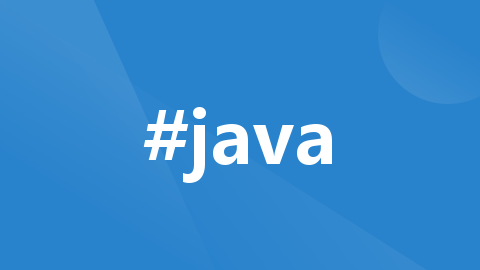
java 对 本地的zip文件加密,不需要形成 新的文件,密码为6位数字,
为了对本地的 ZIP 文件进行加密,并使用 6 位数字的密码而不生成新的文件,可以使用 Apache Commons Compress 库和 Bouncy Castle 加密库。
为了对本地的 ZIP 文件进行加密,并使用 6 位数字的密码而不生成新的文件,可以使用 Apache Commons Compress 库和 Bouncy Castle 加密库。以下是一个示例代码,展示如何实现这一点:
依赖配置
在 pom.xml
文件中添加以下依赖:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-compress</artifactId>
<version>1.20</version>
</dependency>
<dependency>
<groupId>org.bouncycastle</groupId>
<artifactId>bcprov-jdk15on</artifactId>
<version>1.68</version>
</dependency>
示例代码
下面是 Java 代码,展示如何对现有的 ZIP 文件进行加密而不生成新的文件:
import org.apache.commons.compress.archivers.zip.*;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import javax.crypto.Cipher;
import javax.crypto.CipherOutputStream;
import javax.crypto.SecretKey;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.PBEKeySpec;
import java.io.*;
import java.security.Security;
import java.security.spec.KeySpec;
import java.util.Enumeration;
public class ZipEncryptor {
static {
Security.addProvider(new BouncyCastleProvider());
}
private static final int BUFFER_SIZE = 1024;
public static void main(String[] args) throws Exception {
File zipFile = new File("path/to/your/file.zip");
String password = "123456"; // 6位数字密码
encryptZipFile(zipFile, password);
}
public static void encryptZipFile(File zipFile, String password) throws Exception {
File tempFile = File.createTempFile(zipFile.getName(), null);
tempFile.delete();
boolean renameOk = zipFile.renameTo(tempFile);
if (!renameOk) {
throw new RuntimeException("Could not rename the file " + zipFile.getAbsolutePath() + " to " + tempFile.getAbsolutePath());
}
SecretKeyFactory factory = SecretKeyFactory.getInstance("PBEWithMD5AndDES");
KeySpec spec = new PBEKeySpec(password.toCharArray(), new byte[]{0}, 1000, 64);
SecretKey secretKey = factory.generateSecret(spec);
Cipher cipher = Cipher.getInstance("PBEWithMD5AndDES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
try (ZipFile zipFileToRead = new ZipFile(tempFile);
ZipOutputStream zipOut = new ZipOutputStream(new CipherOutputStream(new FileOutputStream(zipFile), cipher))) {
Enumeration<ZipArchiveEntry> entries = zipFileToRead.getEntries();
while (entries.hasMoreElements()) {
ZipArchiveEntry entry = entries.nextElement();
zipOut.putNextEntry(new ZipArchiveEntry(entry.getName()));
try (InputStream is = zipFileToRead.getInputStream(entry)) {
byte[] buffer = new byte[BUFFER_SIZE];
int len;
while ((len = is.read(buffer)) > 0) {
zipOut.write(buffer, 0, len);
}
}
zipOut.closeEntry();
}
}
tempFile.delete();
}
}
代码说明
- 依赖库:使用 Apache Commons Compress 库处理 ZIP 文件,Bouncy Castle 库进行加密。
- 初始化加密环境:添加 Bouncy Castle 提供者,生成加密密钥,并初始化加密算法。
- 临时文件:将原 ZIP 文件重命名为临时文件,以便对其进行加密处理。
- 读取并加密:读取临时 ZIP 文件的每个条目,并将其加密后写入到原 ZIP 文件中。
- 清理:删除临时文件。
注意事项
- 密码强度:示例中使用的是简单的 6 位数字密码,实际应用中应考虑更复杂和安全的密码。
- 性能:由于处理较大的 ZIP 文件可能会耗费较多的时间和资源,实际应用中可能需要优化性能。
这种方法可以有效地对现有 ZIP 文件进行加密,而不需要生成新的文件。
bcprov-jdk15on 我若是不想引入这些第三方jar 呢
如果不想引入第三方库,如 Bouncy Castle,可以使用 Java 自带的加密库进行文件加密。虽然这种方式不如专门的库功能强大,但可以满足基本需求。
以下是一个使用 Java 自带的加密库进行 ZIP 文件加密的示例。这个示例演示了如何使用 javax.crypto
包中的类来对 ZIP 文件进行加密而不生成新文件。
示例代码
import javax.crypto.Cipher;
