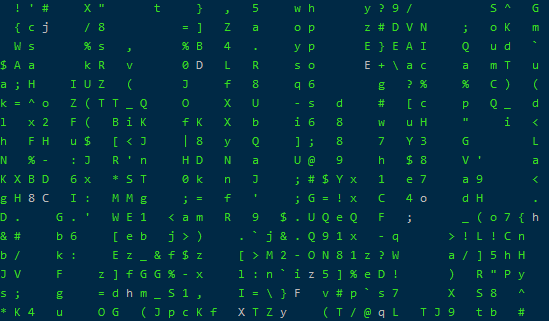
java实现Linux服务器下载文件到windows指定目录
废话不多说。来吧,展示!1,首先找到Linux服务器上想要下载的文件这里我想要下载一个文件名为myqxin.jpg的图片,文件路径为/home/Fileimg/myqxin.jpg2,windows上想要保存文件的位置(自定义)3,执行以下测试代码package com.czxy.music.web.test.day02;import ch.ethz.ssh2.Connection;import
·
📫 作者简介:「子非我鱼」,专注于研究全栈
🔥 三连支持:欢迎 ❤️关注、👍点赞、👉收藏三连,支持一下博主~
文章目录
文件下载
步骤一:首先找到Linux服务器上想要下载的文件
这里我想要下载一个文件名为鬼刀.png的图片,文件路径为/home/myqxin/鬼刀.png
步骤二:windows上想要保存文件的位置(自定义)
步骤三:执行以下测试代码
package com.czxy.music.web.test.day02;
import ch.ethz.ssh2.Connection;
import com.czxy.music.utils.RemoteCommandUtil;
/**
* Created by zfwy on 2020/9/25.
*/
public class Myqxin {
public static void main(String[] args)throws Exception {
// 创建服务对象
RemoteCommandUtil commandUtil = new RemoteCommandUtil();
// 登录到LInux服务
Connection root = commandUtil.login("服务器ip", "用户名", "密码");
// 服务器文件路径
String fwPath = "/home/myqxin";
// windows本地存放目录,不需要加上具体文件
String bdPath = "D:\\Music\\";
// 下载文件
commandUtil.downloadFile(root,"鬼刀.png",fwPath,bdPath,null);
// 批量下载
// commandUtil.downloadDirFile(root,fwPath,bdPath);
}
}
服务器ip:是你LInux访问的ip地址,例如填写(172.18.0.2)
服务用户名:是你Linux用户名,一般为(root)用户
服务密码:是你Linux用户的密码,聪明的你不会忘记
执行效果如下:
步骤四:通过流进行下载,此方式不用考虑项目部署
@GetMapping("/downimage")
public void downimage(HttpServletResponse response) throws Exception {
// 创建服务对象
RemoteCommandUtil commandUtil = new RemoteCommandUtil();
// 登录到LInux服务
Connection root = commandUtil.login("192.168.124.156", "root", "root");
// 服务器文件路径
String fwPath = "/home/data/images";
commandUtil.downloadFileFlow(root,"sun.jpeg",fwPath,response);
}
文件上传
步骤一:自定义想要上传到服务器的地址
步骤二:找到想要上传的文件
刚才我从服务器上下载了鬼刀.png的图片,现在我想要sun.jpeg的文件上传到服务器
步骤三:执行以下测试代码
public class Myqxin {
public static void main(String[] args)throws Exception {
// 创建服务对象
RemoteCommandUtil commandUtil = new RemoteCommandUtil();
// 登录到LInux服务
Connection root = commandUtil.login("服务器ip", "用户名", "密码");
// 服务器文件路径
String fwPath = "/home/myqxin";
// 上传文件
File file = new File("D:\\Music\\sun.jpeg");
// 如果存在
// if(file.exists()){
// 文件重命名
// String newname = "新名称"+".jpeg";
// File newFile = new File(file.getParent() + File.separator + newname);
// if (file.renameTo(newFile))
// commandUtil.uploadFile(root,newFile,fwPath,null);
// }
commandUtil.uploadFile(root,file,fwPath,null);
}
}
执行效果如下:
下面是RemoteCommandUtil.java的源码
package com.myqxin.common.utils;
import ch.ethz.ssh2.*;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.BeanUtils;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
/**
* @author zfwy
*/
@Slf4j
public class RemoteCommandUtil {
//目标服务器端口,默认
private static int port = 22;
private static Connection conn = null;
/**
* 登录服务器主机
*
* @param ip 主机IP
* @param userName 用户名
* @param userPwd 密码
* @return 登录对象
*/
public static Connection login(String ip, String userName, String userPwd) {
boolean flg = false;
Connection conn = null;
try {
conn = new Connection(ip, port);
//连接
conn.connect();
//认证
flg = conn.authenticateWithPassword(userName, userPwd);
if (flg) {
log.info("连接成功");
return conn;
}
} catch (IOException e) {
log.error("连接失败" + e.getMessage());
e.printStackTrace();
}
return conn;
}
/**
* 通过流下载到本地
*
* @param conn SSH连接信息
* @param fileName 要下载的具体文件名称
* @param basePath 服务器上的文件地址/home/img
* @throws IOException
*/
public void downloadFileFlow(Connection conn, String fileName, String basePath, HttpServletResponse response) throws IOException {
SCPClient scpClient = conn.createSCPClient();
try {
SCPInputStream sis = scpClient.get(basePath + "/" + fileName);
SCPInputStream two = scpClient.get(basePath + "/" + fileName);;
long file_length = getFileSizeByByteArrayOutputStream(two);
// 以流的形式下载文件。
byte[] buffer = new byte[(int) file_length];
sis.read(buffer);
sis.close();
// 清空response
response.reset();
// 设置response的Header
response.addHeader("Content-Disposition", "attachment;filename=" + new String(fileName.getBytes()));
response.addHeader("Content-Length", "" + file_length);
OutputStream toClient = new BufferedOutputStream(response.getOutputStream());
response.setContentType("application/octet-stream");
toClient.write(buffer);
toClient.flush();
toClient.close();
} catch (IOException e) {
log.info("文件不存在或连接失败");
e.printStackTrace();
} finally {
log.info("服务关闭");
closeConn();
}
}
public static long getFileSizeByByteArrayOutputStream(InputStream inputStream) {
ByteArrayOutputStream bos = null;
try {
