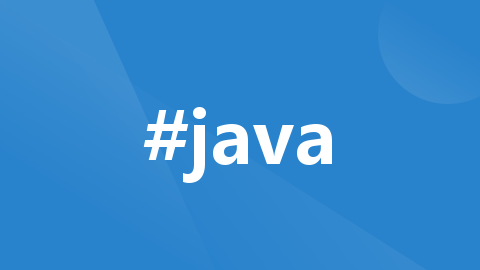
使用java解析xmind文件
使用java解析xmind,新老版本都支持,还可解析图片
·
1、引入依赖
com.github.eljah 解析xmind核心库,后边两个依赖主要是为了防止jar包冲突引入
<dependency>
<groupId>com.github.eljah</groupId>
<artifactId>xmindjbehaveplugin</artifactId>
<exclusions>
<exclusion>
<groupId>org.apache.maven</groupId>
<artifactId>maven-plugin-api</artifactId>
</exclusion>
<exclusion>
<groupId>org.codehaus.plexus</groupId>
<artifactId>plexus-utils</artifactId>
</exclusion>
</exclusions>
<version>0.8</version>
</dependency>
<dependency>
<groupId>org.dom4j</groupId>
<artifactId>dom4j</artifactId>
<version>2.1.3</version>
</dependency>
<dependency>
<groupId>jaxen</groupId>
<artifactId>jaxen</artifactId>
<version>1.2.0</version>
</dependency>
2、建立文件夹Xmind引入以下文件
XmindLegacy.java
package com.bj58.fangcase.fangcasemanage.util.xmind;
import org.dom4j.*;
import org.json.JSONException;
import org.json.JSONObject;
import org.json.XML;
import java.io.IOException;
import java.util.List;
/**
* @author liufree liufreeo@gmail.com
* @Classname XmindLegacy
* @Description TODO
* @Date 2020/4/28 10:26
*/
public class XmindLegacy {
/**
* 返回content.xml和comments.xml合并后的json
*
* @param xmlContent
* @param xmlComments
* @return
* @throws IOException
* @throws DocumentException
*/
public static String getContent(String xmlContent, String xmlComments) throws IOException, DocumentException, JSONException {
//删除content.xml里面不能识别的字符串
xmlContent = xmlContent.replace("xmlns=\"urn:xmind:xmap:xmlns:content:2.0\"", "");
xmlContent = xmlContent.replace("xmlns:fo=\"http://www.w3.org/1999/XSL/Format\"", "");
//删除<topic>节点
xmlContent = xmlContent.replace("<topics type=\"attached\">", "");
xmlContent = xmlContent.replace("</topics>", "");
//去除title中svg:width属性
xmlContent = xmlContent.replaceAll("<title svg:width=\"[0-9]*\">", "<title>");
Document document = DocumentHelper.parseText(xmlContent);// 读取XML文件,获得document对象
Element root = document.getRootElement();
List<Node> topics = root.selectNodes("//topic");
if (xmlComments != null) {
//删除comments.xml里面不能识别的字符串
xmlComments = xmlComments.replace("xmlns=\"urn:xmind:xmap:xmlns:comments:2.0\"", "");
//添加评论到content中
Document commentDocument = DocumentHelper.parseText(xmlComments);
List<Node> commentsList = commentDocument.selectNodes("//comment");
for (Node topic : topics) {
for (Node commentNode : commentsList) {
Element commentElement = (Element) commentNode;
Element topicElement = (Element) topic;
if (topicElement.attribute("id").getValue().equals(commentElement.attribute("object-id").getValue())) {
Element comment = topicElement.addElement("comments");
comment.addAttribute("creationTime", commentElement.attribute("time").getValue());
comment.addAttribute("author", commentElement.attribute("author").getValue());
comment.addAttribute("content", commentElement.element("content").getText());
}
}
}
}
//第一个topic转换为json中的rootTopic
Node rootTopic = root.selectSingleNode("/xmap-content/sheet/topic");
rootTopic.setName("rootTopic");
//将xml中topic节点转换为attached节点
List<Node> topicList = rootTopic.selectNodes("//topic");
for (Node node : topicList) {
node.setName("attached");
}
//选取第一个sheet
Element sheet = (Element) root.elements("sheet").get(0);
String res = sheet.asXML();
//将xml转为json
JSONObject xmlJSONObj = XML.toJSONObject(res);
JSONObject jsonObject = xmlJSONObj.getJSONObject("sheet");
//设置缩进
return jsonObject.toString(4);
}
}
XmindParser.java
package com.bj58.fangcase.fangcasemanage.util.xmind;
import com.alibaba.fastjson.JSON;
import com.bj58.fangcase.fangcasemanage.util.xmind.pojo.JsonRootBean;
import com.esotericsoftware.minlog.Log;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.compress.archivers.ArchiveException;
import org.dom4j.DocumentException;
import org.json.JSONException;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* @author liufree liufreeo@gmail.com
