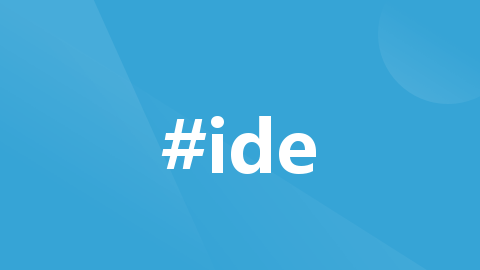
idea代码java语法高亮显示方案设置
方法中,我们使用正则表达式来匹配Java代码中的不同部分(关键字、括号、字符串、注释等),并给它们应用不同的样式。类来实现代码高亮显示。在上述代码中,我们使用了JavaFX提供的。在Java中,可以使用JavaFX的。为了使代码高亮显示生效,我们需要将。作为根节点创建JavaFX场景。组件作为代码编辑器,并使用。类来设置代码高亮的样式。
·
在Java中,可以使用JavaFX的SyntaxHighlighter
类来实现代码高亮显示。以下是一个简单的例子,演示如何使用JavaFX来设置代码高亮显示方案:
import javafx.scene.control.TextArea;
import javafx.scene.layout.StackPane;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.scene.text.TextFlow;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ScrollPane;
import org.fxmisc.richtext.CodeArea;
import org.fxmisc.richtext.LineNumberFactory;
import org.fxmisc.richtext.model.StyleSpans;
import org.fxmisc.richtext.model.StyleSpansBuilder;
import org.reactfx.Subscription;
import java.time.Duration;
import java.util.Collection;
import java.util.Collections;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class SyntaxHighlighting extends Application {
private static final String[] KEYWORDS = new String[]{
"abstract", "assert", "boolean", "break", "byte",
"case", "catch", "char", "class", "const",
"continue", "default", "do", "double", "else",
"enum", "extends", "final", "finally", "float",
"for", "goto", "if", "implements", "import",
"instanceof", "int", "interface", "long", "native",
"new", "package", "private", "protected", "public",
"return", "short", "static", "strictfp", "super",
"switch", "synchronized", "this", "throw", "throws",
"transient", "try", "void", "volatile", "while"
};
private static final String KEYWORD_PATTERN = "\\b(" + String.join("|", KEYWORDS) + ")\\b";
private static final String PAREN_PATTERN = "\\(|\\)";
private static final String BRACE_PATTERN = "\\{|\\}";
private static final String BRACKET_PATTERN = "\\[|\\]";
private static final String SEMICOLON_PATTERN = "\\;";
private static final String STRING_PATTERN = "\"([^\"\\\\]|\\\\.)*\"";
private static final String COMMENT_PATTERN = "//[^\n]*" + "|" + "/\\*(.|\\R)*?\\*/";
private static final Pattern PATTERN = Pattern.compile(
"(?<KEYWORD>" + KEYWORD_PATTERN + ")"
+ "|(?<PAREN>" + PAREN_PATTERN + ")"
+ "|(?<BRACE>" + BRACE_PATTERN + ")"
+ "|(?<BRACKET>" + BRACKET_PATTERN + ")"
+ "|(?<SEMICOLON>" + SEMICOLON_PATTERN + ")"
+ "|(?<STRING>" + STRING_PATTERN + ")"
+ "|(?<COMMENT>" + COMMENT_PATTERN + ")"
);
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage stage) {
// Create a JavaFX TextArea
TextArea textArea = new TextArea();
textArea.setWrapText(true);
textArea.setFont(Font.font("Courier New", 14));
// Set up syntax highlighting
Subscription cleanupWhenNoLongerNeedIt = setupSyntaxHighlighting(textArea);
// Add the TextArea to a ScrollPane
ScrollPane scrollPane = new ScrollPane(textArea);
scrollPane.setFitToHeight(true);
scrollPane.setFitToWidth(true);
// Create a JavaFX StackPane to hold the ScrollPane
StackPane stackPane = new StackPane(scrollPane);
// Create a JavaFX Scene with the StackPane as the root node
Scene scene = new Scene(stackPane, 800, 600);
// Set the Scene to the Stage
stage.setScene(scene);
// Show the Stage
stage.show();
// Clean up the syntax highlighting when the Stage is closed
stage.setOnCloseRequest(event -> cleanupWhenNoLongerNeedIt.unsubscribe());
}
private static Subscription setupSyntaxHighlighting(TextArea textArea) {
CodeArea codeArea = new CodeArea();
codeArea.textProperty().bindBidirectional(textArea.textProperty());
codeArea.setParagraphGraphicFactory(LineNumberFactory.get(codeArea));
codeArea.setStyleSpansFactory(this::computeHighlighting);
Subscription cleanupWhenNoLongerNeedIt = codeArea
.multiPlainChanges()
.successionEnds(Duration.ofMillis(500))
.subscribe(ignore -> codeArea.setStyleSpans(0, computeHighlighting(codeArea.getText())));
return cleanupWhenNoLongerNeedIt;
}
private static StyleSpans<Collection<String>> computeHighlighting(String text) {
Matcher matcher = PATTERN.matcher(text);
int lastKwEnd = 0;
StyleSpansBuilder<Collection<String>> spansBuilder = new StyleSpansBuilder<>();
while (matcher.find()) {
String styleClass =
matcher.group("KEYWORD") != null ? "keyword" :
matcher.group("PAREN") != null ? "paren" :
matcher.group("BRACE") != null ? "brace" :
matcher.group("BRACKET") != null ? "bracket" :
matcher.group("SEMICOLON") != null ? "semicolon" :
matcher.group("STRING") != null ? "string" :
matcher.group("COMMENT") != null ? "comment" :
null;
assert styleClass != null;
spansBuilder.add(Collections.emptyList(), matcher.start() - lastKwEnd);
spansBuilder.add(Collections.singleton(styleClass), matcher.end() - matcher.start());
lastKwEnd = matcher.end();
}
spansBuilder.add(Collections.emptyList(), text.length() - lastKwEnd);
return spansBuilder.create();
}
}
在上述代码中,我们使用了JavaFX提供的CodeArea
组件作为代码编辑器,并使用StyleSpans
类来设置代码高亮的样式。
在computeHighlighting
方法中,我们使用正则表达式来匹配Java代码中的不同部分(关键字、括号、字符串、注释等),并给它们应用不同的样式。
为了使代码高亮显示生效,我们需要将TextArea
组件绑定到CodeArea
组件,并在CodeArea
的multiPlainChanges
方法中设置样式。
最后,将TextArea
组件放入一个ScrollPane
中,并将ScrollPane
放入一个StackPane
中,最后将StackPane
作为根节点创建JavaFX场景。
更多推荐
所有评论(0)