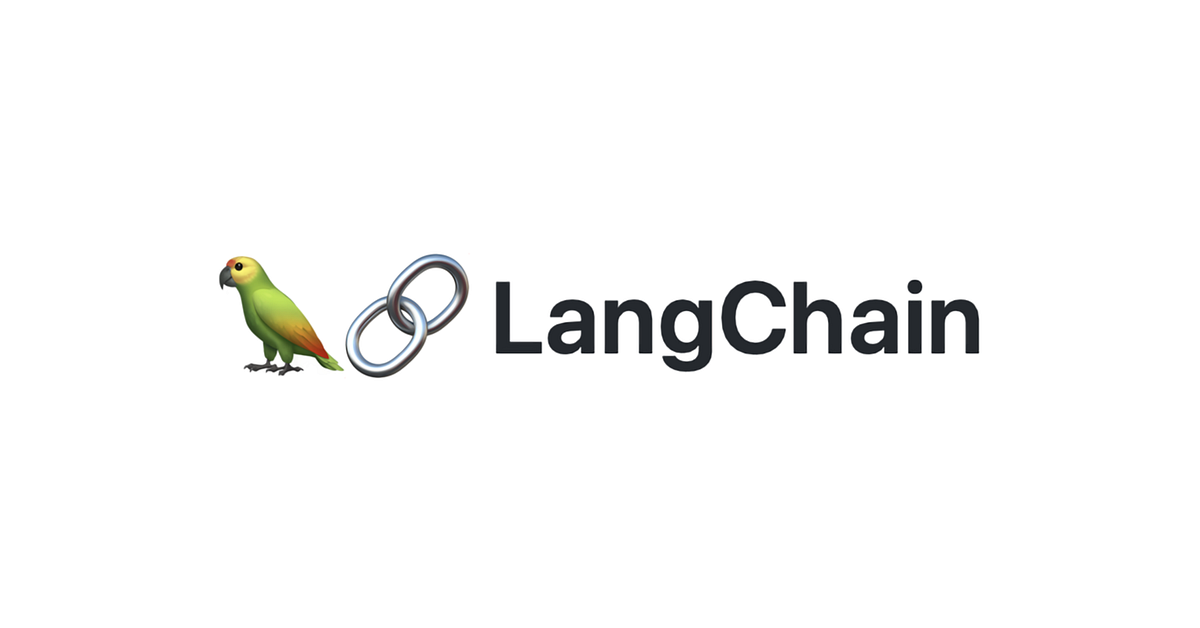
快速入门指南:使用 LangChain 构建智能聊天机器人
欢迎来到本快速入门指南!我将教您如何在一小时内使用 LangChain 构建一个智能聊天机器人。这个机器人能够进行对话并记住之前的互动记录。
·
快速入门指南:使用 LangChain 构建智能聊天机器人
一小时内掌握 LangChain 的核心技能!快速进入AI应用开发
欢迎来到本快速入门指南!我们将教您如何在一小时内使用 LangChain 构建一个智能聊天机器人。这个机器人能够进行对话并记住之前的互动记录。
通过本教程,您将学会:
- 使用聊天模型:了解如何选择和使用不同的语言模型。
- 使用 Prompt 模板:优化用户输入并生成适合模型处理的格式。
- 管理聊天记录:实现聊天机器人能够记住和处理对话历史。
- 使用 LangSmith 调试和跟踪:深入了解聊天机器人的内部工作机制。
- 流式响应:改善用户体验,实现逐字生成的流式响应。
让我们一起开始这个激动人心的旅程吧!
本指南基于最新版的LangChain 。
欢迎点赞收藏转发,您的鼓励是我坚持的动力。感谢
设置
Jupyter Notebook
为了更好地学习和理解 LLM 系统,我们推荐使用 Jupyter Notebook。请按照这里的安装指南进行安装。
安装
要安装 LangChain,请运行以下命令:
pip install langchain
更多详细信息,请参阅我们的安装指南。
快速入门
首先,让我们学习如何单独使用语言模型。LangChain 支持多种不同的语言模型,您可以根据需求选择使用。
import getpass
import os
from langchain_openai import ChatOpenAI
from langchain_core.messages import HumanMessage, AIMessage
os.environ["OPENAI_API_KEY"] = getpass.getpass()
model = ChatOpenAI(model="gpt-3.5-turbo")
response = model.invoke([HumanMessage(content="Hi! I'm Bob")])
print(response.content)
上面的代码中,模型直接回答了问题,但是没有记住对话内容。为了实现聊天机器人,我们需要保存并传递对话历史。
实现聊天机器人
我们可以使用 Message History
类来跟踪模型的输入和输出。首先,让我们安装 langchain-community
以便存储消息历史。
pip install langchain_community
然后,我们可以使用以下代码实现带有消息历史的聊天机器人:
from langchain_core.chat_history import InMemoryChatMessageHistory
from langchain_core.runnables.history import RunnableWithMessageHistory
store = {}
def get_session_history(session_id: str):
if session_id not in store:
store[session_id] = InMemoryChatMessageHistory()
return store[session_id]
with_message_history = RunnableWithMessageHistory(model, get_session_history)
config = {"configurable": {"session_id": "abc2"}}
response = with_message_history.invoke([HumanMessage(content="Hi! I'm Bob")], config=config)
print(response.content)
response = with_message_history.invoke([HumanMessage(content="What's my name?")], config=config)
print(response.content)
现在,聊天机器人能够记住对话内容并正确回答问题。
使用 Prompt 模板
为了使对话更加复杂和个性化,我们可以使用 Prompt Templates
。
from langchain_core.prompts import ChatPromptTemplate, MessagesPlaceholder
prompt = ChatPromptTemplate.from_messages(
[
("system", "You are a helpful assistant. Answer all questions to the best of your ability."),
MessagesPlaceholder(variable_name="messages"),
]
)
chain = prompt | model
with_message_history = RunnableWithMessageHistory(chain, get_session_history)
config = {"configurable": {"session_id": "abc5"}}
response = with_message_history.invoke([HumanMessage(content="Hi! I'm Jim")], config=config)
print(response.content)
管理对话历史
为了防止对话历史过长,我们需要在传递给模型之前对消息进行修剪。
from langchain_core.messages import SystemMessage, trim_messages
from operator import itemgetter
from langchain_core.runnables import RunnablePassthrough
trimmer = trim_messages(max_tokens=65, strategy="last", token_counter=model, include_system=True)
messages = [
SystemMessage(content="you're a good assistant"),
HumanMessage(content="hi! I'm bob"),
AIMessage(content="hi!"),
HumanMessage(content="I like vanilla ice cream"),
AIMessage(content="nice"),
HumanMessage(content="whats 2 + 2"),
AIMessage(content="4"),
HumanMessage(content="thanks"),
AIMessage(content="no problem!"),
HumanMessage(content="having fun?"),
AIMessage(content="yes!"),
]
chain = RunnablePassthrough.assign(messages=itemgetter("messages") | trimmer) | prompt | model
response = chain.invoke({"messages": messages + [HumanMessage(content="what's my name?")], "language": "English"})
print(response.content)
流式响应
为了改善用户体验,我们可以实现逐字生成的流式响应。
config = {"configurable": {"session_id": "abc15"}}
for r in with_message_history.stream(
{"messages": [HumanMessage(content="hi! I'm todd. tell me a joke")], "language": "English"},
config=config,
):
print(r.content, end="|")
通过以上步骤,您已经成功构建了一个智能聊天机器人!享受与您的新机器人助手互动的乐趣吧!
更多推荐
所有评论(0)