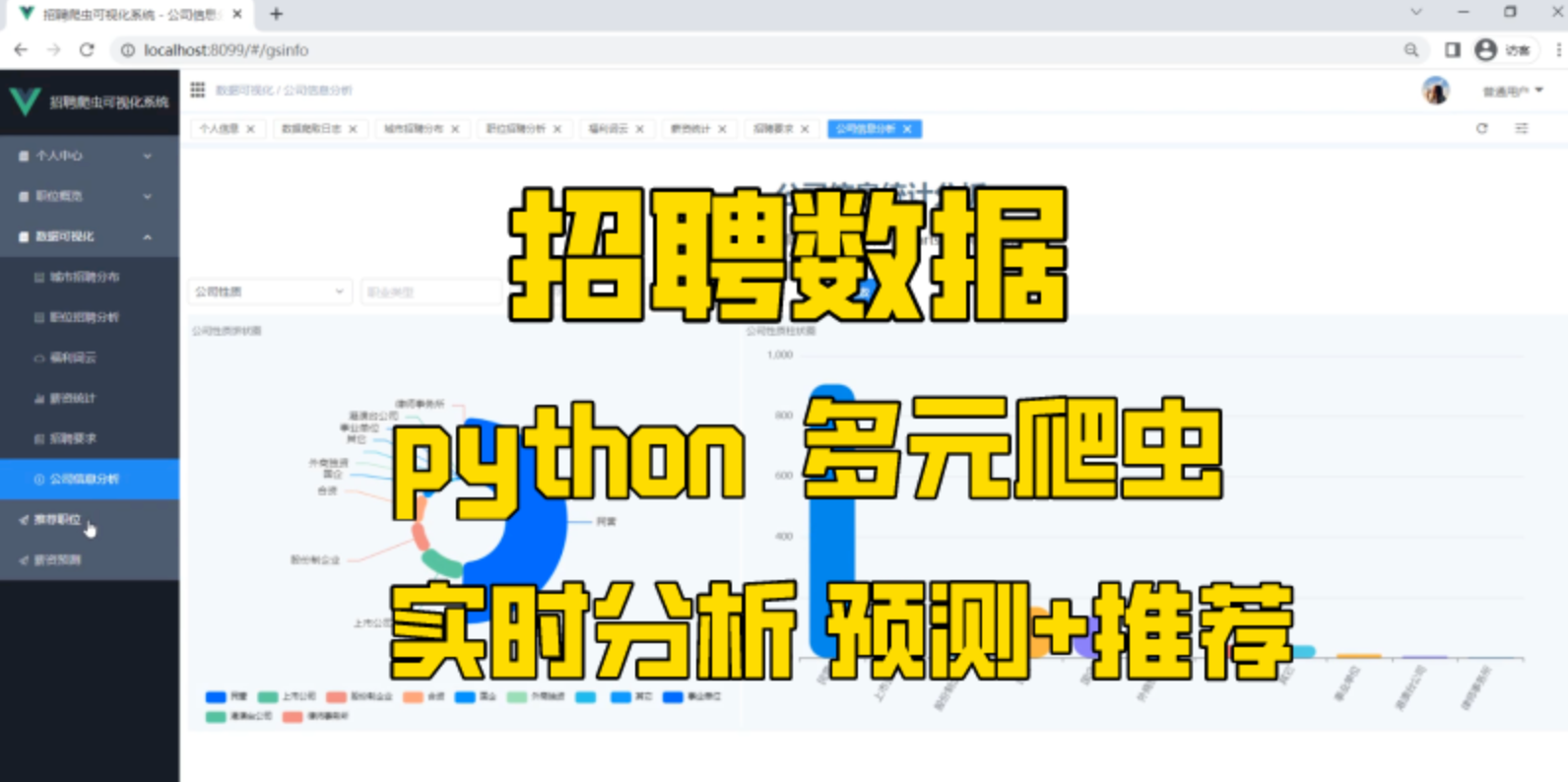
python招聘数据分析系统+爬虫+可视化 +Django框架+vue框架
python招聘数据分析系统+爬虫+可视化 +Django框架+vue框架
[毕业设计]2023-2024年最新最全计算机专业毕设选题推荐汇总
2023年 - 2024年 最新计算机毕业设计 本科 选题大全 汇总
感兴趣的可以先收藏起来,还有大家在毕设选题,项目以及论文编写等相关问题都可以给我留言咨询,希望帮助更多的人 。
1、项目介绍
此系统是一个实时分析招聘信息系统,应用Python爬虫、Flask框架、Echarts等技术实现。系统用户分为三类,管理员,普通用户和特殊用户(特殊用户指残疾人用户),普通用户和特殊用户的招聘数据来源不同,分别展示各自的数据。
项目技术栈:
Python招聘数据 薪资预测Flask框架 Echarts可视化大屏 Vue前端 智联招聘
2、项目界面
(1)招聘数据分析可视化
(2)数据概览
(3)数据爬虫模块
(4)薪资预测模块
3、项目说明
管理员功能
1、个人信息
浏览或修改个人信息
2、修改密码
修改登录密码
3、51 Job 数据概览
浏览正常用户招聘数据
4、残疾人招聘数据概览
浏览特殊用户招聘数据
5、51job数据获取
动态获取51job数据
6、数据爬取日志
查看数据爬取日志
7、用户管理
管理系统用户
普通用户功能
1、个人信息
浏览或修改个人信息
2、修改密码
修改登录密码
3、职位数据
将爬取到的数据进行展示
4、数据获取
动态获取51job数据
5、数据爬取日志
查看数据爬取日志
6、城市招聘分布
将数据通过工作城市进行分组,计算每个城市有多少条招聘信息,通过echarts柱状图进行展示
7、福利词云
分析公司福利, 用词云图进行展示
8、薪资统计
通过行业、学历要求和工作地点分析薪资占比
9、招聘要求
经验和学历两个维度分析各个行业的招聘要求占比
10、公司信息分析
对公司属性、公司性质、公司规模进行分析
11、推荐职位
根据个人信息系统推荐合适的职位
12、薪资预测
通过工资中位数对薪资进行预测
4、部分代码
from flask import Blueprint,request,jsonify
from data.mysqlHelper import get_a_conn
from data.data_zhilian import getZhilian
index_api = Blueprint('index_api', __name__)
# 51job城市字典表
@index_api.route('/getCityDict', methods=['POST'])
def getCityDict():
try:
mysql = get_a_conn()
sql = "select city_code value,city_name label from tbl_city"
res = mysql.fetchall(sql)
return jsonify({'code': '200', 'info': res})
except Exception as e:
return jsonify({'code': '500', 'info': e})
# 取消收藏
@index_api.route('/delCollect', methods=['POST'])
def delCollect():
try:
user_id = request.json.get('user_id')
job_id = int(request.json.get('job_id'))
mysql = get_a_conn()
sql = "delete from tbl_user_job where user_id = '%s' and job_id = '%s'" % (user_id, job_id)
mysql.fetchall(sql)
return jsonify({'code': '200', 'info': '取消成功'})
except Exception as e:
return jsonify({'code': '500', 'info': e})
# 收藏
@index_api.route('/collect', methods=['POST'])
def collect():
try:
user_id = request.json.get('user_id')
job_id = int(request.json.get('job_id'))
mysql = get_a_conn()
sql = "insert into tbl_user_job (user_id,job_id) values (%s,%s) " % (user_id, job_id)
mysql.fetchall(sql)
return jsonify({'code': '200', 'info': '新增成功'})
except Exception as e:
return jsonify({'code': '500', 'info': e})
# 获取日志
@index_api.route('/getLogs', methods=['POST'])
def getLogs():
pageno = int(request.json.get('pageNo', 1))
pagesize = int(request.json.get('pageSize', 10))
userRole = request.json.get('userRole')
userName = request.json.get('userName')
mysql = get_a_conn()
sql = "SELECT * FROM tbl_data_log where 1=1 "
