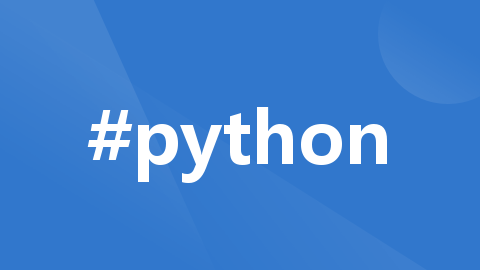
python 线程只能start一次,如何解决?
在Python中,如果你尝试对一个已经启动的线程(Thread对象)再次调用.start()方法,会抛出异常,提示“thread already started”。这是因为Python的线程模型不允许一个线程被启动多次。每个线程实例只能执行一次其run()方法,而.start()方法用于启动线程,实际上是调用该线程的run()方法。
·
在Python中,如果你尝试对一个已经启动的线程(Thread对象)再次调用.start()
方法,会抛出RuntimeError
异常,提示“thread already started”。这是因为Python的线程模型不允许一个线程被启动多次。每个线程实例只能执行一次其run()
方法,而.start()
方法用于启动线程,实际上是调用该线程的run()
方法。
如果你需要“重启”一个线程,即让它执行完一次任务后能够再次执行新的任务,有几种解决方案:
1. 使用循环
在run()
方法内部使用循环,并在循环中检查某个条件来退出循环或执行不同的任务。
import threading
class MyThread(threading.Thread):
def __init__(self, task_queue):
super().__init__()
self.task_queue = task_queue
self.running = True
def run(self):
while self.running:
if not self.task_queue.empty():
task = self.task_queue.get()
# 执行任务
print(f"Executing task: {task}")
# 假设任务执行完毕
self.task_queue.task_done()
def stop(self):
self.running = False
# 使用示例
from queue import Queue
task_queue = Queue()
thread = MyThread(task_queue)
thread.start()
# 向队列中添加任务
task_queue.put("Task 1")
task_queue.put("Task 2")
# 停止线程(如果需要)
# thread.stop()
# thread.join()
这里其实并没有真正结束线程,我们通过传入任务的方式进行多任务的操作,实际上线程一直存在,此时也没必要start,只需要不断往queue中put任务即可
2. 创建新的线程实例
如果任务之间有较大的不同,或者任务之间需要隔离的上下文,可以创建新的线程实例来“重启”线程。
import threading
def task(task_id):
print(f"Executing task {task_id}")
# 第一次启动线程
thread1 = threading.Thread(target=task, args=("1",))
thread1.start()
thread1.join()
# 创建并启动新的线程实例来执行新任务
thread2 = threading.Thread(target=task, args=("2",))
thread2.start()
thread2.join()
3. 使用线程池
如果你的应用场景涉及到大量短任务或者需要频繁地启动和停止线程,考虑使用concurrent.futures.ThreadPoolExecutor
。
from concurrent.futures import ThreadPoolExecutor
def task(task_id):
print(f"Executing task {task_id}")
with ThreadPoolExecutor(max_workers=5) as executor:
# 提交任务
executor.submit(task, "1")
executor.submit(task, "2")
# 可以继续提交更多任务
# 退出with块后,线程池将自动关闭
更多推荐
所有评论(0)