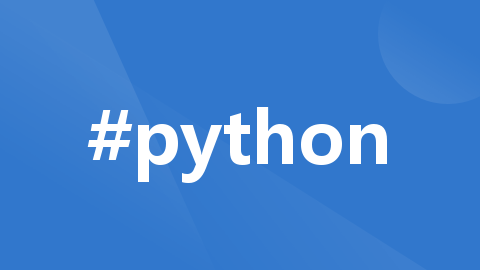
一个简单的Python智能合约开发
在Python中开发智能合约,我们可以使用Web3.py库,它是一个用于与以太坊区块链交互的Python库。以下是一个简单的Python智能合约开发教程,包括安装所需库、创建智能合约、部署智能合约和调用智能合约的功能。view关键字表明这个函数只是从合约读取数据,并不修改它,这使得它在只读上下文(如交易或对其他合约的调用)中是安全的。运行此脚本,它将调用智能合约的set函数设置值42,然后调用ge
智能合约是一种自动执行合同条款的计算机程序,它基于区块链技术。在Python中开发智能合约,我们可以使用Web3.py库,它是一个用于与以太坊区块链交互的Python库。以下是一个简单的Python智能合约开发教程,包括安装所需库、创建智能合约、部署智能合约和调用智能合约的功能。
1.安装所需库
首先,我们需要安装以下库:
pip install web3
创建智能合约
我们将使用Solidity编写一个简单的智能合约。创建一个名为SimpleStorage.sol的文件,并添加以下内容:
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 private storedData;
function set(uint256 x) public {
storedData = x;
}
function get() public view returns (uint256) {
return storedData;
}
}
这是一个名为SimpleStorage的简单Solidity合约。它包含两个函数:
set(uint256 x) public:这个函数接受一个无符号整数(uint256)作为输入,并将其设置为storedData变量的值。public关键字表示这个函数可以从合约外部调用。
get() public view returns (uint256):这个函数不接受任何参数,返回storedData变量的当前值。view关键字表明这个函数只是从合约读取数据,并不修改它,这使得它在只读上下文(如交易或对其他合约的调用)中是安全的。
合约还包括一个私有变量storedData,类型为uint256,用于存储由set函数设置并由get函数检索的值。
3.编译智能合约
要编译智能合约,我们需要使用Solidity编译器(如solc)。首先,确保你已经安装了solc。然后,在命令行中运行以下命令:
solc --abi --bin SimpleStorage.sol -o build
这将生成一个名为build的文件夹,其中包含编译后的智能合约的ABI(应用程序二进制接口)和字节码文件。
4.部署智能合约
现在我们需要使用Web3.py库部署智能合约。首先,创建一个名为deploy_contract.py的文件,并添加以下内容:
from web3 import Web3
from solc import compile_source
# 连接到本地以太坊节点
w3 = Web3(Web3.HTTPProvider('http://localhost:8545'))
# 确保连接成功
assert w3.isConnected()
# 读取编译后的智能合约
with open('build/SimpleStorage.abi', 'r') as file:
contract_abi = file.read()
with open('build/SimpleStorage.bin', 'r') as file:
contract_bytecode = file.read()
# 设置部署者的地址和私钥
deployer_address = '0xYourDeployerAddress'
deployer_private_key = '0xYourDeployerPrivateKey'
# 创建合约对象
simple_storage = w3.eth.contract(abi=contract_abi, bytecode=contract_bytecode)
# 估算部署合约所需的gas
gas_estimate = simple_storage.constructor().estimateGas()
# 构建部署交易
transaction = simple_storage.constructor().buildTransaction({
'from': deployer_address,
'gas': gas_estimate,
'nonce': w3.eth.getTransactionCount(deployer_address),
})
# 签署交易
signed_txn = w3.eth.account.signTransaction(transaction, deployer_private_key)
# 发送交易并获取交易哈希
tx_hash = w3.eth.sendRawTransaction(signed_txn.rawTransaction)
# 等待交易被矿工确认并获取合约地址
tx_receipt = w3.eth.waitForTransactionReceipt(tx_hash)
contract_address = tx_receipt['contractAddress']
print(f"Contract deployed at address: {contract_address}")
确保替换deployer_address和deployer_private_key为你的以太坊账户地址和私钥。运行此脚本,它将部署智能合约并输出合约地址。
5.调用智能合约
现在我们已经有了部署好的智能合约,我们可以调用它的set和get函数。创建一个名为interact_with_contract.py的文件,并添加以下内容:
from web3 import Web3
from solc import compile_source
# 连接到本地以太坊节点
w3 = Web3(Web3.HTTPProvider('http://localhost:8545'))
# 确保连接成功
assert w3.isConnected()
# 读取编译后的智能合约
with open('build/SimpleStorage.abi', 'r') as file:
contract_abi = file.read()
# 设置合约地址和部署者的地址
contract_address = '0xYourContractAddress'
deployer_address = '0xYourDeployerAddress'
# 创建合约对象
simple_storage = w3.eth.contract(address=contract_address, abi=contract_abi)
# 调用合约的set函数
set_txn = simple_storage.functions.set(42).buildTransaction({
'from': deployer_address,
'gas': 100000,
'nonce': w3.eth.getTransactionCount(deployer_address),
})
signed_set_txn = w3.eth.account.signTransaction(set_txn, '0xYourDeployerPrivateKey')
tx_hash = w3.eth.sendRawTransaction(signed_set_txn.rawTransaction)
w3.eth.waitForTransactionReceipt(tx_hash)
# 调用合约的get函数并打印结果
result = simple_storage.functions.get().call()
print(f"Stored value: {result}")
确保替换contract_address、deployer_address和deployer_private_key为你的合约地址、以太坊账户地址和私钥。运行此脚本,它将调用智能合约的set函数设置值42,然后调用get函数并打印存储的值。
更多推荐
所有评论(0)