if userRole != None and userRole != '' and userRole != '1':
sql += "and user_name = '" + str(userName) + "'"
sql += "ORDER BY end_time desc limit %s,%s" % ((pageno - 1) * pagesize, pagesize)
result = mysql.fetchall(sql)
sql_count = 'select count(1) num from tbl_data_log where 1=1 '
if userRole != None and userRole != '' and userRole != '1':
sql_count += "and user_name = '" + str(userName) + "'"
count = mysql.fetchall(sql_count)
total = count[0].get('num')
return jsonify({'code': '200', 'info': result, 'pageno': pageno, 'pagesize': pagesize, 'total': total})
# 爬取数据
@index_api.route('/getJobData', methods=['POST'])
def getJobs():
username = request.json.get('username')
search = request.json.get('search', '+')
pagesize = int(request.json.get('pageSize', 2))
city_id = request.json.get('city_id', 358)
if search == '':
search = ''
if city_id == '':
city_id = 358
result = getZhilian(username,city_id, search, pagesize)
return jsonify({'code': '200', 'info': result})
# 用户新增
@index_api.route('/addUser', methods=['POST'])
def addUser():
try:
account = request.json.get('account')
name = request.json.get('name')
email = request.json.get('email')
phone = request.json.get('phone')
role = request.json.get('role')
remarks = request.json.get('remarks')
mysql = get_a_conn()
sql = "insert into tbl_user (name,account,pwd,email,phone,login_flag,remarks,role) values ('%s','%s','%s','%s','%s','%s','%s','%s') " % (
name, account, '123456', email, phone, '1', remarks, role)
mysql.fetchall(sql)
return jsonify({'code': '200', 'info': '新增成功'})
except Exception as e:
return jsonify({'code': '500', 'info': e})
# 用户信息
@index_api.route('/userInfo', methods=['POST'])
def userInfo():
account = request.form.get("account")
mysql = get_a_conn()
sql = "SELECT * FROM tbl_user where 1=1 "
if account != None and account != '':
sql += " and account = '"
sql += account
sql += "'"
result = mysql.fetchall(sql)
sql_count = 'select count(1) num from tbl_user'
count = mysql.fetchall(sql_count)
total = count[0].get('num')
return jsonify({'code': '200', 'info': result})
# 用户编辑
@index_api.route('/editUser', methods=['POST'])
def editUser():
try:
print(request.form)
print('=============')
print(request.json)
id = request.json.get('id')
name = request.json.get('name')
email = request.json.get('email')
phone = request.json.get('phone')
role = request.json.get('role')
remarks = request.json.get('remarks')
icon = request.json.get('img')
location = '' if (request.json.get('location') == 'None' or request.json.get('location') == None) else request.json.get('location')