* @Classname XmindParser
* @Description 解析主体
* @Date 2020/4/27 14:05
*/
@Slf4j
public class XmindParser {
public static final String xmindZenJson = "content.json";
public static final String xmindLegacyContent = "content.xml";
public static final String xmindLegacyComments = "comments.xml";
public static String filePath="";
/**
* 解析脑图文件,返回content整合后的内容
*
* @param xmindFile
* @return
* @throws IOException
* @throws ArchiveException
* @throws DocumentException
*/
public static String parseJson(String xmindFile) throws IOException, ArchiveException, DocumentException, JSONException {
String res = ZipUtils.extract(xmindFile);
filePath=res;
log.info(filePath);
String content = null;
if (isXmindZen(res, xmindFile)) {
content = getXmindZenContent(xmindFile, res);
log.info("1111 = " + content);
} else {
content = getXmindLegacyContent(xmindFile, res);
content = content.replaceAll("xhtml:img","image");
content = content.replaceAll("xhtml:src","src");
log.info("老版本吗-------" + JSON.toJSONString(content, true));
}
//删除生成的文件夹
File dir = new File(res);
// boolean flag = deleteDir(dir);
// if (flag) {
// // do something
// }
String strContent = content.replaceAll("-", "");
JsonRootBean jsonRootBean = JSON.parseObject(strContent, JsonRootBean.class);
return (JSON.toJSONString(jsonRootBean, false));
}
public static Object parseObject(String xmindFile) throws DocumentException, ArchiveException, IOException, JSONException {
String content = parseJson(xmindFile);
JsonRootBean jsonRootBean = JSON.parseObject(content, JsonRootBean.class);
return jsonRootBean;
}
public static boolean deleteDir(File dir) {
if (dir.isDirectory()) {
String[] children = dir.list();
//递归删除目录中的子目录下
for (int i = 0; i < children.length; i++) {
boolean success = deleteDir(new File(dir, children[i]));
if (!success) {
return false;
}
}
}
// 目录此时为空,可以删除
return dir.delete();
}
/**
* @return
*/
public static String getXmindZenContent(String xmindFile, String extractFileDir) throws IOException, ArchiveException {
List<String> keys = new ArrayList<>();
keys.add(xmindZenJson);
Map<String, String> map = ZipUtils.getContents(keys, xmindFile, extractFileDir);
String content = map.get(xmindZenJson);
// content = content.substring(1, content.lastIndexOf("]"));
content = XmindZen.getContent(content);
return content;
}
/**
* @return
*/
public static String getXmindLegacyContent(String xmindFile, String extractFileDir) throws IOException, ArchiveException, DocumentException, JSONException {
List<String> keys = new ArrayList<>();
keys.add(xmindLegacyContent);
keys.add(xmindLegacyComments);
Map<String, String> map = ZipUtils.getContents(keys, xmindFile, extractFileDir);
String contentXml = map.get(xmindLegacyContent);
String commentsXml = map.get(xmindLegacyComments);
String xmlContent = XmindLegacy.getContent(contentXml, commentsXml);
return xmlContent;
}
private static boolean isXmindZen(String res, String xmindFile) throws IOException, ArchiveException {
//解压
File parent = new File(res);
if (parent.isDirectory()) {
String[] files = parent.list(new ZipUtils.FileFilter());
for (int i = 0; i < Objects.requireNonNull(files).length; i++) {
if (files[i].equals(xmindZenJson)) {
return true;
}
}
}
return false;
}
public static void main(String[] args) throws IOException, ArchiveException, DocumentException, JSONException {
// String fileName = "/Users/lijianxi/Desktop/【租房价格】价格助手权限优化.xmind";
String fileName2 = "/Users/lijianxi/Desktop/租房AI生成标题描述.xmind";
String res = XmindParser.parseJson(fileName2);
JsonRootBean jsonRootBean = JSON.parseObject(res, JsonRootBean.class);
System.out.println(JSON.toJSONString(jsonRootBean, true));
}
}
XmindZen.java
package com.bj58.fangcase.fangcasemanage.util.xmind;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import org.dom4j.DocumentException;
import java.io.IOException;
/**
