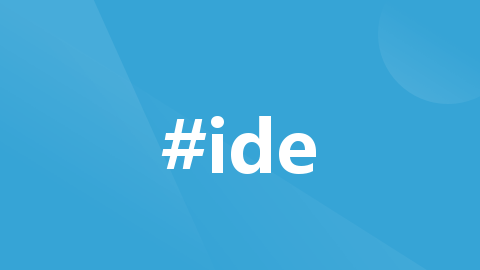
java 使用geotools依赖库构建地理处理工具类
【代码】java 使用geotools依赖库构建地理处理工具类。
·
文章目录
所需依赖 pom.xml
<repositories>
<repository>
<id>osgeo</id>
<name>OSGeo Release Repository</name>
<url>https://repo.osgeo.org/repository/release/</url>
<snapshots><enabled>false</enabled></snapshots>
<releases><enabled>true</enabled></releases>
</repository>
<repository>
<id>osgeo-snapshot</id>
<name>OSGeo Snapshot Repository</name>
<url>https://repo.osgeo.org/repository/snapshot/</url>
<snapshots><enabled>true</enabled></snapshots>
<releases><enabled>false</enabled></releases>
</repository>
</repositories>
<properties>
<java.version>1.8</java.version>
<geotools.version>21.0</geotools.version>
</properties>
<!-- JTS Topology Suite -->
<dependency>
<groupId>org.locationtech.jts</groupId>
<artifactId>jts-core</artifactId>
<version>1.16.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.locationtech.jts.io/jts-io-common -->
<dependency>
<groupId>org.locationtech.jts.io</groupId>
<artifactId>jts-io-common</artifactId>
<version>1.16.1</version>
</dependency>
<!-- JSON library -->
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.9</version>
</dependency>
<!-- GeoTools -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
<!-- GeoTools dependencies -->
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-geojsondatastore</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-main</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-geojson</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-process-feature</artifactId>
<version>${geotools.version}</version>
</dependency>
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-geojsondatastore</artifactId>
<version>${geotools.version}</version>
</dependency>
<!-- JTS拓扑套件是一个用于 建模和操作二维线性几何体的API。
它提供了许多几何谓词和函数。JTS符合opengis联盟发布的SQL的简单特性规范。
-->
<dependency>
<groupId>com.vividsolutions</groupId>
<artifactId>jts</artifactId>
<version>1.13</version>
</dependency>
执行相交操作,获取相交的要素集合
private static SimpleFeatureCollection performIntersect(SimpleFeatureCollection sourceFeatures, SimpleFeatureCollection targetFeatures) {
// 创建空的 SimpleFeatureCollection 来保存相交的要素
List<SimpleFeature> intersectedFeatureList = new ArrayList<>();
SimpleFeatureType featureType = createFeatureType(sourceFeatures.features().next());
try (SimpleFeatureIterator sourceIterator = sourceFeatures.features()) {
while (sourceIterator.hasNext()) {
SimpleFeature sourceFeature = sourceIterator.next();
Geometry sourceGeometry = (Geometry) sourceFeature.getDefaultGeometry();
try (SimpleFeatureIterator targetIterator = targetFeatures.features()) {
while (targetIterator.hasNext()) {
SimpleFeature targetFeature = targetIterator.next();
Geometry targetGeometry = (Geometry) targetFeature.getDefaultGeometry();
// 执行相交操作
if (sourceGeometry.intersects(targetGeometry)) {
// 获取两个要素的相交部分
Geometry intersection = sourceGeometry.intersection(targetGeometry);
// 创建 GeometryFactory
GeometryFactory geometryFactory = JTSFactoryFinder.getGeometryFactory();
// 创建 SimpleFeatureBuilder
SimpleFeatureBuilder featureBuilder = new SimpleFeatureBuilder(featureType);
// 添加面积字段 // 将面积从平方米转换为平方千米
double area = intersection.getArea()/ 1000000;
featureBuilder.set("orarea", sourceFeature.getAttribute("area"));
featureBuilder.set("area", area);
// 将相交部分的 SimpleFeature 添加到 featureBuilder 中
featureBuilder.set("geometry", intersection);
// featureBuilder.add(intersection);
SimpleFeature feature = featureBuilder.buildFeature(null);