exp = '' if request.json.get('exp') == 'None' else request.json.get('exp')
deu = '' if request.json.get('deu') == 'None' else request.json.get('deu')
major = '' if request.json.get('major') == 'None' else request.json.get('major')
mysql = get_a_conn()
sql = "update tbl_user set name = '%s',email='%s',phone='%s',role='%s',remarks='%s',location='%s',exp='%s',deu='%s',major='%s',img='%s' where id = '%s'" % (
name, email, phone, role, remarks,location,exp,deu,major, icon,id)
mysql.fetchall(sql)
return jsonify({'code': '200', 'info': '修改成功'})
except Exception as e:
return jsonify({'code': '500', 'info': e})
# 用户停用
@index_api.route('/stopUser', methods=['POST'])
def stopUser():
try:
id = request.form.get('id')
if (id == None or id == '' or id == 'undefined'):
return jsonify({'code': '500', 'info': 'id不存在,启用失败'})
mysql = get_a_conn()
sql = "update tbl_user set login_flag = '0' where id = '%s'" % id
mysql.fetchall(sql)
return jsonify({'code': '200', 'info': '停用成功'})
except Exception as e:
return jsonify({'code': '500', 'info': e})
# 重置密码
@index_api.route('/chongzhi', methods=['POST'])
def chongzhi():
try:
id = request.form.get('id')
if (id == None or id == '' or id == 'undefined'):
return jsonify({'code': '500', 'info': 'id不存在,重置失败'})
mysql = get_a_conn()
sql = "update tbl_user set pwd = '123456' where id = '%s'" % id
mysql.fetchall(sql)
return jsonify({'code': '200', 'info': '重置成功,密码为 123456'})
except Exception as e:
return jsonify({'code': '500', 'info': e})
# 用户启用
@index_api.route('/startUser', methods=['POST'])
def startUser():
try:
id = request.form.get('id')
if (id == None or id == '' or id == 'undefined'):
return jsonify({'code': '500', 'info': 'id不存在,启用失败'})
mysql = get_a_conn()
sql = "update tbl_user set login_flag = '1' where id = '%s'" % id
mysql.fetchall(sql)
return jsonify({'code': '200', 'info': '启用成功'})
except Exception as e:
return jsonify({'code': '500', 'info': e})
# 用户删除
@index_api.route('/delUser', methods=['POST'])
def delUser():
try:
id = request.form.get('id')
if (id == None or id == '' or id == 'undefined'):
return jsonify({'code': '500', 'info': 'id不存在,删除失败'})
mysql = get_a_conn()
sql = "delete from tbl_user where id = '%s'" % id
mysql.fetchall(sql)
return jsonify({'code': '200', 'info': '删除成功'})
except Exception as e:
return jsonify({'code': '500', 'info': '删除失败' + e})
# 用户列表
@index_api.route('/getUsers', methods=['POST'])
def getUsers():
pageno = int(request.form.get('pageNo', 1))