* @author liufree liufreeo@gmail.com
* @Classname XmindZen
* @Description TODO
* @Date 2020/4/28 10:26
*/
public class XmindZen {
/**
* "notes": {
* "plain": {
* "content": "这是副节点1的备注"
* },
* "ops": {
* "ops": [
* {
* "insert": "这是副节点1的备注\n"
* }
* ]
* }
* 转换为
* "notes":{
* "content":"这是副节点1的备注"
* }
*
* @param jsonContent
* @return
* @throws IOException
* @throws DocumentException
*/
public static String getContent(String jsonContent) {
JSONObject jsonObject = JSONArray.parseArray(jsonContent).getJSONObject(0);
JSONObject rootTopic = jsonObject.getJSONObject("rootTopic");
transferNotes(rootTopic);
JSONObject children = rootTopic.getJSONObject("children");
recursionChildren(children);
return jsonObject.toString();
}
/**
* 递归转换children
*
* @param children
*/
private static void recursionChildren(JSONObject children) {
if (children == null) {
return;
}
JSONArray attachedArray = children.getJSONArray("attached");
if (attachedArray == null) {
return;
}
for (Object attached : attachedArray) {
JSONObject attachedObject = (JSONObject) attached;
transferNotes(attachedObject);
JSONObject childrenObject = attachedObject.getJSONObject("children");
if (childrenObject == null) {
continue;
}
recursionChildren(childrenObject);
}
}
private static void transferNotes(JSONObject object) {
JSONObject notes = object.getJSONObject("notes");
if (notes == null) {
return;
}
JSONObject plain = notes.getJSONObject("plain");
if (plain != null) {
String content = plain.getString("content");
notes.remove("plain");
notes.put("content", content);
} else {
notes.put("content", null);
}
}
}
ZipUtils.java
package com.bj58.fangcase.fangcasemanage.util.xmind;
import lombok.Value;
import org.apache.commons.compress.archivers.ArchiveException;
import org.apache.commons.compress.archivers.examples.Expander;
import lombok.extern.slf4j.Slf4j;
import java.io.*;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* @author liufree
* @Classname ZipUtil
* @Description zip解压工具
* @Date 2020/4/14 15:39
*/
@Slf4j
public class ZipUtils {
private static String currentPath="/opt/case_management/Xmind";
// private static String currentPath=System.getProperty("user.dir");
/**
* 找到压缩文件中匹配的子文件,返回的为
* getContents("comments.xml,
* unzip
* @param subFileNames
* @param fileName
*/
public static Map<String,String> getContents(List<String> subFileNames, String fileName,String extractFileDir) throws IOException, ArchiveException {
String destFilePath =extractFileDir;
Map<String,String> map = new HashMap<>();
File destFile = new File(destFilePath);
if (destFile.isDirectory()) {
String[] res = destFile.list(new FileFilter());
for (int i = 0; i < Objects.requireNonNull(res).length; i++) {
if (subFileNames.contains(res[i])) {
String s = destFilePath + File.separator + res[i];
String content = getFileContent(s);
map.put(res[i], content);
}
}
}
return map;
}
/**
* 返回解压后的文件夹名字
* @param fileName
* @return
* @throws IOException
* @throws ArchiveException
*/
public static String extract(String fileName) throws IOException, ArchiveException {
File file = new File(fileName);
Expander expander = new Expander();
//目标文件夹名字
String destFileName =currentPath +File.separator+ "XMind"+System.currentTimeMillis();
File folder = new File(destFileName);
boolean mkdir = folder.mkdirs();
log.info("destFileName"+destFileName);
log.info("创建文件夹失败了吗"+mkdir);
if(mkdir){
expander.expand(file,folder );
}
return destFileName;
}
//这是一个内部类过滤器,策略模式
static class FileFilter implements FilenameFilter {
@Override
public boolean accept(File dir, String name) {
//String的 endsWith(String str)方法 筛选出以str结尾的字符串
if (name.endsWith(".xml") || name.endsWith(".json")) {
return true;
}
return false;
}
}
public static String getFileContent(String fileName) throws IOException {
File file;
try {
file = new File(fileName);
} catch (Exception e) {
throw new RuntimeException("找不到该文件");
}