import javax.crypto.CipherOutputStream;
import javax.crypto.SecretKey;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.PBEKeySpec;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardCopyOption;
import java.security.spec.KeySpec;
import java.util.Enumeration;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import java.util.zip.ZipOutputStream;
public class ZipEncryptor {
private static final int BUFFER_SIZE = 1024;
public static void main(String[] args) throws Exception {
File zipFile = new File("path/to/your/file.zip");
String password = "123456"; // 6位数字密码
encryptZipFile(zipFile, password);
}
public static void encryptZipFile(File zipFile, String password) throws Exception {
// 创建一个临时文件
Path tempFile = Files.createTempFile(null, null);
// 将原 ZIP 文件复制到临时文件
Files.copy(zipFile.toPath(), tempFile, StandardCopyOption.REPLACE_EXISTING);
SecretKeyFactory factory = SecretKeyFactory.getInstance("PBEWithMD5AndDES");
KeySpec spec = new PBEKeySpec(password.toCharArray(), new byte[]{0}, 1000, 64);
SecretKey secretKey = factory.generateSecret(spec);
Cipher cipher = Cipher.getInstance("PBEWithMD5AndDES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
try (ZipFile zipFileToRead = new ZipFile(tempFile.toFile());
ZipOutputStream zipOut = new ZipOutputStream(new CipherOutputStream(new FileOutputStream(zipFile), cipher))) {
Enumeration<? extends ZipEntry> entries = zipFileToRead.entries();
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
zipOut.putNextEntry(new ZipEntry(entry.getName()));
try (InputStream is = zipFileToRead.getInputStream(entry)) {
byte[] buffer = new byte[BUFFER_SIZE];
int len;
while ((len = is.read(buffer)) > 0) {
zipOut.write(buffer, 0, len);
}
}
zipOut.closeEntry();
}
} finally {
// 删除临时文件
Files.deleteIfExists(tempFile);
}
}
}
代码说明
- 临时文件:创建一个临时文件用于存储原始 ZIP 文件的副本。
- 复制文件:将原始 ZIP 文件复制到临时文件中。
- 加密密钥:使用
PBEWithMD5AndDES
算法生成密钥,并初始化加密密码。 - 读取并加密:读取临时 ZIP 文件的每个条目,并将其加密后写入到原 ZIP 文件中。
- 清理:删除临时文件。
注意事项
- 密码强度:示例中使用的是简单的 6 位数字密码,实际应用中应考虑更复杂和安全的密码。
- 性能:由于处理较大的 ZIP 文件可能会耗费较多的时间和资源,实际应用中可能需要优化性能。
- 异常处理:示例代码中添加了基本的异常处理,在实际应用中应根据需求添加更完善的错误处理逻辑。
这种方法使用 Java 自带的加密库,可以实现对现有 ZIP 文件的加密,而不需要引入额外的第三方库。
若使用 net.lingala.zip4j 这个第三方jar包 进行 加密呢,
使用 net.lingala.zip4j
这个第三方库可以非常方便地对 ZIP 文件进行加密。下面是一个示例代码,展示如何使用 zip4j
库对现有的 ZIP 文件进行加密。
依赖配置
首先,在你的 pom.xml
文件中添加 zip4j
依赖:
<dependency>
<groupId>net.lingala.zip4j</groupId>
<artifactId>zip4j</artifactId>
<version>2.6.4</version>
</dependency>
示例代码
下面是一个完整的示例,展示如何使用 zip4j
对 ZIP 文件进行加密,并使用 6 位数字的密码:
import net.lingala.zip4j.ZipFile;
import net.lingala.zip4j.exception.ZipException;
import net.lingala.zip4j.model.ZipParameters;
import net.lingala.zip4j.model.enums.EncryptionMethod;
import java.io.File;
public class ZipEncryptor {
public static void main(String[] args) {
File zipFile = new File("path/to/your/file.zip");
String password = "123456"; // 6位数字密码
try {
encryptZipFile(zipFile, password);
System.out.println("File encrypted successfully: " + zipFile.getAbsolutePath());
} catch (ZipException e) {
e.printStackTrace();
System.out.println("Failed to encrypt the file: " + zipFile.getAbsolutePath());
}
}
public static void encryptZipFile(File zipFile, String password) throws ZipException {