bos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = inputStream.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
return bos.size();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (bos != null) {
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return -1;
}
/**
* 实现下载服务器上的文件到本地指定目录
*
* @param conn SSH连接信息
* @param fileName 要下载的具体文件名称
* @param basePath 服务器上的文件地址/home/img
* @param localPath 本地路径:D:\
* @param newName 文件重命名
* @throws IOException
*/
public void downloadFile(Connection conn, String fileName, String basePath, String localPath, String newName) throws IOException {
SCPClient scpClient = conn.createSCPClient();
try {
SCPInputStream sis = scpClient.get(basePath + "/" + fileName);
File f = new File(localPath);
if (!f.exists()) {
f.mkdirs();
}
File newFile = null;
if (StringUtils.isBlank(newName)) {
newFile = new File(localPath + fileName);
} else {
newFile = new File(localPath + newName);
}
FileOutputStream fos = new FileOutputStream(newFile);
byte[] b = new byte[4096];
int i;
while ((i = sis.read(b)) != -1) {
fos.write(b, 0, i);
}
fos.flush();
fos.close();
sis.close();
log.info("下载完成");
} catch (IOException e) {
log.info("文件不存在或连接失败");
e.printStackTrace();
} finally {
log.info("服务关闭");
closeConn();
}
}
/**
* 上传文件到服务器
*
* @param conn SSH连接信息
* @param f 文件对象
* @param remoteTargetDirectory 上传路径
* @param mode 默认为null
*/
public void uploadFile(Connection conn, File f, String remoteTargetDirectory, String mode) {
try {
SCPClient scpClient = new SCPClient(conn);
SCPOutputStream os = scpClient.put(f.getName(), f.length(), remoteTargetDirectory, mode);
byte[] b = new byte[4096];
FileInputStream fis = new FileInputStream(f);
int i;
while ((i = fis.read(b)) != -1) {
os.write(b, 0, i);
}
os.flush();
fis.close();
os.close();
log.info("上传成功");
} catch (IOException e) {
e.printStackTrace();
} finally {
closeConn();
}
}
/**
* 关闭服务
*/
public static void closeConn() {
if (null == conn) {
return;
}
conn.close();
}
/**
* 批量下载目录所有文件
*
* @param root SSH连接信息
* @param fwPath 服务器上的文件地址/home/img
* @param bdPath 本地路径:D:\
*/
public void downloadDirFile(Connection root, String fwPath, String bdPath) throws IOException {
SCPClient scpClient = root.createSCPClient();
try {
List<String> allFilePath = getAllFilePath(root, fwPath);
File f = new File(bdPath);
if (!f.exists()) {
f.mkdirs();
}
FileOutputStream fos = null;
SCPInputStream sis = null;
for (String path : allFilePath) {
String[] split = path.split("/");
String filename = split[split.length - 1];
sis = scpClient.get(path);
File newFile = new File(bdPath);
fos = new FileOutputStream(newFile + "\\" + filename);
byte[] b = new byte[4096];
int i;
while ((i = sis.read(b)) != -1) {
fos.write(b, 0, i);
}
}
fos.flush();
fos.close();
sis.close();
log.info("下载完成");
} catch (IOException e) {
log.info("文件不存在或连接失败");
e.printStackTrace();
} finally {
log.info("服务关闭");
closeConn();
}
}
/**
* 获取目录下所有文件路径
*
* @param conn
* @param fwPath
* @return
*/
public List<String> getAllFilePath(Connection conn, String fwPath) {
List<String> folderNameList = new ArrayList<String>();
try {
// 创建一个会话
Session sess = conn.openSession();
sess.requestPTY("bash");
sess.startShell();
StreamGobbler stdout = new StreamGobbler(sess.getStdout());
StreamGobbler stderr = new StreamGobbler(sess.getStderr());
BufferedReader stdoutReader = new BufferedReader(new InputStreamReader(stdout));
BufferedReader stderrReader = new BufferedReader(new InputStreamReader(stdout));
PrintWriter out = new PrintWriter(sess.getStdin());
out.println("cd " + fwPath);
out.println("ll");
out.println("exit");
out.close();
sess.waitForCondition(ChannelCondition.CLOSED | ChannelCondition.EOF | ChannelCondition.EXIT_STATUS, 30000);
while (true) {
String line = stdoutReader.readLine();
if (line == null)
break;
//获取文件名称
if (line.contains("-rw-r--r--")) {
//取出正确的文件名称
StringBuffer sb =
new StringBuffer(line.substring(line.lastIndexOf(":") + 3, line.length()));
line = sb.toString().replace(" ", fwPath + "/");
folderNameList.add(line);
}
}
//关闭连接
sess.close();
stderrReader.close();
stdoutReader.close();
} catch (IOException e) {
log.info("文件不存在或连接失败");
System.exit(2);
e.printStackTrace();
} finally {
log.info("服务关闭");
closeConn();
}
return folderNameList;
}
}
需要往你的pom.xml导入SSH依赖
<!-- SSH2 -->
<dependency>
<groupId>ch.ethz.ganymed</groupId>
<artifactId>ganymed-ssh2</artifactId>
<version>262</version>
</dependency>
更多推荐
所有评论(0)