pagesize = int(request.form.get('pageSize', 10))
name = request.form.get("name")
phone = request.form.get("phone")
mysql = get_a_conn()
sql = "SELECT * FROM tbl_user where 1=1 "
if name != None and name != '':
sql += " and name like '%"
sql += name
sql += "%'"
if phone != None and phone != '':
sql += " and phone = '%s'" % (phone)
sql += " ORDER BY id asc limit %s,%s" % ((pageno - 1) * pagesize, pagesize)
result = mysql.fetchall(sql)
sql_count = 'select count(1) num from tbl_user'
count = mysql.fetchall(sql_count)
total = count[0].get('num')
return jsonify({'code': '200', 'info': result, 'pageno': pageno, 'pagesize': pagesize, 'total': total})
# 修改密码
@index_api.route('/changePwd', methods=['POST'])
def changePwd():
account = request.form.get('account', None)
oldPwd = request.form.get('oldPwd', None)
newPwd = request.form.get('newPwd', None)
mysql = get_a_conn()
sql = """ SELECT * FROM tbl_user t where t.account = '%s' and t.pwd = '%s' """ % (account, oldPwd)
result = mysql.fetchall(sql)
if (result):
sql = "update tbl_user set pwd = '%s' WHERE account = '%s'" % (newPwd, account)
result1 = mysql.fetchall(sql)
print(result1)
return jsonify({'code': '200', 'info': '修改成功'})
else:
return jsonify({'code': '500', 'info': '旧密码输入不正确'})
# 注册
@index_api.route('/reg', methods=['POST'])
def reg():
try:
account = request.form.get('account', None)
pwd = request.form.get('pwd', None)
name = request.form.get('name', None)
role = request.form.get('role', None)
# 账号是否存在校验
mysql = get_a_conn()
sql_username = 'select count(1) count from tbl_user t where t.account = "%s"' % (account)
result = mysql.fetchall(sql_username)
if (result[0].get('count') > 0):
return jsonify({'code': '500', 'info': '该账号已注册'})
else:
sql = "INSERT into tbl_user (name,account,pwd,login_flag,role) values ('%s','%s','%s','%s','%s')" % (
name, account, pwd, '1', role)
mysql = get_a_conn()
mysql.fetchall(sql)
return jsonify({'code': '200', 'info': '注册成功,请登录'})
except Exception as e:
return jsonify({'code': '500', 'info': e})
# 登录
@index_api.route('/login', methods=['POST'])
def login():
account = request.form.get('account', None)
pwd = request.form.get('pwd', None)
mysql = get_a_conn()
sql = """ SELECT * FROM tbl_user t where t.account = '%s' and t.pwd = '%s' """ % (account, pwd)
result = mysql.fetchall(sql)
if (result):
return {'code': '200', 'info': '%s登录成功' % account, 'session':result}
else:
return {'code': '500', 'info': '%s登录失败' % account}
源码获取:
🍅由于篇幅限制,获取完整文章或源码、代做项目的,查看我的头像和用户名就可以找到我啦🍅
大家点赞、收藏、关注、评论啦 、查看👇🏻获取联系方式👇🏻
更多推荐
所有评论(0)