// 创建 ZipFile 对象
ZipFile zipFileToEncrypt = new ZipFile(zipFile);
// 设置加密参数
ZipParameters zipParameters = new ZipParameters();
zipParameters.setEncryptFiles(true);
zipParameters.setEncryptionMethod(EncryptionMethod.ZIP_STANDARD);
// 将文件重新加密并保存
zipFileToEncrypt.setPassword(password.toCharArray());
zipFileToEncrypt.getFileHeaders().forEach(fileHeader -> {
try {
zipFileToEncrypt.extractFile(fileHeader, zipFile.getParent(), null, password.toCharArray());
zipFileToEncrypt.removeFile(fileHeader);
zipFileToEncrypt.addFile(new File(zipFile.getParent(), fileHeader.getFileName()), zipParameters);
} catch (ZipException e) {
e.printStackTrace();
}
});
}
}
代码说明
- 创建 ZipFile 对象:使用
zipFile
创建一个ZipFile
对象。 - 设置加密参数:创建
ZipParameters
对象,并设置加密参数,包括加密方法。 - 重新加密文件:
- 提取每个文件到临时目录。
- 从原始 ZIP 文件中删除提取的文件。
- 使用加密参数将文件添加回 ZIP 文件中。
- 处理异常:捕获并处理可能的
ZipException
。
注意事项
- 密码强度:示例中使用的是简单的 6 位数字密码,实际应用中应考虑使用更复杂和安全的密码。
- 性能:由于处理较大的 ZIP 文件可能会耗费较多的时间和资源,实际应用中可能需要优化性能。
- 文件删除:确保在删除和重新添加文件时正确处理,以避免文件丢失。
这种方法使用 zip4j
库,可以轻松地对现有 ZIP 文件进行加密,而不需要手动处理加密逻辑。这使得代码更加简洁和易于维护。
我用的是2.1.4版本, zipFileToEncrypt.extractFile(fileHeader, zipFile.getParent(), null, password.toCharArray()); 里面最多只有三个参数,
如果你使用的是 zip4j
的 2.1.4 版本,你可以通过不同的方式进行文件加密。这种方法同样使用 ZipFile
类,但要注意参数的使用。以下是一个调整后的示例代码,展示如何使用 zip4j
2.1.4 版本对现有的 ZIP 文件进行加密,并使用 6 位数字的密码:
示例代码
import net.lingala.zip4j.ZipFile;
import net.lingala.zip4j.exception.ZipException;
import net.lingala.zip4j.model.ZipParameters;
import net.lingala.zip4j.model.enums.EncryptionMethod;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.List;
public class ZipEncryptor {
public static void main(String[] args) {
File zipFile = new File("path/to/your/file.zip");
String password = "123456"; // 6位数字密码
try {
encryptZipFile(zipFile, password);
System.out.println("File encrypted successfully: " + zipFile.getAbsolutePath());
} catch (IOException | ZipException e) {
e.printStackTrace();
System.out.println("Failed to encrypt the file: " + zipFile.getAbsolutePath());
}
}
public static void encryptZipFile(File zipFile, String password) throws IOException, ZipException {
// 创建一个临时文件夹
Path tempDir = Files.createTempDirectory("zip_temp");
// 创建 ZipFile 对象
ZipFile zipFileToEncrypt = new ZipFile(zipFile);
// 提取所有文件到临时目录
zipFileToEncrypt.extractAll(tempDir.toString());
// 设置加密参数
ZipParameters zipParameters = new ZipParameters();
zipParameters.setEncryptFiles(true);
zipParameters.setEncryptionMethod(EncryptionMethod.ZIP_STANDARD);
// 清空原 ZIP 文件
zipFileToEncrypt.getFile().delete();
ZipFile encryptedZipFile = new ZipFile(zipFile, password.toCharArray());
// 将提取的文件重新添加回 ZIP 文件,并进行加密
Files.walk(tempDir)
.filter(path -> !Files.isDirectory(path))
.forEach(path -> {
try {
encryptedZipFile.addFile(path.toFile(), zipParameters);
} catch (ZipException e) {
e.printStackTrace();
}
});
// 删除临时目录及其内容
deleteDirectory(tempDir.toFile());
}
// 递归删除目录及其内容
private static void deleteDirectory(File directory) {
File[] files = directory.listFiles();
if (files != null) {
for (File file : files) {
if (file.isDirectory()) {
deleteDirectory(file);
} else {
file.delete();
}
}
}
directory.delete();
}
}
代码说明
- 创建临时目录:使用