// 添加相交的要素到结果列表
intersectedFeatureList.add(feature);
// intersectedFeatureList.add(sourceFeature);
break;
}
}
}
}
}
// 创建相交的要素集合
return featureListToCollection(intersectedFeatureList, featureType);
}
创建投影转换
private static MathTransform createTransform(CoordinateReferenceSystem sourceCRS, CoordinateReferenceSystem targetCRS) {
// 创建投影变换
MathTransform transform = null;
try {
transform = CRS.findMathTransform(sourceCRS, targetCRS, true);
} catch (Exception e) {
e.printStackTrace();
}
return transform;
}
对Geometry添加投影
private static Geometry projectGeometry(Geometry geometry, MathTransform transform) {
// 进行投影
try {
return JTS.transform(geometry, transform);
} catch (TransformException e) {
e.printStackTrace();
}
return null;
}
设置投影坐标系
private static CoordinateReferenceSystem getTargetCRS() {
// 获取目标投影坐标系(例如,UTM Zone 32N)
// 此处为
// 获取目标投影坐标系(例如,UTM Zone 32N)
CoordinateReferenceSystem targetCRS = null;
try {
targetCRS = CRS.decode("EPSG:32632"); // 使用 EPSG 编码指定投影坐标系
} catch (Exception e) {
e.printStackTrace();
}
return targetCRS;
}
创建feature属性
private static SimpleFeatureType createFeatureType(SimpleFeature sourceFeature) {
// 创建要素类型的 Schema
SimpleFeatureTypeBuilder typeBuilder = new SimpleFeatureTypeBuilder();
typeBuilder.setName("GeometryFeature");
typeBuilder.setCRS(sourceFeature.getFeatureType().getCoordinateReferenceSystem());
typeBuilder.add("geometry", Geometry.class);
typeBuilder.add("orarea", Double.class);
typeBuilder.add("area", Double.class);
return typeBuilder.buildFeatureType();
}
MultiPolygon转geojson
private static String convertToGeoJson(MultiPolygon geometry, SimpleFeatureType featureType) throws IOException {
FeatureJSON featureJSON = new FeatureJSON();
StringWriter writer = new StringWriter();
featureJSON.writeFeatureCollection((FeatureCollection) geometry, writer);
return writer.toString();
}
创建包含几何对象的 SimpleFeature
// 创建包含几何对象的 SimpleFeature
private static SimpleFeature createFeature(Geometry geometry, SimpleFeature sourceFeature, SimpleFeature targetFeature) {
SimpleFeatureType featureType = sourceFeature.getFeatureType();
SimpleFeatureBuilder featureBuilder = new SimpleFeatureBuilder(featureType);
// 将几何对象、源要素属性和目标要素属性添加到新的 SimpleFeature 中
featureBuilder.add(geometry);
featureBuilder.addAll(sourceFeature.getAttributes());
featureBuilder.addAll(targetFeature.getAttributes());
return featureBuilder.buildFeature(null);
}
添加SimpleFeatureCollection
private static SimpleFeatureCollection featureListToCollection(List<SimpleFeature> featureList,SimpleFeatureType featureType) {
// SimpleFeatureType featureType = prototype.getSchema();
return new ListFeatureCollection(featureType, featureList);
}
完整GeoJsonIntersectionTool工具类代码
import org.geotools.data.simple.SimpleFeatureCollection;
import org.geotools.data.simple.SimpleFeatureIterator;
import org.locationtech.jts.geom.Geometry;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class GeoJsonIntersectionTool {
public static void intersectGeoJSON(String geoJsonFile1, String geoJsonFile2,String savegeoJsonFile3) throws Exception {
// 读取源 .geojson 文件
File sourceFile = new File(geoJsonFile1);
// 读取目标 .geojson 文件
File targetFile = new File(geoJsonFile2);
// 创建 GeoTools 的 FeatureJSON 对象
FeatureJSON featureJSON = new FeatureJSON();
// 读取文件内容为字节数组
byte[] bytes = Files.readAllBytes(sourceFile.toPath());
// 将字节数组转换为字符串
String content = new String(bytes, StandardCharsets.UTF_8);
// 读取源文件中的要素集合
