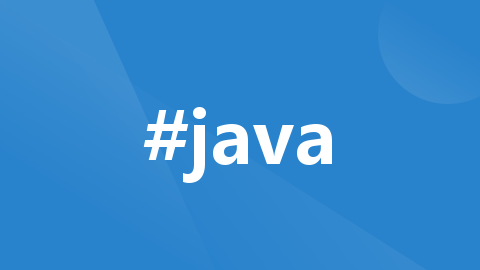
java 使用多线程处理业务逻辑和批量处理数据
将数据分批可以使用guava里面的工具类,pom中引入。
·
将数据分批可以使用guava里面的工具类,pom中引入
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>23.0</version>
</dependency>
package com.jettech.utils;
import com.google.common.collect.Lists;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
/**
* 线程池执行多业务逻辑
*/
public class ThreadExecutesBusiness {
public static void main(String[] args) {
executesMultiBusiness();
executesBusiness();
}
/**
* 线程池执行多业务逻辑
* 如:有四个业务要处理 每个业务使用一个线程执行
*/
public static void executesMultiBusiness(){
// 每个线程池执行一个业务逻辑
ExecutorService executorService = Executors.newFixedThreadPool(4);
CountDownLatch countDownLatch = new CountDownLatch(4);
try {
executorService.execute(()-> {
try {
Thread.sleep(1000L);
countDownLatch.countDown();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("业务一");
});
executorService.execute(()->{
try {
Thread.sleep(2000L);
countDownLatch.countDown();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("业务二");
});
executorService.execute(()->{
try {
Thread.sleep(3000L);
countDownLatch.countDown();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("业务三");
});
executorService.execute(()->{
try {
Thread.sleep(4000L);
countDownLatch.countDown();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("业务四");
});
//主线程阻塞,如果线程池之间也是需要前后结果的话,应该也是阻塞的 只有当countDown变为0的时候,主线程才不是阻塞的
try {
countDownLatch.await();
} catch (InterruptedException e) {
e.printStackTrace();
}
} finally {
executorService.shutdown();
}
}
/**
* 线程池执行批量任务
*/
public static void executesBusiness() {
List<Integer> list = new ArrayList<>();
for (int i = 0; i < 240; i++) {
list.add(i);
}
// 分批 每批100个
List<List<Integer>> batchList = Lists.partition(list,100);
// 创建线程池 线程数是 分批的数
ExecutorService executorService = Executors.newFixedThreadPool(batchList.size());
//线程计数器,就是 分批的数
CountDownLatch countDownLatch = new CountDownLatch(batchList.size());
batchList.forEach(e->{
//每个分批用一个线程执行
executorService.execute(()->{
e.forEach(num->{
// 取到每个数据进行业务执行
System.out.println(num);
});
});
countDownLatch.countDown();
});
try {
//主线程阻塞,如果线程池之间也是需要前后结果的话,应该也是阻塞的 只有当countDown变为0的时候,主线程才不是阻塞的
countDownLatch.await();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
executorService.shutdown();
}
}
}
更多推荐
所有评论(0)