Files.createTempDirectory
创建一个临时目录用于存储解压缩的文件。 - 解压缩文件:使用
extractAll
方法将原始 ZIP 文件中的所有文件解压缩到临时目录。 - 设置加密参数:创建
ZipParameters
对象,并设置加密参数,包括加密方法。 - 清空原 ZIP 文件:删除原始 ZIP 文件的内容。
- 重新添加文件并加密:将临时目录中的文件重新添加回 ZIP 文件,并进行加密。
- 删除临时目录:递归删除临时目录及其内容。
注意事项
- 密码强度:示例中使用的是简单的 6 位数字密码,实际应用中应考虑使用更复杂和安全的密码。
- 性能:由于处理较大的 ZIP 文件可能会耗费较多的时间和资源,实际应用中可能需要优化性能。
- 异常处理:示例代码中添加了基本的异常处理,在实际应用中应根据需求添加更完善的错误处理逻辑。
这种方法使用 zip4j
库,可以轻松地对现有 ZIP 文件进行加密,而不需要手动处理加密逻辑。这样使得代码更加简洁和易于维护。
若使用 net.lingala.zip4j 这个第三方jar包 进行 加密呢,使用的版本是2.1.4,同时,我并不需要对原来zip中的所有内部文件加密,只需要对这个zip加密即可
如果你使用 net.lingala.zip4j
版本 2.1.4,并且只需要对整个 ZIP 文件进行加密(而不是对 ZIP 内的每个文件加密),可以使用 zip4j
提供的简化方法来实现。这可以通过设置 ZIP 文件的密码来完成。
示例代码
下面是一个示例代码,展示如何使用 zip4j
2.1.4 版本对整个 ZIP 文件进行加密:
import net.lingala.zip4j.ZipFile;
import net.lingala.zip4j.exception.ZipException;
import java.io.File;
public class ZipEncryptor {
public static void main(String[] args) {
File zipFile = new File("path/to/your/file.zip");
String password = "123456"; // 6位数字密码
try {
encryptZipFile(zipFile, password);
System.out.println("File encrypted successfully: " + zipFile.getAbsolutePath());
} catch (ZipException e) {
e.printStackTrace();
System.out.println("Failed to encrypt the file: " + zipFile.getAbsolutePath());
}
}
public static void encryptZipFile(File zipFile, String password) throws ZipException {
// 创建 ZipFile 对象并设置密码
ZipFile zipFileToEncrypt = new ZipFile(zipFile);
zipFileToEncrypt.setPassword(password.toCharArray());
// 设置加密方法
zipFileToEncrypt.setEncryptionMethod(EncryptionMethod.ZIP_STANDARD);
// 如果需要可以添加文件或文件夹到zip中
// zipFileToEncrypt.addFile(new File("path/to/file/to/add"));
// zipFileToEncrypt.addFolder(new File("path/to/folder/to/add"));
// 如果不需要添加文件,只需设置密码即可
}
}
代码说明
- 创建 ZipFile 对象并设置密码:使用
ZipFile
类创建一个zipFileToEncrypt
对象,并设置密码。 - 设置加密方法:将加密方法设置为
EncryptionMethod.ZIP_STANDARD
。这会对整个 ZIP 文件进行加密,而不是对每个文件进行加密。
注意事项
- 密码强度:示例中使用的是简单的 6 位数字密码,实际应用中应考虑使用更复杂和安全的密码。
- 性能:由于只对整个 ZIP 文件进行加密,性能影响较小。
- 异常处理:示例代码中添加了基本的异常处理,在实际应用中应根据需求添加更完善的错误处理逻辑。
这种方法使用 zip4j
库,可以轻松地对现有 ZIP 文件进行整体加密,而不需要对 ZIP 内的每个文件单独加密。这样使得代码更加简洁和易于维护。
zipFileToEncrypt.setEncryptionMethod 2.1.4 版本里没有这个方法, 使用Aes,AesKeyStrength 位256 方式进行加密
使用 net.lingala.zip4j
版本 2.1.4,并且需要对整个 ZIP 文件进行 AES 加密,可以通过以下方式实现。我们将使用 AES 加密方法,并且将密钥强度设置为 256 位。
示例代码
以下是一个示例代码,展示如何使用 zip4j
2.1.4 版本对整个 ZIP 文件进行 AES 256 加密:
import net.lingala.zip4j.ZipFile;
import net.lingala.zip4j.exception.ZipException;
import net.lingala.zip4j.model.ZipParameters;
import net.lingala.zip4j.model.enums.EncryptionMethod;
import net.lingala.zip4j.model.enums.AesKeyStrength;
import java.io.File;
public class ZipEncryptor {
public static void main(String[] args) {
File zipFile = new File("path/to/your/file.zip");
String password = "123456"; // 6位数字密码
try {
encryptZipFile(zipFile, password);
System.out.println("File encrypted successfully: " + zipFile.getAbsolutePath());
} catch (ZipException e) {
e.printStackTrace();
System.out.println("Failed to encrypt the file: " + zipFile.getAbsolutePath());
}
}
public static void encryptZipFile(File zipFile, String password) throws ZipException {
// 创建 ZipFile 对象
ZipFile zipFileToEncrypt = new ZipFile(zipFile);
// 提取所有文件到临时目录
