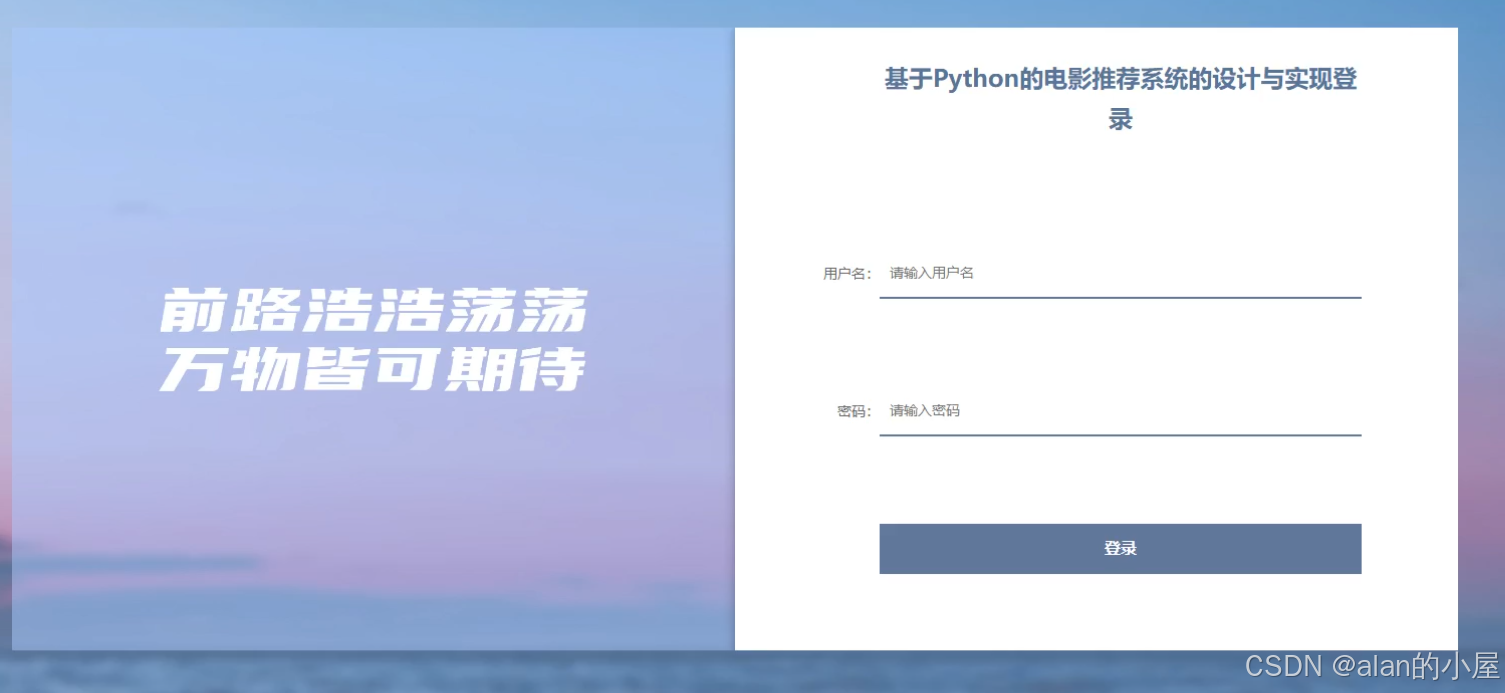
基于django的电影推荐系统(源码+LW+讲解和调试)
👑本项目是基于Django框架✅✅的电影推荐✅✅系统旨在为用户提供个化的电影推荐体验。用户可以注册并登录后,浏览丰富的电影数据库,查看影片的详细信息,包括评分、简介评论。系统采用推荐算法,根据用户的观看历史和评分,生成个性化的电影推荐列表。平台支持用户评分和评论,促进社区互动。管理员可以通过后台管理功能维护电影数据,分析用户反馈,以优化推荐效果。系统还提供搜索和筛选功能,方便用户根据类型、年份等
📝目录
👑项目简介
👑本项目是基于Django框架✅✅的电影推荐✅✅系统旨在为用户提供个化的电影推荐体验。用户可以注册并登录后,浏览丰富的电影数据库,查看影片的详细信息,包括评分、简介评论。系统采用推荐算法,根据用户的观看历史和评分,生成个性化的电影推荐列表。平台支持用户评分和评论,促进社区互动。管理员可以通过后台管理功能维护电影数据,分析用户反馈,以优化推荐效果。系统还提供搜索和筛选功能,方便用户根据类型、年份等条件查找影片。整体而言,该项目旨在提升用户的观影体验,帮助他们发现更多感兴趣的电影。
📷效果展示
📚技术栈
Python
Python是一种广泛使用的高级编程语言,以其简洁的语法和强大的功能而闻名。它支持多种编程范式,包括面向对象、函数式和命令式编程,使得开发者可以根据需要选择最合适的方法。Python拥有丰富的标准库和活跃的社区,适用于数据分析、机器学习、Web开发等多个领域。
Django
Django是一个高级的Web框架,基于Python开发,旨在帮助开发者快速构建安全、可维护的Web应用。它遵循“电池全包含”的原则,提供了许多内置功能,如用户认证、数据库管理和表单处理,从而减少了开发过程中常见的重复工作。Django强调快速开发和优雅设计,是构建复杂Web应用的理想选择。
MySQL
MySQL是一种流行的开源关系数据库管理系统,以高性能和可靠性著称。它使用结构化查询语言(SQL)进行数据管理,广泛应用于各种应用程序,包括Web应用和企业级系统。MySQL支持事务处理、外键和多用户环境,能够处理大量数据和高并发请求,适合各种规模的项目。
⌨️部分代码参考
package com.controller;
/**
* 用户
* 后端接口
*/
@RestController
@RequestMapping("/yonghu")
public class YonghuController {
@Autowired
private YonghuService yonghuService;
@Autowired
private TokenService tokenService;
/**
* 登录
*/
@IgnoreAuth
@RequestMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
YonghuEntity u = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("yonghuming", username));
if(u==null || !u.getMima().equals(password)) {
return R.error("账号或密码不正确");
}
String token = tokenService.generateToken(u.getId(), username,"yonghu", "用户" );
return R.ok().put("token", token);
}
/**
* 注册
*/
@IgnoreAuth
@RequestMapping("/register")
public R register(@RequestBody YonghuEntity yonghu){
//ValidatorUtils.validateEntity(yonghu);
YonghuEntity u = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("yonghuming", yonghu.getYonghuming()));
if(u!=null) {
return R.error("注册用户已存在");
}
Long uId = new Date().getTime();
yonghu.setId(uId);
yonghuService.insert(yonghu);
return R.ok();
}
/**
* 退出
*/
@RequestMapping("/logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
YonghuEntity u = yonghuService.selectById(id);
return R.ok().put("data", u);
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
YonghuEntity u = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("yonghuming", username));
if(u==null) {
return R.error("账号不存在");
}
u.setMima("123456");
yonghuService.updateById(u);
return R.ok("密码已重置为:123456");
