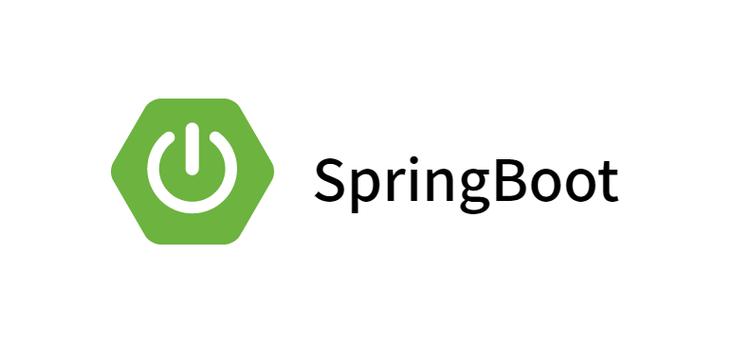
springboot基础学习 之数据库连接与配置
public interface BookRepository extends JpaRepository { // 自动提供了基本的CRUD操作 }通过定义实体类和JpaRepository接口,你可以轻松地进行数据库操作。Spring Boot将自动配置数据源和JPA相关的Bean,你只需注入并使用它即可。这些是关于数据库连接与配置的基本信息。在实践中,你可以根据具体的业务需求调整配置,使用不
在Spring Boot中,配置数据库连接是常见的任务之一。Spring Boot通过提供简单的配置方式和自动配置功能,使得数据库连接的配置变得相对简单。下面是一些关于数据库连接与配置的基本信息:
1. 配置数据源
Spring Boot支持多种数据源,包括嵌入式数据库(如H2)、关系型数据库(如MySQL、PostgreSQL)等。在application.properties
或application.yml
中配置数据源的相关属性。
示例 - MySQL 数据库配置:
propertiesCopy code
# 数据库连接配置 spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase spring.datasource.username=myuser spring.datasource.password=mypassword # 数据源驱动程序类名 spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver # Hibernate配置 spring.jpa.hibernate.ddl-auto=update spring.jpa.show-sql=true
在这个例子中:
spring.datasource.url
:指定数据库连接URL。spring.datasource.username
和spring.datasource.password
:数据库的用户名和密码。spring.datasource.driver-class-name
:指定数据库驱动程序类名,对于MySQL,使用com.mysql.cj.jdbc.Driver
。spring.jpa.hibernate.ddl-auto
:Hibernate的DDL操作。在开发阶段,可以设置为update
,使Hibernate在启动时更新数据库结构。spring.jpa.show-sql
:是否打印SQL语句,便于调试。
示例 - H2 嵌入式数据库配置:
如果你想使用内存中的嵌入式数据库(如H2),配置如下:
propertiesCopy code
# H2数据库连接配置 spring.datasource.url=jdbc:h2:mem:testdb spring.datasource.driver-class-name=org.h2.Driver spring.datasource.username=sa spring.datasource.password=password # Hibernate配置 spring.jpa.hibernate.ddl-auto=update spring.jpa.show-sql=true
在这个例子中,jdbc:h2:mem:testdb
表示使用内存中的H2数据库。
2. 多数据源配置
如果你需要连接多个数据库,Spring Boot也提供了多数据源的支持。你可以通过配置多个DataSource
和EntityManager
来实现。
示例 - 多数据源配置:
propertiesCopy code
# 主数据源配置 spring.datasource.primary.url=jdbc:mysql://localhost:3306/primarydb spring.datasource.primary.username=primaryuser spring.datasource.primary.password=primarypassword spring.datasource.primary.driver-class-name=com.mysql.cj.jdbc.Driver # 第二个数据源配置 spring.datasource.secondary.url=jdbc:mysql://localhost:3306/secondarydb spring.datasource.secondary.username=secondaryuser spring.datasource.secondary.password=secondarypassword spring.datasource.secondary.driver-class-name=com.mysql.cj.jdbc.Driver
3. 使用 JPA 进行数据库操作
Spring Boot通常与JPA(Java Persistence API)一起使用,简化了数据库操作的过程。只需定义实体类和JpaRepository接口,Spring Boot将自动生成数据库表和提供基本的CRUD操作。
示例 - 定义实体类:
javaCopy code
import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class Book { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String title; private String author; // getters and setters }
示例 - 定义 JpaRepository 接口:
javaCopy code
import org.springframework.data.jpa.repository.JpaRepository; public interface BookRepository extends JpaRepository<Book, Long> { // 自动提供了基本的CRUD操作 }
通过定义实体类和JpaRepository接口,你可以轻松地进行数据库操作。Spring Boot将自动配置数据源和JPA相关的Bean,你只需注入BookRepository
并使用它即可。
这些是关于数据库连接与配置的基本信息。在实践中,你可以根据具体的业务需求调整配置,使用不同的数据库,或者配置多数据源。Spring Boot的自动配置和便利功能使得数据库连接和操作变得相对简单。
更多推荐
所有评论(0)