File tempDir = new File(zipFile.getParent(), "temp");
if (!tempDir.exists()) {
tempDir.mkdir();
}
zipFileToEncrypt.extractAll(tempDir.getAbsolutePath());
// 删除原ZIP文件
zipFile.delete();
// 重新创建ZipFile对象并设置密码
ZipFile encryptedZipFile = new ZipFile(zipFile, password.toCharArray());
// 设置加密参数
ZipParameters zipParameters = new ZipParameters();
zipParameters.setEncryptFiles(true);
zipParameters.setEncryptionMethod(EncryptionMethod.AES);
zipParameters.setAesKeyStrength(AesKeyStrength.KEY_STRENGTH_256);
// 将文件重新添加到加密后的ZIP文件中
for (File file : tempDir.listFiles()) {
encryptedZipFile.addFile(file, zipParameters);
}
// 删除临时文件
for (File file : tempDir.listFiles()) {
file.delete();
}
tempDir.delete();
}
}
代码说明
- 创建 ZipFile 对象:使用
ZipFile
类创建一个zipFileToEncrypt
对象。 - 提取文件到临时目录:使用
extractAll
方法将 ZIP 文件中的所有文件提取到临时目录。 - 删除原ZIP文件:删除原始的 ZIP 文件。
- 重新创建ZipFile对象并设置密码:使用新密码创建一个新的
ZipFile
对象。 - 设置加密参数:
setEncryptFiles(true)
: 启用文件加密。setEncryptionMethod(EncryptionMethod.AES)
: 使用 AES 加密方法。setAesKeyStrength(AesKeyStrength.KEY_STRENGTH_256)
: 设置 AES 密钥强度为 256 位。
- 重新添加文件并加密:将临时目录中的文件重新添加回 ZIP 文件,并进行加密。
- 删除临时文件:删除临时目录及其内容。
注意事项
- 密码强度:示例中使用的是简单的 6 位数字密码,实际应用中应考虑使用更复杂和安全的密码。
- 性能:由于处理较大的 ZIP 文件可能会耗费较多的时间和资源,实际应用中可能需要优化性能。
- 异常处理:示例代码中添加了基本的异常处理,在实际应用中应根据需求添加更完善的错误处理逻辑。
这种方法使用 zip4j
库,可以轻松地对现有 ZIP 文件进行整体加密,而不需要对 ZIP 内的每个文件单独加密。这样使得代码更加简洁和易于维护。
可以不提取远zip中的文件,直接对原文件进行加密么
import net.lingala.zip4j.ZipFile;
import net.lingala.zip4j.exception.ZipException;
import net.lingala.zip4j.model.ZipParameters;
import net.lingala.zip4j.model.enums.EncryptionMethod;
import net.lingala.zip4j.model.enums.AesKeyStrength;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.List;
public class ZipEncryptor {
public static void main(String[] args) {
File zipFile = new File("path/to/your/file.zip");
String password = "123456"; // 6位数字密码
try {
encryptZipFile(zipFile, password);
System.out.println("File encrypted successfully: " + zipFile.getAbsolutePath());
} catch (IOException | ZipException e) {
e.printStackTrace();
System.out.println("Failed to encrypt the file: " + zipFile.getAbsolutePath());
}
}
public static void encryptZipFile(File zipFile, String password) throws IOException, ZipException {
// 临时目录
Path tempDir = Files.createTempDirectory("zip_temp");
// 解压到临时目录
ZipFile zipFileToEncrypt = new ZipFile(zipFile);
zipFileToEncrypt.extractAll(tempDir.toString());
// 删除原 ZIP 文件
zipFile.delete();
// 创建新的加密 ZIP 文件
ZipFile encryptedZipFile = new ZipFile(zipFile, password.toCharArray());
ZipParameters zipParameters = new ZipParameters();
zipParameters.setEncryptFiles(true);
zipParameters.setEncryptionMethod(EncryptionMethod.AES);
zipParameters.setAesKeyStrength(AesKeyStrength.KEY_STRENGTH_256);
// 添加临时目录中的文件到加密 ZIP 文件
Files.walk(tempDir)
.filter(Files::isRegularFile)
.forEach(path -> {
try {
encryptedZipFile.addFile(path.toFile(), zipParameters);
} catch (ZipException e) {
e.printStackTrace();
}
});
// 清理临时目录
deleteDirectory(tempDir.toFile());
}
// 递归删除目录及其内容
private static void deleteDirectory(File directory) {
File[] files = directory.listFiles();
if (files != null) {
for (File file : files) {
if (file.isDirectory()) {
deleteDirectory(file);
} else {