FileReader fileReader = new FileReader(file);
BufferedReader bufferedReder = new BufferedReader(fileReader);
StringBuilder stringBuffer = new StringBuilder();
while (bufferedReder.ready()) {
stringBuffer.append(bufferedReder.readLine());
}
//打开的文件需关闭,在unix下可以删除,否则在windows下不能删除(file.delete())
bufferedReder.close();
fileReader.close();
return stringBuffer.toString();
}
/*public static void main(String[] args) throws IOException, ArchiveException {
String fileName = "doc/XmindZen解析.xmind";
List<String> list= new ArrayList<>();
// list.add("comments.xml");
list.add("content.json");
System.out.println(File.separator);
// System.out.println(getContents(list, fileName));
}
*/
public static void main(String[] args) throws IOException {
String filepath = currentPath + File.separator + "XMind" + System.currentTimeMillis();
System.out.println("filepath = " + filepath);
File file = new File(filepath);
boolean newFile = file.mkdir();
System.out.println("newFile = " + newFile);
System.out.println("file.isDirectory() = " + file.isDirectory());
System.out.println("file.exists() = " + file.exists());
}
}
/**
* Copyright 2020 bejson.com
*/
package com.bj58.fangcase.fangcasemanage.util.xmind.pojo;
import lombok.Data;
import java.util.List;
/**
* Auto-generated: 2020-03-24 11:24:27
*
* @author bejson.com (i@bejson.com)
* @website http://www.bejson.com/java2pojo/
*/
@Data
public class Attached {
private String id;
private String title;
private Notes notes;
private List<Attached> expectedResultList; // 如果节点是步骤,则该节点为预期结果列表
private Children children;
private MarkerRefs markerrefs;
private List<MarkerId> markers;
private Image image;
}
各个bean包
/**
* Copyright 2020 bejson.com
*/
package com.bj58.fangcase.fangcasemanage.util.xmind.pojo;
import lombok.Data;
import java.util.List;
/**
* Auto-generated: 2020-03-24 11:24:27
*
* @author bejson.com (i@bejson.com)
* @website http://www.bejson.com/java2pojo/
*/
@Data
public class Children {
private List<Attached> attached;
}
/**
* Copyright 2020 bejson.com
*/
package com.bj58.fangcase.fangcasemanage.util.xmind.pojo;
import lombok.Data;
/**
* Auto-generated: 2020-03-24 11:24:27
*
* @author bejson.com (i@bejson.com)
* @website http://www.bejson.com/java2pojo/
*/
@Data
public class Comments {
private long creationTime;
private String author;
private String content;
}
package com.bj58.fangcase.fangcasemanage.util.xmind.pojo;
import lombok.Data;
@Data
public class Image {
public String src;
}
/**
* Copyright 2020 bejson.com
*/
package com.bj58.fangcase.fangcasemanage.util.xmind.pojo;
import lombok.Data;
/**
* Auto-generated: 2020-03-24 11:24:27
*
* @author bejson.com (i@bejson.com)
* @website http://www.bejson.com/java2pojo/
*/
@Data
public class JsonRootBean {
private String id;
private String title;
private RootTopic rootTopic; // 暂时替换为Attached
}
package com.bj58.fangcase.fangcasemanage.util.xmind.pojo;
import lombok.Data;
@Data
public class MarkerId {
private String markerId;
}
package com.bj58.fangcase.fangcasemanage.util.xmind.pojo;
import lombok.Data;
import java.util.List;
import java.util.Map;
@Data
public class MarkerRefs {
private List<Map<String, String>> markerref;
}
/**
* Copyright 2020 bejson.com
*/
package com.bj58.fangcase.fangcasemanage.util.xmind.pojo;
import lombok.Data;
/**
* Auto-generated: 2020-03-24 11:24:27
*
* @author bejson.com (i@bejson.com)
* @website http://www.bejson.com/java2pojo/
*/
@Data
public class Notes {
private String content;
}
/**
* Copyright 2020 bejson.com
*/
package com.bj58.fangcase.fangcasemanage.util.xmind.pojo;
import lombok.Data;
/**
* Auto-generated: 2020-03-24 11:24:27
*
* @author bejson.com (i@bejson.com)
* @website http://www.bejson.com/java2pojo/
*/
@Data
public class RootTopic extends Attached{
}
package com.bj58.fangcase.fangcasemanage.util.xmind.enums;
public enum MarkerEnum {
MOKUAI("star", "模块"),