}
/**
* 后台列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,YonghuEntity yonghu,
HttpServletRequest request){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
PageUtils page = yonghuService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yonghu), params), params));
return R.ok().put("data", page);
}
/**
* 前台列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,YonghuEntity yonghu,
HttpServletRequest request){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
PageUtils page = yonghuService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yonghu), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( YonghuEntity yonghu){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
ew.allEq(MPUtil.allEQMapPre( yonghu, "yonghu"));
return R.ok().put("data", yonghuService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(YonghuEntity yonghu){
EntityWrapper< YonghuEntity> ew = new EntityWrapper< YonghuEntity>();
ew.allEq(MPUtil.allEQMapPre( yonghu, "yonghu"));
YonghuView yonghuView = yonghuService.selectView(ew);
return R.ok("查询用户成功").put("data", yonghuView);
}
/**
* 后台详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
YonghuEntity yonghu = yonghuService.selectById(id);
return R.ok().put("data", yonghu);
}
/**
* 前台详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
YonghuEntity yonghu = yonghuService.selectById(id);
return R.ok().put("data", yonghu);
}
/**
* 后台保存
*/
@RequestMapping("/save")
@SysLog("新增用户")
public R save(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
if(yonghuService.selectCount(new EntityWrapper<YonghuEntity>().eq("yonghuming", yonghu.getYonghuming()))>0) {
return R.error("用户名已存在");
}
yonghu.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yonghu);
YonghuEntity u = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("yonghuming", yonghu.getYonghuming()));
if(u!=null) {
return R.error("用户已存在");
}
yonghu.setId(new Date().getTime());
yonghuService.insert(yonghu);
return R.ok();
}
/**
* 前台保存
*/
@SysLog("新增用户")
@RequestMapping("/add")
public R add(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
if(yonghuService.selectCount(new EntityWrapper<YonghuEntity>().eq("yonghuming", yonghu.getYonghuming()))>0) {
return R.error("用户名已存在");
}
yonghu.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yonghu);
YonghuEntity u = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("yonghuming", yonghu.getYonghuming()));