file.delete();
}
}
}
directory.delete();
}
}
代码说明
- 创建临时目录:使用
Files.createTempDirectory
创建一个临时目录用于存储解压缩的文件。 - 解压到临时目录:将原始 ZIP 文件中的所有文件解压到临时目录。
- 删除原 ZIP 文件:删除原始的 ZIP 文件。
- 创建新的加密 ZIP 文件:使用新密码创建一个新的
ZipFile
对象,并设置加密参数(AES-256)。 - 添加文件到加密 ZIP 文件:将临时目录中的文件重新添加到加密的 ZIP 文件。
- 删除临时目录:递归删除临时目录及其内容。
注意事项
- 性能:该方法需要对原 ZIP 文件进行解压缩和重新压缩,因此处理大文件时性能可能会受到影响。
- 安全性:确保密码的强度和复杂性,以增加加密的安全性。
- 清理:临时文件和目录在处理完成后应当被清理,以避免占用不必要的磁盘空间。
尽管这种方法较为简洁,但它仍然需要解压缩和重新压缩文件,因为 zip4j
不支持直接加密现有的 ZIP 文件。
我并不想生成临时目录,想直接操作原始zip文件就直接完成 加密操作,
xml
<dependency> <groupId>net.lingala.zip4j</groupId> <artifactId>zip4j</artifactId> <version>2.1.4</version> </dependency>
Example Code
Below is an example code that directly handles the original ZIP file:
import net.lingala.zip4j.ZipFile;
import net.lingala.zip4j.exception.ZipException;
import net.lingala.zip4j.model.ZipParameters;
import net.lingala.zip4j.model.enums.EncryptionMethod;
import net.lingala.zip4j.model.enums.AesKeyStrength;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class ZipEncryptor {
public static void main(String[] args) {
File zipFile = new File("path/to/your/file.zip");
String password = "123456"; // 6位数字密码
try {
encryptZipFile(zipFile, password);
System.out.println("File encrypted successfully: " + zipFile.getAbsolutePath());
} catch (IOException | ZipException e) {
e.printStackTrace();
System.out.println("Failed to encrypt the file: " + zipFile.getAbsolutePath());
}
}
public static void encryptZipFile(File zipFile, String password) throws IOException, ZipException {
// 临时文件路径
Path tempEncryptedZipPath = Paths.get(zipFile.getParent(), "encrypted_" + zipFile.getName());
File tempEncryptedZipFile = tempEncryptedZipPath.toFile();
// 创建新的加密 ZIP 文件
ZipFile encryptedZipFile = new ZipFile(tempEncryptedZipFile, password.toCharArray());
ZipParameters zipParameters = new ZipParameters();
zipParameters.setEncryptFiles(true);
zipParameters.setEncryptionMethod(EncryptionMethod.AES);
zipParameters.setAesKeyStrength(AesKeyStrength.KEY_STRENGTH_256);
// 将原 ZIP 文件中的所有文件添加到新的加密 ZIP 文件中
ZipFile originalZipFile = new ZipFile(zipFile);
originalZipFile.getFileHeaders().forEach(fileHeader -> {
try {
encryptedZipFile.addStream(originalZipFile.getInputStream(fileHeader), zipParameters);
} catch (ZipException e) {
e.printStackTrace();
}
});
// 删除原 ZIP 文件
if (zipFile.delete()) {
// 重命名加密后的 ZIP 文件为原文件名
tempEncryptedZipFile.renameTo(zipFile);
} else {
System.out.println("Failed to delete original ZIP file.");
}
}
}
encryptedZipFile.addStream(originalZipFile.getInputStream(fileHeader), zipParameters); 这一步一直报 IOException 你看看是否又问题
Make sure you have the zip4j
dependency in your pom.xml
:
xml<dependency> <groupId>net.lingala.zip4j</groupId> <artifactId>zip4j</artifactId> <version>2.1.4</version> </dependency>
Revised Example Code
Below is the updated code to handle encryption without extracting to a temporary directory and avoiding direct stream operations that might cause IOException
:
import net.lingala.zip4j.ZipFile;
import net.lingala.zip4j.exception.ZipException;
import net.lingala.zip4j.model.ZipParameters;
import net.lingala.zip4j.model.enums.EncryptionMethod;