YUQIJIEGUO("flag", "预期结果"),
YOUXIANJI("priority", "优先级"),
QIANZHITIAOJIAN("people", "前置条件"),
BUZHOU("arrow", "步骤");
private String tubiao;
private String caseType;
MarkerEnum(String tubiao, String caseType) {
this.tubiao = tubiao;
this.caseType = caseType;
}
}
public static void getAllXmindCaseIter(List<List<Attached>> arrayListList,
List<Attached> parentAttachedList, List<Attached> childAttachedList) {
//xmind一条用例节点数不能超过10个
if (parentAttachedList.size() >= 10) {
List<Attached> attachedListOneCompleteCase = new ArrayList<>();
CollectionUtils.addAll(attachedListOneCompleteCase, new Object[parentAttachedList.size()]);
Collections.copy(attachedListOneCompleteCase, parentAttachedList);
arrayListList.add(attachedListOneCompleteCase);
return;
}
int cnt = 0;
for (Attached attachedChild : childAttachedList) {
if (attachedChild.getTitle() != null) {
if (attachedChild.getTitle().startsWith("#")) {
continue;
}
}
++cnt;
parentAttachedList.add(attachedChild);
if (attachedChild.getChildren() == null) {
List<Attached> attachedListOneCompleteCase = new ArrayList<>();
CollectionUtils.addAll(attachedListOneCompleteCase, new Object[parentAttachedList.size()]);
Collections.copy(attachedListOneCompleteCase, parentAttachedList);
arrayListList.add(attachedListOneCompleteCase);
} else {
getAllXmindCaseIter(arrayListList, parentAttachedList, attachedChild.getChildren().getAttached());
}
parentAttachedList.remove(parentAttachedList.size() - 1);
}
if (cnt == 0) {
List<Attached> attachedListOneCompleteCase = new ArrayList<>();
CollectionUtils.addAll(attachedListOneCompleteCase, new Object[parentAttachedList.size()]);
Collections.copy(attachedListOneCompleteCase, parentAttachedList);
arrayListList.add(attachedListOneCompleteCase);
}
}
// 获取xmind的所有用例
public static List<EsCaseEntity> getAllCaseFromXmindFile(String fileName) {
List<EsCaseEntity> casesList = new ArrayList<>();
if (Objects.isNull(fileName)) {
return null;
}
File fileXmind = new File(fileName);
if (!fileXmind.isFile()) {
System.out.println("不是文件");
return null;
}
try {
String res = XmindParser.parseJson(fileName);
JsonRootBean jsonRootBean = JSON.parseObject(res, JsonRootBean.class);
System.out.println("JSON.toJSONString(jsonRootBean) = " + JSON.toJSONString(jsonRootBean));
// 暂时替换为attached
Attached attachedRootTopic = (Attached) jsonRootBean.getRootTopic();
if (Objects.isNull(attachedRootTopic)) {
return null;
}
// 开始获取数据
List<List<Attached>> attachedListListCase = new ArrayList<>();
getAllXmindCase(attachedListListCase, attachedRootTopic);
System.out.println("attachedListListCase.sie = " + attachedListListCase.size());
for (List<Attached> oneAttachedList : attachedListListCase) {
casesList.add(getCaseFromAttachedList(oneAttachedList));
}
return casesList;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
// 通过attached列表获取一个case
public static EsCaseEntity getCaseFromAttachedList(List<Attached> attachedList) {
if (Objects.isNull(attachedList) || attachedList.isEmpty()) {
return null;
}
EsCaseEntity oneCase = new EsCaseEntity();
String title = attachedList.get(0).getTitle();
String url = "";
if (attachedList.size() > 1) {
//设置标题
title = attachedList.get(1).getTitle();
}
if (attachedList.size() > 2) {
//新版xmind标记通过markerIdList来
List<MarkerId> markerIdList = attachedList.get(2).getMarkers();
//旧版xmind标记通过MarkerRefs来 与markerIdList互斥
MarkerRefs markerRefs = attachedList.get(2).getMarkerrefs();
int smokeBelong = 0;
int isSmoke=0;
if (markerIdList != null && markerRefs == null) {
for (MarkerId markerId : markerIdList) {
if (markerId.getMarkerId().equals("starblue")) {
//后端用例
isSmoke=1;
smokeBelong= 2;
} else if (markerId.getMarkerId().equals("starred")) {
//前端用例
smokeBelong = 1;
isSmoke=1;
}
}
}
if (markerRefs != null) {
List<Map<String, String>> markerrefList = markerRefs.getMarkerref();
if (markerrefList != null) {