if(u!=null) {
return R.error("用户已存在");
}
yonghu.setId(new Date().getTime());
yonghuService.insert(yonghu);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
@Transactional
@SysLog("修改用户")
public R update(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
//ValidatorUtils.validateEntity(yonghu);
if(yonghuService.selectCount(new EntityWrapper<YonghuEntity>().ne("id", yonghu.getId()).eq("yonghuming", yonghu.getYonghuming()))>0) {
return R.error("用户名已存在");
}
yonghuService.updateById(yonghu);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
@SysLog("删除用户")
public R delete(@RequestBody Long[] ids){
yonghuService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
}
package com.aspect;
/**
* 系统日志,切面处理类
*/
@Aspect
@Component
public class SysLogAspect {
@Autowired
private SyslogService syslogService;
@Pointcut("@annotation(com.annotation.SysLog)")
public void logPointCut() {
}
@Around("logPointCut()")
public Object around(ProceedingJoinPoint point) throws Throwable {
long beginTime = System.currentTimeMillis();
//执行方法
Object result = point.proceed();
//执行时长(毫秒)
long time = System.currentTimeMillis() - beginTime;
//保存日志
saveSysLog(point, time);
return result;
}
private void saveSysLog(ProceedingJoinPoint joinPoint, long time) {
MethodSignature signature = (MethodSignature) joinPoint.getSignature();
Method method = signature.getMethod();
SyslogEntity sysLog = new SyslogEntity();
SysLog syslog = method.getAnnotation(SysLog.class);
if(syslog != null){
//注解上的描述
sysLog.setOperation(syslog.value());
}
//请求的方法名
String className = joinPoint.getTarget().getClass().getName();
String methodName = signature.getName();
sysLog.setMethod(className + "." + methodName + "()");
//请求的参数
Object[] args = joinPoint.getArgs();
try{
String params = new Gson().toJson(args[0]);
sysLog.setParams(params);
}catch (Exception e){
}
//获取request
HttpServletRequest request = HttpContextUtils.getHttpServletRequest();
//设置IP地址
sysLog.setIp(IPUtils.getIpAddr(request));
//用户名
String username = (String)request.getSession().getAttribute("username");
sysLog.setUsername(username);
sysLog.setTime(time);
sysLog.setAddtime(new Date());
//保存系统日志
syslogService.insert(sysLog);
}
}
📜项目论文
🙈为什么选择我
- 项目可根据要求更改或定制,满足多样化需求🍰
- 直接对接项目开发者,无中间商赚差价💰️
- 博主自己参与项目开发,了解项目架构和细节,提供全面答疑👨💻
- 提供源码、数据库、搭建环境、bug调试、技术辅导一条龙服务🐉
- todesk、向日葵、腾讯会议、语音电话快捷交流,高效沟通📞
📩源码获取
欢迎大家点赞👍、收藏⭐️、关注❤ 、咨询📧 ,下方查看👇🏻获取联系方式👇🏻
更多推荐
所有评论(0)