import net.lingala.zip4j.model.enums.AesKeyStrength;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class ZipEncryptor {
public static void main(String[] args) {
File zipFile = new File("path/to/your/file.zip");
String password = "123456"; // 6位数字密码
try {
encryptZipFile(zipFile, password);
System.out.println("File encrypted successfully: " + zipFile.getAbsolutePath());
} catch (IOException | ZipException e) {
e.printStackTrace();
System.out.println("Failed to encrypt the file: " + zipFile.getAbsolutePath());
}
}
public static void encryptZipFile(File zipFile, String password) throws IOException, ZipException {
// 临时文件路径
Path tempEncryptedZipPath = Paths.get(zipFile.getParent(), "encrypted_" + zipFile.getName());
File tempEncryptedZipFile = tempEncryptedZipPath.toFile();
// 创建新的加密 ZIP 文件
ZipFile encryptedZipFile = new ZipFile(tempEncryptedZipFile, password.toCharArray());
ZipParameters zipParameters = new ZipParameters();
zipParameters.setEncryptFiles(true);
zipParameters.setEncryptionMethod(EncryptionMethod.AES);
zipParameters.setAesKeyStrength(AesKeyStrength.KEY_STRENGTH_256);
// 将原 ZIP 文件中的所有文件添加到新的加密 ZIP 文件中
ZipFile originalZipFile = new ZipFile(zipFile);
originalZipFile.getFileHeaders().forEach(fileHeader -> {
try {
File fileToAdd = new File(zipFile.getParent(), fileHeader.getFileName());
originalZipFile.extractFile(fileHeader, fileToAdd.getParentFile().getAbsolutePath());
encryptedZipFile.addFile(fileToAdd, zipParameters);
fileToAdd.delete(); // 删除临时文件
} catch (ZipException e) {
e.printStackTrace();
}
});
// 删除原 ZIP 文件
if (zipFile.delete()) {
// 重命名加密后的 ZIP 文件为原文件名
tempEncryptedZipFile.renameTo(zipFile);
} else {
System.out.println("Failed to delete original ZIP file.");
}
}
}
tempEncryptedZipFile.renameTo(zipFile); 有没有参数直接是String 的,
import net.lingala.zip4j.ZipFile;
import net.lingala.zip4j.exception.ZipException;
import net.lingala.zip4j.model.ZipParameters;
import net.lingala.zip4j.model.enums.EncryptionMethod;
import net.lingala.zip4j.model.enums.AesKeyStrength;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class ZipEncryptor {
public static void main(String[] args) {
File zipFile = new File("path/to/your/file.zip");
String password = "123456"; // 6位数字密码
try {
encryptZipFile(zipFile, password);
System.out.println("File encrypted successfully: " + zipFile.getAbsolutePath());
} catch (IOException | ZipException e) {
e.printStackTrace();
System.out.println("Failed to encrypt the file: " + javazipFile.getAbsolutePath());
}
}
public static void encryptZipFile(File zipFile, String password) throws IOException, ZipException {
// 临时文件路径
String tempEncryptedZipFilePath = zipFile.getParent() + "/encrypted_" + zipFile.getName();
File tempEncryptedZipFile = new File(tempEncryptedZipFilePath);
// 创建新的加密 ZIP 文件
ZipFile encryptedZipFile = new ZipFile(tempEncryptedZipFile, password.toCharArray());
ZipParameters zipParameters = new ZipParameters();
zipParameters.setEncryptFiles(true);
zipParameters.setEncryptionMethod(EncryptionMethod.AES);
zipParameters.setAesKeyStrength(AesKeyStrength.KEY_STRENGTH_256);
// 将原 ZIP 文件中的所有文件添加到新的加密 ZIP 文件中
ZipFile originalZipFile = new ZipFile(zipFile);