for (Map<String, String> markerref : markerrefList) {
if (markerref.get("markerid").equals("starblue")) {
//后端用例
smokeBelong = 2;
} else if (markerref.get("markerid").equals("starred")) {
//前端用例
smokeBelong = 1;
}
}
}
}
oneCase.setIsSmoke(isSmoke);
oneCase.setSmokeBelong(smokeBelong);
if(oneCase.getContent()==null){
oneCase.setContent(new HashMap<Integer, String>());
}
oneCase.getContent().put(1, attachedList.get(2).getTitle());
}
//设置caseId
oneCase.setCaseId(attachedList.get(attachedList.size()-1).getId());
oneCase.setCaseDes(title);
if (attachedList.size() > 3) {
for (int i = 3; i < attachedList.size(); i++) {
oneCase.getContent().put(i-1, attachedList.get(i).getTitle());
}
}
//设置结果
if(oneCase.getExt()==null){
oneCase.setExt(new HashMap<String, String>());
}
oneCase.getExt().put("caseResult", CaseResultStatusEnums.WAIT_EXECUTE.val+"");
parseImage(attachedList);
System.out.println("oneCase = " + oneCase);
return oneCase;
}
@SneakyThrows
public static void parseImage(List<Attached> attachedList){
String destFileName = XmindParser.filePath;
String filePath = "";
for (int i = 0; i < attachedList.size(); i++) {
Image image = attachedList.get(i).getImage();
if(image!=null){
if(image.getSrc()!=null){
if(destFileName!=null){
String imgTmpPath = image.getSrc().substring(4);
filePath = destFileName + File.separator + imgTmpPath;
}
System.out.println("图片位置 = " + filePath);
}
}
}
}
// 获取一个根节点的所有用例
public static void getAllXmindCase(List<List<Attached>> attachedListList, Attached attachedRootTopic) {
List<Attached> attachedList = new ArrayList<>();
attachedList.add(attachedRootTopic);
getAllXmindCaseIter(attachedListList, attachedList,
attachedList.get(attachedList.size() - 1).getChildren().getAttached());
}
public static void getAllXmindCaseIter(List<List<Attached>> arrayListList,
List<Attached> parentAttachedList, List<Attached> childAttachedList) {
//xmind一条用例节点数不能超过10个
if (parentAttachedList.size() >= 10) {
List<Attached> attachedListOneCompleteCase = new ArrayList<>();
CollectionUtils.addAll(attachedListOneCompleteCase, new Object[parentAttachedList.size()]);
Collections.copy(attachedListOneCompleteCase, parentAttachedList);
arrayListList.add(attachedListOneCompleteCase);
return;
}
int cnt = 0;
for (Attached attachedChild : childAttachedList) {
if(attachedChild.getTitle()!=null) {
if (attachedChild.getTitle().startsWith("#")) {
continue;
}
}
++cnt;
parentAttachedList.add(attachedChild);
if (attachedChild.getChildren() == null) {
List<Attached> attachedListOneCompleteCase = new ArrayList<>();
CollectionUtils.addAll(attachedListOneCompleteCase, new Object[parentAttachedList.size()]);
Collections.copy(attachedListOneCompleteCase, parentAttachedList);
arrayListList.add(attachedListOneCompleteCase);
} else {
getAllXmindCaseIter(arrayListList, parentAttachedList, attachedChild.getChildren().getAttached());
}
parentAttachedList.remove(parentAttachedList.size() - 1);
}
if (cnt == 0) {
List<Attached> attachedListOneCompleteCase = new ArrayList<>();
CollectionUtils.addAll(attachedListOneCompleteCase, new Object[parentAttachedList.size()]);
Collections.copy(attachedListOneCompleteCase, parentAttachedList);
arrayListList.add(attachedListOneCompleteCase);
}
}
3、主要方法
String fileName = "/Users/xxx/Desktop/xxx.xmind";
//解析文件为字符串
String res = XmindParser.parseJson(fileName);
//字符串转换为JaonRootBean
JsonRootBean jsonRootBean = JSON.parseObject(res, JsonRootBean.class);
//获取xmin从第一个节点到最后一个节点的List
Attached attachedRootTopic = (Attached) jsonRootBean.getRootTopic();
List<List<Attached>> attachedListListCase = new ArrayList<>();
getAllXmindCase(attachedListListCase, attachedRootTopic);
for (List<Attached> attacheds : attachedListListCase) {
log.info(attacheds.get(attacheds.size()-1).toString());
}
4、思路
主要是将xmind文件解析为一个字符串后,然后将字符串转换为一个JsonRootBean,然后可以获取xmind每一个节点的数据,根据每一个节点的数据,可以进行自己的定制化使用
更多推荐
所有评论(0)