SimpleFeatureCollection sourceFeatures = (SimpleFeatureCollection) featureJSON.readFeatureCollection(readFileToString(sourceFile));
// 读取目标文件中的要素集合
SimpleFeatureCollection targetFeatures = (SimpleFeatureCollection) featureJSON.readFeatureCollection(readFileToString(targetFile));
// 执行相交操作,获取相交的要素集合
SimpleFeatureCollection intersectedFeatures = performIntersect(sourceFeatures, targetFeatures);
// 将相交的要素集合写入输出文件
File outputFile = new File(savegeoJsonFile3);
writeFeatureCollection(intersectedFeatures,outputFile);
System.out.println("相交的 .geojson 数据已写入输出文件:" + outputFile.getAbsolutePath());
}
public static void writeFeatureCollection(SimpleFeatureCollection featureCollection, File outputFile) throws IOException {
FileOutputStream fos = new FileOutputStream(outputFile);
FeatureJSON featureJSON = new FeatureJSON();
featureJSON.writeFeatureCollection(featureCollection, fos);
fos.flush();
fos.close();
}
public static String readFileToString(File file) throws IOException {
byte[] bytes = Files.readAllBytes(file.toPath());
return new String(bytes, StandardCharsets.UTF_8);
}
private static SimpleFeatureCollection performIntersect(SimpleFeatureCollection sourceFeatures, SimpleFeatureCollection targetFeatures) {
// 创建空的 SimpleFeatureCollection 来保存相交的要素
List<SimpleFeature> intersectedFeatureList = new ArrayList<>();
SimpleFeatureType featureType = createFeatureType(sourceFeatures.features().next());
try (SimpleFeatureIterator sourceIterator = sourceFeatures.features()) {
while (sourceIterator.hasNext()) {
SimpleFeature sourceFeature = sourceIterator.next();
Geometry sourceGeometry = (Geometry) sourceFeature.getDefaultGeometry();
try (SimpleFeatureIterator targetIterator = targetFeatures.features()) {
while (targetIterator.hasNext()) {
SimpleFeature targetFeature = targetIterator.next();
Geometry targetGeometry = (Geometry) targetFeature.getDefaultGeometry();
// 执行相交操作
if (sourceGeometry.intersects(targetGeometry)) {
// 获取两个要素的相交部分
Geometry intersection = sourceGeometry.intersection(targetGeometry);
// 创建 GeometryFactory
GeometryFactory geometryFactory = JTSFactoryFinder.getGeometryFactory();
// 创建 SimpleFeatureBuilder
SimpleFeatureBuilder featureBuilder = new SimpleFeatureBuilder(featureType);
// 添加面积字段 // 将面积从平方米转换为平方千米
double area = intersection.getArea()/ 1000000;
featureBuilder.set("orarea", sourceFeature.getAttribute("area"));
featureBuilder.set("area", area);
// 将相交部分的 SimpleFeature 添加到 featureBuilder 中
featureBuilder.set("geometry", intersection);
// featureBuilder.add(intersection);
SimpleFeature feature = featureBuilder.buildFeature(null);
// 添加相交的要素到结果列表
intersectedFeatureList.add(feature);
// intersectedFeatureList.add(sourceFeature);
break;
}
}
}
}
}
// 创建相交的要素集合
return featureListToCollection(intersectedFeatureList, featureType);
}
private static SimpleFeatureType createFeatureType(SimpleFeature sourceFeature) {
// 创建要素类型的 Schema
SimpleFeatureTypeBuilder typeBuilder = new SimpleFeatureTypeBuilder();
typeBuilder.setName("GeometryFeature");
typeBuilder.setCRS(sourceFeature.getFeatureType().getCoordinateReferenceSystem());
typeBuilder.add("geometry", Geometry.class);
typeBuilder.add("orarea", Double.class);
typeBuilder.add("area", Double.class);
return typeBuilder.buildFeatureType();
}
private static SimpleFeatureCollection featureListToCollection(List<SimpleFeature> featureList,SimpleFeatureType featureType) {
// SimpleFeatureType featureType = prototype.getSchema();
return new ListFeatureCollection(featureType, featureList);
}
}
更多推荐
所有评论(0)