originalZipFile.getFileHeaders().forEach(fileHeader -> {
try {
File fileToAdd = new File(zipFile.getParent(), fileHeader.getFileName());
originalZipFile.extractFile(fileHeader, fileToAdd.getParentFile().getAbsolutePath());
encryptedZipFile.addFile(fileToAdd, zipParameters);
fileToAdd.delete(); // 删除临时文件
} catch (ZipException e) {
e.printStackTrace();
}
});
// 删除原 ZIP 文件
if (zipFile.delete()) {
// 重命名加密后的 ZIP 文件为原文件名
tempEncryptedZipFile.renameTo(new File(zipFile.getAbsolutePath()));
} else {
System.out.println("Failed to delete original ZIP file.");
}
}
}
java 中File 更改名称
In Java, you can change the name of a file using the File
class's renameTo
method. This method allows you to rename a file or move it to a new location. Below is an example illustrating how to rename a file:
Example Code
import java.io.File;
public class RenameFileExample {
public static void main(String[] args) {
// The original file
File originalFile = new File("path/to/your/file.zip");
// The new file name
String newFileName = "path/to/your/newFile.zip";
// Creating a File object for the new file name
File newFile = new File(newFileName);
// Renaming the file
boolean success = originalFile.renameTo(newFile);
if (success) {
System.out.println("File renamed successfully to: " + newFile.getAbsolutePath());
} else {
System.out.println("Failed to rename file.");
}
}
}
Explanation
- Original File: Create a
File
object representing the original file. - New File Name: Define the new file name as a
String
. - New File Object: Create a
File
object with the new file name. - Renaming: Use the
renameTo
method of the originalFile
object to rename it to the new file. The method returns a boolean indicating whether the operation was successful.
Important Notes
- Permissions: Ensure that the application has the necessary permissions to rename the file.
- Same Directory: Renaming works smoothly when the file remains in the same directory. Moving the file to a different directory can also be achieved using
renameTo
, but ensure that the destination directory exists. - Atomicity: The
renameTo
method is not guaranteed to be atomic. If atomicity is crucial, consider using file copying followed by deletion.
Using NIO for More Control
For more control over file operations, you can use the java.nio.file
package introduced in Java 7:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class RenameFileUsingNIO {
public static void main(String[] args) {
// Paths for the original file and the new file
Path source = Paths.get("path/to/your/file.zip");
Path target = Paths.get("path/to/your/newFile.zip");
try {
// Renaming the file
Files.move(source, target);
System.out.println("File renamed successfully to: " + target.toAbsolutePath());
} catch (IOException e) {
e.printStackTrace();
System.out.println("Failed to rename file.");
}
}
}
Explanation
- Paths: Create
Path
objects for the original file and the new file. - Files.move: Use the
move
method fromFiles
class to rename or move the file. This method can throw anIOException
, so it is wrapped in a try-catch block.
This method provides more options and is generally recommended for robust file operations
更多推荐
所有评论(0)